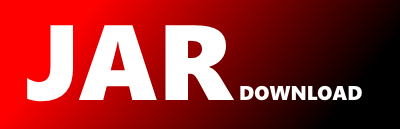
AddressValidation3USLib.AV3US Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of AV3US Show documentation
Show all versions of AV3US Show documentation
This package simplifies making API calls to Address Validation 3 US. It allows for testing on our trial environments and running against our production environments as well while implementing best practices and failover.
package AddressValidation3USLib;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.UnsupportedEncodingException;
import java.net.URL;
import java.net.URLEncoder;
import javax.net.ssl.HttpsURLConnection;
import com.google.gson.Gson;
/**
* Address Validation 3 - US
* Incomplete or inaccurate address information can impact your business in countless ways.
*
* Address Validation — US instantly verifies, corrects and appends addresses with near-perfect accuracy to meet the United States Postal Service’s mailing standards. Our CASS-certified engine validates addresses using multiple authoritative sources, including 160+ million USPS addresses and 15 million non-postal addresses, ensuring you have the most accurate addresses available.
*
* Our service ensures cost-saving delivery rates while reducing the expense associated with lost, undeliverable, and returned mail.
* @author Service Objects
* @version 1.0
* @since 2023-02-14
*/
public class AV3US {
/**
* AV3US constructor
*/
public AV3US() {}
/**
* Returns parsed and validated address elements including Delivery Point Validation (DPV), Residential Delivery Indicator (RDI), and Suite data. GetBestMatches will attempt to validate the input address against a CASS approved engine, and make corrections where possible. Multiple address candidates may be returned if a definitive decision cannot be made by the service.
* @param BusinessName Name of business associated with this address. Used to append Suite data.
* @param AddressOne Address line of the address to validate.
* @param AddressTwo This line is for address information that does not contribute to DPV coding an address. For example “C/O John Smith” does not help validate the address, but is still useful in delivery.
* @param City The city of the address to validate.
* @param State The state of the address to validate. For example, “NY”. This does not need to be contracted, full state names will work as well. The state isn’t required, but if one is not provided, the Zip code is required.
* @param Zip The zip code of the address to validate. A zip code isn’t required, but if one is not provided, the City and State are required.
* @param LicenseKey Your license key to use the service.
* @param IsLive Option to use live service or trial service
* @return AV3_GBM_Response This the container of the validation results.
*/
public AV3.GBM_Response GetBestMatches(String BusinessName, String AddressOne, String AddressTwo, String City, String State, String Zip, String LicenseKey, Boolean IsLive) throws Exception{
//Initialize variables
String businessName = "";
String addressOne = "";
String addressTwo = "";
String city = "";
String state = "";
String zip = "";
//Setup url endpoints to live, backup and trial;
String mainUrl = "https://ws.serviceobjects.com/AV3/api.svc/GetBestMatchesJson?";
String backupUrl = "https://wsbackup.serviceobjects.com/AV3/api.svc/GetBestMatchesJson?";
String trialUrl = "https://trial.serviceobjects.com/AV3/api.svc/GetBestMatchesJson?";
try {
//Pull in method arguements and encode the values for the call to the service
businessName = URLEncoder.encode(BusinessName, "UTF-8").replaceAll("\\+", "%20");
addressOne = URLEncoder.encode(AddressOne, "UTF-8").replaceAll("\\+", "%20");
addressTwo = URLEncoder.encode(AddressTwo, "UTF-8").replaceAll("\\+", "%20");
city = URLEncoder.encode(City, "UTF-8").replaceAll("\\+", "%20");
state = URLEncoder.encode(State, "UTF-8").replaceAll("\\+", "%20");
zip = URLEncoder.encode(Zip, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
}
//Build the querystring which will be the same regradless of endpoint
String QueryStringParameters = "BusinessName=" + businessName + "&Address=" + addressOne + "&Address2=" + addressTwo + "&City=" + city + "&State=" + state + "&PostalCode=" + zip + "&LicenseKey=" + LicenseKey;
String Message = "";
StringBuilder RawResponse = new StringBuilder();
AV3.GBM_Response Response = null;
if(IsLive == true)
{
RawResponse = HttpGet(mainUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null || (Response.Error != null && Response.Error.TypeCode == "3")) //Failover condition, typecode 3 is Service Objects Fatal
{
Message += "Response is null or API Error.TypeCode 3 Error, executing failover to wsbackup.serviceobjects.com;";
RawResponse = HttpGet(backupUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to wsbackup.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
}
else
{
RawResponse = HttpGet(trialUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to trial.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
return Response;
}
/**
* Returns parsed and validated address elements along with a list of potential secondary numbers for a given input address. The operation can be leveraged in conjunction with the GetBestMatches operation to find secondary numbers for input data that has either missing or incorrect unit information. For an example workflow please see the Workflow section below.
* @param Address Address line of the address to validate.
* @param City The city of the address to validate.
* @param State The state of the address to validate. For example, “NY”. This does not need to be contracted, full state names will work as well. The state isn’t required, but if one is not provided, the Zip code is required.
* @param Zip The zip code of the address to validate. A zip code isn’t required, but if one is not provided, the City and State are required.
* @param LicenseKey Your license key to use the service.
* @param IsLive Option to use live service or trial service
* @return BestMatchesResponse This the container of the validation results.
*/
public AV3.GSN_Response GetSecondaryNumbers(String Address, String City, String State, String Zip, String LicenseKey, Boolean IsLive) throws Exception{
//Initialize variables
String address = "";
String city = "";
String state = "";
String zip = "";
//Setup url endpoints to live, backup and trial;
String mainUrl = "https://ws.serviceobjects.com/AV3/api.svc/GetSecondaryNumbersJson?";
String backupUrl = "https://wsbackup.serviceobjects.com/AV3/api.svc/GetSecondaryNumbersJson?";
String trialUrl = "https://trial.serviceobjects.com/AV3/api.svc/GetSecondaryNumbersJson?";
try {
//Pull in method arguements and encode the values for the call to the service
address = URLEncoder.encode(Address, "UTF-8").replaceAll("\\+", "%20");
city = URLEncoder.encode(City, "UTF-8").replaceAll("\\+", "%20");
state = URLEncoder.encode(State, "UTF-8").replaceAll("\\+", "%20");
zip = URLEncoder.encode(Zip, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
}
//Build the querystring which will be the same regradless of endpoint
String QueryStringParameters = "Address=" + address + "&City=" + city + "&State=" + state + "&PostalCode=" + zip + "&LicenseKey=" + LicenseKey;
String Message = "";
StringBuilder RawResponse = new StringBuilder();
AV3.GSN_Response Response = null;
if(IsLive == true)
{
RawResponse = HttpGet(mainUrl + QueryStringParameters);
Response = (AV3.GSN_Response)(ProcessResponse(RawResponse, "GSN_Response"));
if (Response == null || (Response.Error != null && Response.Error.TypeCode == "3")) //Failover condition, typecode 3 is Service Objects Fatal
{
Message += "Response is null or API Error.TypeCode 3 Error, executing failover to wsbackup.serviceobjects.com;";
RawResponse = HttpGet(backupUrl + QueryStringParameters);
Response = (AV3.GSN_Response)(ProcessResponse(RawResponse, "GSN_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to wsbackup.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
}
else
{
RawResponse = HttpGet(trialUrl + QueryStringParameters);
Response = (AV3.GSN_Response)(ProcessResponse(RawResponse, "GSN_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to trial.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
return Response;
}
/**
* Takes a single line of address information as the input and returns the best candidate with parsed and corrected address information. This operation may return multiple address candidates if a single best match cannot be determined.
* @param BusinessName Name of business associated with this address. Used to append Suite data.
* @param Address Address line of the address to validate.
* @param LicenseKey Your license key to use the service.
* @param IsLive Option to use live service or trial service
* @return BestMatchesResponse This the container of the validation results.
*/
public AV3.GBM_Response GetBestMatchesSingleLine(String BusinessName, String Address, String LicenseKey, Boolean IsLive) throws Exception{
//Initialize variables
String address = "";
String businessName = "";
//Setup url endpoints to live, backup and trial;
String mainUrl = "https://ws.serviceobjects.com/AV3/api.svc/GetBestMatchesSingleLineJson?";
String backupUrl = "https://wsbackup.serviceobjects.com/AV3/api.svc/GetBestMatchesSingleLineJson?";
String trialUrl = "https://trial.serviceobjects.com/AV3/api.svc/GetBestMatchesSingleLineJson?";
try {
//Pull in method arguements and encode the values for the call to the service
businessName = URLEncoder.encode(BusinessName, "UTF-8").replaceAll("\\+", "%20");
address = URLEncoder.encode(Address, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
}
//Build the querystring which will be the same regradless of endpoint
String QueryStringParameters = "BusinessName=" + businessName + "&Address=" + address + "&LicenseKey=" + LicenseKey;
String Message = "";
StringBuilder RawResponse = new StringBuilder();
AV3.GBM_Response Response = null;
if(IsLive == true)
{
RawResponse = HttpGet(mainUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null || (Response.Error != null && Response.Error.TypeCode == "3")) //Failover condition, typecode 3 is Service Objects Fatal
{
Message += "Response is null or API Error.TypeCode 3 Error, executing failover to wsbackup.serviceobjects.com;";
RawResponse = HttpGet(backupUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to wsbackup.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
}
else
{
RawResponse = HttpGet(trialUrl + QueryStringParameters);
Response = (AV3.GBM_Response)(ProcessResponse(RawResponse, "GBM_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to trial.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
return Response;
}
/**
* This operation will validate that a given city-state-zip validate together properly. The inputs can be marginally incorrect, and this operation will correct them. For instance, a combination with a valid city, slightly misspelled state, and totally incorrect zip code will be corrected to a valid city – state – zip code combination.
* @param City The city of the address to validate.
* @param State The state of the address to validate. For example, “NY”. This does not need to be contracted, full state names will work as well. The state isn’t required, but if one is not provided, the Zip code is required.
* @param Zip The zip code of the address to validate. A zip code isn’t required, but if one is not provided, the City and State are required.
* @param LicenseKey Your license key to use the service.
* @param IsLive Option to use live service or trial service
* @return BestMatchesResponse This the container of the validation results.
*/
public AV3.CSZ_Response ValidateCityStateZip(String City, String State, String Zip, String LicenseKey, Boolean IsLive) throws Exception{
//Initialize variables
String city = "";
String state = "";
String zip = "";
//Setup url endpoints to live, backup and trial;
String mainUrl = "https://ws.serviceobjects.com/AV3/api.svc/CityStateZipInfo/";
String backupUrl = "https://wsbackup.serviceobjects.com/AV3/api.svc/CityStateZipInfo/";
String trialUrl = "https://trial.serviceobjects.com/AV3/api.svc/CityStateZipInfo/";
try {
//Pull in method arguements and encode the values for the call to the service
city = URLEncoder.encode(City, "UTF-8").replaceAll("\\+", "%20");
state = URLEncoder.encode(State, "UTF-8").replaceAll("\\+", "%20");
zip = URLEncoder.encode(Zip, "UTF-8").replaceAll("\\+", "%20");
} catch (UnsupportedEncodingException e) {
}
//Build the querystring which will be the same regradless of endpoint
String QueryStringParameters = city + "/" + state + "/" + zip + "/" + LicenseKey + "?format=json";
String Message = "";
StringBuilder RawResponse = new StringBuilder();
AV3.CSZ_Response Response = null;
if(IsLive == true)
{
RawResponse = HttpGet(mainUrl + QueryStringParameters);
Response = (AV3.CSZ_Response)(ProcessResponse(RawResponse, "CSZ_Response"));
if (Response == null || (Response.Error != null && Response.Error.TypeCode == "3")) //Failover condition, typecode 3 is Service Objects Fatal
{
Message += "Response is null or API Error.TypeCode 3 Error, executing failover to wsbackup.serviceobjects.com;";
RawResponse = HttpGet(backupUrl + QueryStringParameters);
Response = (AV3.CSZ_Response)(ProcessResponse(RawResponse, "CSZ_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to wsbackup.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
}
else
{
RawResponse = HttpGet(trialUrl + QueryStringParameters);
Response = (AV3.CSZ_Response)(ProcessResponse(RawResponse, "CSZ_Response"));
if (Response == null) //No Response returned from service, throw new exception.
{
Message += "Response is null on failover API call to trial.serviceobjects.com; Check network access to Service Objects endpoints.";
throw new Exception(Message);
}
else //There is a response so return it. There could be an API error but that will be in the Response.Error object and the end user will see it.
{
return Response;
}
}
return Response;
}
/**
* Returns a BestMatchesResponse object based on the input JSON string
* @param RawResponse Is a JSON string.
* @param ResponseType Is the string type of response object to cast to.
* @return Object Is the strongly typed response object for this service.
*/
private Object ProcessResponse(StringBuilder RawResponse, String ResponseType) throws Exception {
Gson gson = new Gson();
Object result = null;
switch(ResponseType) {
case "GBM_Response":
result = gson.fromJson(RawResponse.toString(), AV3.GBM_Response.class);
if(result == null)
{
throw new Exception("ERROR: trying to get GBM_Response");
}
return result;
case "GSN_Response":
result = gson.fromJson(RawResponse.toString(), AV3.GBM_Response.class);
if(result == null)
{
throw new Exception("ERROR: trying to get GSN_Response");
}
return result;
case "CSZ_Response":
result = gson.fromJson(RawResponse.toString(), AV3.GBM_Response.class);
if(result == null)
{
throw new Exception("ERROR: trying to get CSZ_Response");
}
return result;
default:
throw new Exception("ERROR: trying to get GBM_Response");
}
}
/**
* Makes the call to an endpoint and returns a string representation of the response from the service.
* @param endpoint Is the URL API endpoint of the service operation.
* @return StringBuilder Is the string representation of the response from the service.
*/
private StringBuilder HttpGet(String endpoint) {
int timeout = 5000;
URL url = null;
HttpsURLConnection conn = null;
StringBuilder sb = new StringBuilder();
try {
url = new URL(endpoint);
System.out.println(url);
conn = (HttpsURLConnection) url.openConnection();
conn.setConnectTimeout(timeout);
conn.setRequestMethod("GET");
conn.setRequestProperty("Accept", "application/json");
if (conn.getResponseCode() != 200) {
throw new RuntimeException("Failed : HTTP error code : " + conn.getResponseCode());
}
BufferedReader br = new BufferedReader(new InputStreamReader((conn.getInputStream())));
String output;
// System.out.println("info from the server \n");
while ((output = br.readLine()) != null) {
sb.append(output);
//System.out.println(output);
}
return sb;
} catch (Exception ex) {
System.out.println(ex.getMessage());
ex.printStackTrace();
}
finally {
if (conn != null)
conn.disconnect();
}
return sb;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy