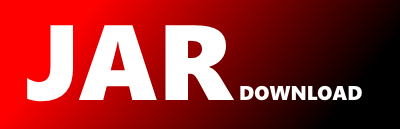
com.seq.api.BaseQueryBuilder Maven / Gradle / Ivy
package com.seq.api;
import com.seq.exception.ChainException;
import com.seq.http.Client;
import java.util.ArrayList;
import java.util.List;
/**
* Abstract base class providing an interface for building queries.
* @param the QueryBuilder class that extends BaseQueryBuilder
*/
public abstract class BaseQueryBuilder> {
protected Query next;
/**
* Execute the API query and return one page of items.
* @param client ledger API connection object
* @return a page of S objects that satisfy the query
* @throws ChainException
*/
public abstract S getPage(Client client) throws ChainException;
/**
* Executes the API query.
* @param client ledger API connection object
* @return an iterable over S objects that satisfy the query
* @throws ChainException
*/
public abstract S getIterable(Client client) throws ChainException;
public BaseQueryBuilder() {
this.next = new Query();
}
/**
* Sets the number of actions to include in each page.
* @param size the number of items on the page
* @return updated builder
*/
public T setPageSize(int size) {
this.next.pageSize = size;
return (T) this;
}
/**
* Sets the filter expression for the query.
* @param filter a filter expression
* @return updated builder
*/
public T setFilter(String filter) {
this.next.filter = filter;
return (T) this;
}
/**
* Adds a filter parameter that will be interpolated into the filter expression.
* @param param a filter parameter
* @return updated builder
*/
public T addFilterParameter(Object param) {
this.next.filterParams.add(param);
return (T) this;
}
/**
* Specifies the parameters that will be interpolated into the filter expression.
* @param params list of filter parameters
*/
public T setFilterParameters(List> params) {
this.next.filterParams = new ArrayList<>(params);
return (T) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy