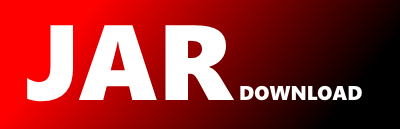
com.seq.api.BasePageIterable Maven / Gradle / Ivy
package com.seq.api;
import com.seq.exception.ChainException;
import com.seq.http.Client;
import java.lang.reflect.Type;
import java.util.Iterator;
public abstract class BasePageIterable implements Iterable {
private Client client;
private String path;
private Query nextQuery;
private final Type itemClass;
public BasePageIterable(Client client, String path, Query nextQuery, final Type itemClass) {
this.client = client;
this.path = path;
this.nextQuery = nextQuery;
this.itemClass = itemClass;
}
private T getPage() throws ChainException {
return this.client.request(this.path, this.nextQuery, this.itemClass);
}
public Iterator iterator() {
return new Iterator() {
public T currentPage;
/**
* Returns the next item in the results items.
* @return page object of type T
*/
public T next() {
return currentPage;
}
/**
* Returns true if there is another item in the results items.
* @return boolean
*/
public boolean hasNext() {
if (currentPage != null && currentPage.lastPage) {
return false;
}
try {
currentPage = getPage();
nextQuery = currentPage.next;
if (currentPage.items.size() == 0) {
return false;
}
} catch (ChainException e) {
return false;
}
return true;
}
/**
* This method is unsupported.
* @throws UnsupportedOperationException
*/
public void remove() throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy