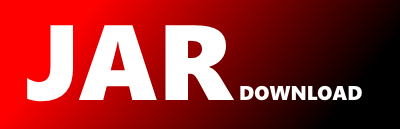
AddressValidation3USLib.AV3 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AV3US Show documentation
Show all versions of AV3US Show documentation
This package simplifies making API calls to Address Validation 3 US. It allows for testing on our trial environments and running against our production environments as well while implementing best practices and failover.
package AddressValidation3USLib;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.annotations.SerializedName;
/**
* AV3 Response Classes
*
* @author Service Objects
* @version 1.0
* @since 2023-02-14
*/
public class AV3 {
/**
* AV3 constructor
*/
public AV3() {}
/**
* GBM_Response
*/
public class GBM_Response {
/**
* GBM_Response constructor
*/
public GBM_Response() {}
/**The corrected address candidates.*/
@SerializedName("Addresses")
public Address[] Addresses;
/**Error object indicating why the service could not return a result.*/
@SerializedName("Error")
public Error Error;
/**Indicates if the unaltered input address is CASS certified. See “What is CASS?” below for more information.*/
@SerializedName("IsCASS")
public boolean IsCASS;
/**The corrected address candidate.*/
public class Address{
/**
* Address constructor
*/
public Address() {}
/**The corrected address line 1.*/
public String Address1;
/**The corrected address line 2.*/
public String Address2;
/**The corrected city name.*/
public String City;
/**The corrected state name.*/
public String State;
/**The corrected zip code + 4.*/
public String Zip;
/**Indicates if the address is for a residence.*/
public String IsResidential;
/**Number that correlates to a DPV(Delivery Point Validation) result. An indicator displaying whether or not the address is recognized as deliverable by the USPS.*/
public String DPV;
/**Explains DPV result.*/
public String DPVDesc;
/**Number that correlates to DPV notes description. Service Objects may add or change Note descriptions, but will never modify existing codes.*/
public String DPVNotes;
/**Details about the DPV result. Service Objects may add or change Note descriptions, but will never modify existing codes.*/
public String DPVNotesDesc;
/**Number that correlates to a Corrections Description. Service Objects may add or change Correction descriptions, but will never modify existing codes.*/
public String Corrections;
/**Description of what was corrected in an address. Service Objects may add or change Correction descriptions, but will never modify existing codes.*/
public String CorrectionsDesc;
/**The post office delivery barcode digits.*/
public String BarcodeDigits;
/**4 chars: 1 for the route type, 3 for the route code. Identifies a group of addresses when prepended by 5-digit Zip.*/
public String CarrierRoute;
/**The congress code is the congressional district number.*/
public String CongressCode;
/**The county code of the given address.*/
public String CountyCode;
/**The name of the county in which the given address lies.*/
public String CountyName;
/**The parsed house number of the given address.*/
public String FragmentHouse;
/**The parsed pre-directional of the address's street.*/
public String FragmentPreDir;
/**The parsed name of the street in the given address.*/
public String FragmentStreet;
/**The parsed suffix of the street in the given address.*/
public String FragmentSuffix;
/**The parsed post-directional of the address's street.*/
public String FragmentPostDir;
/**The parsed unit type (e.g. “Apt” or “Ste”)*/
public String FragmentUnit;
/**The parsed “Fragment” box, apartment or unit number.*/
public String Fragment;
/**The parsed type of the apartment, box, unit, etc.*/
public String FragmentPMBPrefix;
/**The parsed apartment, box, unit, etc. number of the given address.*/
public String FragmentPMBNumber;
}
/**The Error response.*/
public class Error{
/**
* Error constructor
*/
public Error() {}
/**The error type.*/
public String Type;
/**The error type code.*/
public String TypeCode;
/**The error description.*/
public String Desc;
/**The error description code.*/
public String DescCode;
}
/**Override of toString for end users to get quick output.*/
@Override
public String toString() {
Gson gson = new GsonBuilder().serializeNulls().create();
return gson.toJson(this);
}
}
/**
* GSN
*/
public class GSN_Response {
/**
* GSN_Response constructor
*/
public GSN_Response() {}
/**The corrected address line 1.*/
@SerializedName("Address1")
public String Address1;
/**The corrected city name.*/
@SerializedName("City")
public String City;
/**The corrected state name.*/
@SerializedName("State")
public String State;
/**The corrected zip code + 4.*/
@SerializedName("Zip")
public String Zip;
/**The total number of secondary numbers found for the input address.*/
@SerializedName("TotalCount")
public String TotalCount;
/**A list of possible secondary numbers for the input address.*/
@SerializedName("SecondaryNumbers")
public String[] SecondaryNumbers;
/**Error object indicating why the service could not return a result.*/
@SerializedName("Error")
public Error Error;
/**The Error response.*/
public class Error{
/**
* Error constructor
*/
public Error() {}
/**The error type.*/
public String Type;
/**The error type code.*/
public String TypeCode;
/**The error description.*/
public String Desc;
/**The error description code.*/
public String DescCode;
}
/**Override of toString for end users to get quick output.*/
@Override
public String toString() {
Gson gson = new GsonBuilder().serializeNulls().create();
return gson.toJson(this);
}
}
/**
* CSZ
*/
public class CSZ_Response {
/**
* CSZ constructor
*/
public CSZ_Response() {}
/**The CityStateZip response object.*/
@SerializedName("CityStateZip")
public CityStateZip CityStateZip;
/**The CityStateZip response*/
public class CityStateZip{
/**
* CityStateZip constructor
*/
public CityStateZip() {}
/**The corrected city name.*/
public String City;
/**The corrected state name.*/
public String State;
/**The corrected zip code. The standard zip code for the city and state is used if it was corrected.*/
public String Zip;
/**Indicates whether this location has General Delivery service.*/
public String GeneralDeliveryService;
/**Whether this location has PO Box service.*/
public String POBoxService;
/**Indicates whether this location has Street Delivery service.*/
public String StreetService;
/**Indicates whether this location has Rural Route / Highway Contract service.*/
public String RRHCService;
/**Indicates whether this location has Urbanization service.*/
public String UrbanizationService;
/**If PO Box service is available in the area, this is its lowest valid PO Box number.*/
public String POBoxRangeLow;
/** If PO Box service is available in the area, this is its highest valid PO Box number.*/
public String POBoxRangeHigh;
/**If the Zip code is assigned to a single entity like a University or Government building.*/
public String IsUniqueZipCode;
}
/**Error object indicating why the service could not return a result.*/
@SerializedName("Error")
public Error Error;
/**The Error response.*/
public class Error{
/**
* Error constructor
*/
public Error() {}
/**The error type.*/
public String Type;
/**The error type code.*/
public String TypeCode;
/**The error description.*/
public String Desc;
/**The error description code.*/
public String DescCode;
}
/**Override of toString for end users to get quick output.*/
@Override
public String toString() {
Gson gson = new GsonBuilder().serializeNulls().create();
return gson.toJson(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy