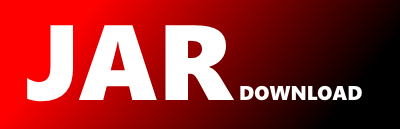
com.shapesecurity.shift.es2018.reducer.Director Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of es2018 Show documentation
Show all versions of es2018 Show documentation
Shift format ECMAScript 2018 AST tooling
// Generated by director.js
/**
* Copyright 2018 Shape Security, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.shapesecurity.shift.es2018.reducer;
import com.shapesecurity.functional.data.ImmutableList;
import com.shapesecurity.functional.data.Maybe;
import com.shapesecurity.shift.es2018.ast.*;
import javax.annotation.Nonnull;
public final class Director {
@Nonnull
public static State reduceArrayAssignmentTarget(
@Nonnull Reducer reducer,
@Nonnull ArrayAssignmentTarget node) {
return reducer.reduceArrayAssignmentTarget(node, reduceListMaybeAssignmentTargetAssignmentTargetWithDefault(reducer, node.elements), reduceMaybeAssignmentTarget(reducer, node.rest));
}
@Nonnull
public static State reduceArrayBinding(
@Nonnull Reducer reducer,
@Nonnull ArrayBinding node) {
return reducer.reduceArrayBinding(node, reduceListMaybeBindingBindingWithDefault(reducer, node.elements), reduceMaybeBinding(reducer, node.rest));
}
@Nonnull
public static State reduceArrayExpression(
@Nonnull Reducer reducer,
@Nonnull ArrayExpression node) {
return reducer.reduceArrayExpression(node, reduceListMaybeSpreadElementExpression(reducer, node.elements));
}
@Nonnull
public static State reduceArrowExpression(
@Nonnull Reducer reducer,
@Nonnull ArrowExpression node) {
return reducer.reduceArrowExpression(node, reduceFormalParameters(reducer, node.params), reduceFunctionBodyExpression(reducer, node.body));
}
@Nonnull
public static State reduceAssignmentExpression(
@Nonnull Reducer reducer,
@Nonnull AssignmentExpression node) {
return reducer.reduceAssignmentExpression(node, reduceAssignmentTarget(reducer, node.binding), reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceAssignmentTarget(
@Nonnull Reducer reducer,
@Nonnull AssignmentTarget node) {
if (node instanceof AssignmentTargetPattern) {
return reduceAssignmentTargetPattern(reducer, (AssignmentTargetPattern) node);
} else if (node instanceof SimpleAssignmentTarget) {
return reduceSimpleAssignmentTarget(reducer, (SimpleAssignmentTarget) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceAssignmentTargetAssignmentTargetWithDefault(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetAssignmentTargetWithDefault node) {
if (node instanceof AssignmentTarget) {
return reduceAssignmentTarget(reducer, (AssignmentTarget) node);
} else if (node instanceof AssignmentTargetWithDefault) {
return reduceAssignmentTargetWithDefault(reducer, (AssignmentTargetWithDefault) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceAssignmentTargetIdentifier(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetIdentifier node) {
return reducer.reduceAssignmentTargetIdentifier(node);
}
@Nonnull
public static State reduceAssignmentTargetPattern(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetPattern node) {
if (node instanceof ObjectAssignmentTarget) {
return reduceObjectAssignmentTarget(reducer, (ObjectAssignmentTarget) node);
} else if (node instanceof ArrayAssignmentTarget) {
return reduceArrayAssignmentTarget(reducer, (ArrayAssignmentTarget) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceAssignmentTargetProperty(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetProperty node) {
if (node instanceof AssignmentTargetPropertyIdentifier) {
return reduceAssignmentTargetPropertyIdentifier(reducer, (AssignmentTargetPropertyIdentifier) node);
} else if (node instanceof AssignmentTargetPropertyProperty) {
return reduceAssignmentTargetPropertyProperty(reducer, (AssignmentTargetPropertyProperty) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceAssignmentTargetPropertyIdentifier(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetPropertyIdentifier node) {
return reducer.reduceAssignmentTargetPropertyIdentifier(node, reduceAssignmentTargetIdentifier(reducer, node.binding), reduceMaybeExpression(reducer, node.init));
}
@Nonnull
public static State reduceAssignmentTargetPropertyProperty(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetPropertyProperty node) {
return reducer.reduceAssignmentTargetPropertyProperty(node, reducePropertyName(reducer, node.name), reduceAssignmentTargetAssignmentTargetWithDefault(reducer, node.binding));
}
@Nonnull
public static State reduceAssignmentTargetWithDefault(
@Nonnull Reducer reducer,
@Nonnull AssignmentTargetWithDefault node) {
return reducer.reduceAssignmentTargetWithDefault(node, reduceAssignmentTarget(reducer, node.binding), reduceExpression(reducer, node.init));
}
@Nonnull
public static State reduceAwaitExpression(
@Nonnull Reducer reducer,
@Nonnull AwaitExpression node) {
return reducer.reduceAwaitExpression(node, reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceBinaryExpression(
@Nonnull Reducer reducer,
@Nonnull BinaryExpression node) {
return reducer.reduceBinaryExpression(node, reduceExpression(reducer, node.left), reduceExpression(reducer, node.right));
}
@Nonnull
public static State reduceBinding(
@Nonnull Reducer reducer,
@Nonnull Binding node) {
if (node instanceof BindingPattern) {
return reduceBindingPattern(reducer, (BindingPattern) node);
} else if (node instanceof BindingIdentifier) {
return reduceBindingIdentifier(reducer, (BindingIdentifier) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceBindingBindingWithDefault(
@Nonnull Reducer reducer,
@Nonnull BindingBindingWithDefault node) {
if (node instanceof Binding) {
return reduceBinding(reducer, (Binding) node);
} else if (node instanceof BindingWithDefault) {
return reduceBindingWithDefault(reducer, (BindingWithDefault) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceBindingIdentifier(
@Nonnull Reducer reducer,
@Nonnull BindingIdentifier node) {
return reducer.reduceBindingIdentifier(node);
}
@Nonnull
public static State reduceBindingPattern(
@Nonnull Reducer reducer,
@Nonnull BindingPattern node) {
if (node instanceof ObjectBinding) {
return reduceObjectBinding(reducer, (ObjectBinding) node);
} else if (node instanceof ArrayBinding) {
return reduceArrayBinding(reducer, (ArrayBinding) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceBindingProperty(
@Nonnull Reducer reducer,
@Nonnull BindingProperty node) {
if (node instanceof BindingPropertyIdentifier) {
return reduceBindingPropertyIdentifier(reducer, (BindingPropertyIdentifier) node);
} else if (node instanceof BindingPropertyProperty) {
return reduceBindingPropertyProperty(reducer, (BindingPropertyProperty) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceBindingPropertyIdentifier(
@Nonnull Reducer reducer,
@Nonnull BindingPropertyIdentifier node) {
return reducer.reduceBindingPropertyIdentifier(node, reduceBindingIdentifier(reducer, node.binding), reduceMaybeExpression(reducer, node.init));
}
@Nonnull
public static State reduceBindingPropertyProperty(
@Nonnull Reducer reducer,
@Nonnull BindingPropertyProperty node) {
return reducer.reduceBindingPropertyProperty(node, reducePropertyName(reducer, node.name), reduceBindingBindingWithDefault(reducer, node.binding));
}
@Nonnull
public static State reduceBindingWithDefault(
@Nonnull Reducer reducer,
@Nonnull BindingWithDefault node) {
return reducer.reduceBindingWithDefault(node, reduceBinding(reducer, node.binding), reduceExpression(reducer, node.init));
}
@Nonnull
public static State reduceBlock(
@Nonnull Reducer reducer,
@Nonnull Block node) {
return reducer.reduceBlock(node, reduceListStatement(reducer, node.statements));
}
@Nonnull
public static State reduceBlockStatement(
@Nonnull Reducer reducer,
@Nonnull BlockStatement node) {
return reducer.reduceBlockStatement(node, reduceBlock(reducer, node.block));
}
@Nonnull
public static State reduceBreakStatement(
@Nonnull Reducer reducer,
@Nonnull BreakStatement node) {
return reducer.reduceBreakStatement(node);
}
@Nonnull
public static State reduceCallExpression(
@Nonnull Reducer reducer,
@Nonnull CallExpression node) {
return reducer.reduceCallExpression(node, reduceExpressionSuper(reducer, node.callee), reduceListSpreadElementExpression(reducer, node.arguments));
}
@Nonnull
public static State reduceCatchClause(
@Nonnull Reducer reducer,
@Nonnull CatchClause node) {
return reducer.reduceCatchClause(node, reduceBinding(reducer, node.binding), reduceBlock(reducer, node.body));
}
@Nonnull
public static State reduceClassDeclaration(
@Nonnull Reducer reducer,
@Nonnull ClassDeclaration node) {
return reducer.reduceClassDeclaration(node, reduceBindingIdentifier(reducer, node.name), reduceMaybeExpression(reducer, node._super), reduceListClassElement(reducer, node.elements));
}
@Nonnull
public static State reduceClassElement(
@Nonnull Reducer reducer,
@Nonnull ClassElement node) {
return reducer.reduceClassElement(node, reduceMethodDefinition(reducer, node.method));
}
@Nonnull
public static State reduceClassExpression(
@Nonnull Reducer reducer,
@Nonnull ClassExpression node) {
return reducer.reduceClassExpression(node, reduceMaybeBindingIdentifier(reducer, node.name), reduceMaybeExpression(reducer, node._super), reduceListClassElement(reducer, node.elements));
}
@Nonnull
public static State reduceCompoundAssignmentExpression(
@Nonnull Reducer reducer,
@Nonnull CompoundAssignmentExpression node) {
return reducer.reduceCompoundAssignmentExpression(node, reduceSimpleAssignmentTarget(reducer, node.binding), reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceComputedMemberAssignmentTarget(
@Nonnull Reducer reducer,
@Nonnull ComputedMemberAssignmentTarget node) {
return reducer.reduceComputedMemberAssignmentTarget(node, reduceExpressionSuper(reducer, node.object), reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceComputedMemberExpression(
@Nonnull Reducer reducer,
@Nonnull ComputedMemberExpression node) {
return reducer.reduceComputedMemberExpression(node, reduceExpressionSuper(reducer, node.object), reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceComputedPropertyName(
@Nonnull Reducer reducer,
@Nonnull ComputedPropertyName node) {
return reducer.reduceComputedPropertyName(node, reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceConditionalExpression(
@Nonnull Reducer reducer,
@Nonnull ConditionalExpression node) {
return reducer.reduceConditionalExpression(node, reduceExpression(reducer, node.test), reduceExpression(reducer, node.consequent), reduceExpression(reducer, node.alternate));
}
@Nonnull
public static State reduceContinueStatement(
@Nonnull Reducer reducer,
@Nonnull ContinueStatement node) {
return reducer.reduceContinueStatement(node);
}
@Nonnull
public static State reduceDataProperty(
@Nonnull Reducer reducer,
@Nonnull DataProperty node) {
return reducer.reduceDataProperty(node, reducePropertyName(reducer, node.name), reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceDebuggerStatement(
@Nonnull Reducer reducer,
@Nonnull DebuggerStatement node) {
return reducer.reduceDebuggerStatement(node);
}
@Nonnull
public static State reduceDirective(
@Nonnull Reducer reducer,
@Nonnull Directive node) {
return reducer.reduceDirective(node);
}
@Nonnull
public static State reduceDoWhileStatement(
@Nonnull Reducer reducer,
@Nonnull DoWhileStatement node) {
return reducer.reduceDoWhileStatement(node, reduceStatement(reducer, node.body), reduceExpression(reducer, node.test));
}
@Nonnull
public static State reduceEmptyStatement(
@Nonnull Reducer reducer,
@Nonnull EmptyStatement node) {
return reducer.reduceEmptyStatement(node);
}
@Nonnull
public static State reduceExport(
@Nonnull Reducer reducer,
@Nonnull Export node) {
return reducer.reduceExport(node, reduceFunctionDeclarationClassDeclarationVariableDeclaration(reducer, node.declaration));
}
@Nonnull
public static State reduceExportAllFrom(
@Nonnull Reducer reducer,
@Nonnull ExportAllFrom node) {
return reducer.reduceExportAllFrom(node);
}
@Nonnull
public static State reduceExportDeclaration(
@Nonnull Reducer reducer,
@Nonnull ExportDeclaration node) {
if (node instanceof ExportAllFrom) {
return reduceExportAllFrom(reducer, (ExportAllFrom) node);
} else if (node instanceof ExportFrom) {
return reduceExportFrom(reducer, (ExportFrom) node);
} else if (node instanceof ExportLocals) {
return reduceExportLocals(reducer, (ExportLocals) node);
} else if (node instanceof Export) {
return reduceExport(reducer, (Export) node);
} else if (node instanceof ExportDefault) {
return reduceExportDefault(reducer, (ExportDefault) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceExportDefault(
@Nonnull Reducer reducer,
@Nonnull ExportDefault node) {
return reducer.reduceExportDefault(node, reduceFunctionDeclarationClassDeclarationExpression(reducer, node.body));
}
@Nonnull
public static State reduceExportFrom(
@Nonnull Reducer reducer,
@Nonnull ExportFrom node) {
return reducer.reduceExportFrom(node, reduceListExportFromSpecifier(reducer, node.namedExports));
}
@Nonnull
public static State reduceExportFromSpecifier(
@Nonnull Reducer reducer,
@Nonnull ExportFromSpecifier node) {
return reducer.reduceExportFromSpecifier(node);
}
@Nonnull
public static State reduceExportLocalSpecifier(
@Nonnull Reducer reducer,
@Nonnull ExportLocalSpecifier node) {
return reducer.reduceExportLocalSpecifier(node, reduceIdentifierExpression(reducer, node.name));
}
@Nonnull
public static State reduceExportLocals(
@Nonnull Reducer reducer,
@Nonnull ExportLocals node) {
return reducer.reduceExportLocals(node, reduceListExportLocalSpecifier(reducer, node.namedExports));
}
@Nonnull
public static State reduceExpression(
@Nonnull Reducer reducer,
@Nonnull Expression node) {
if (node instanceof MemberExpression) {
return reduceMemberExpression(reducer, (MemberExpression) node);
} else if (node instanceof ClassExpression) {
return reduceClassExpression(reducer, (ClassExpression) node);
} else if (node instanceof LiteralBooleanExpression) {
return reduceLiteralBooleanExpression(reducer, (LiteralBooleanExpression) node);
} else if (node instanceof LiteralInfinityExpression) {
return reduceLiteralInfinityExpression(reducer, (LiteralInfinityExpression) node);
} else if (node instanceof LiteralNullExpression) {
return reduceLiteralNullExpression(reducer, (LiteralNullExpression) node);
} else if (node instanceof LiteralNumericExpression) {
return reduceLiteralNumericExpression(reducer, (LiteralNumericExpression) node);
} else if (node instanceof LiteralRegExpExpression) {
return reduceLiteralRegExpExpression(reducer, (LiteralRegExpExpression) node);
} else if (node instanceof LiteralStringExpression) {
return reduceLiteralStringExpression(reducer, (LiteralStringExpression) node);
} else if (node instanceof ArrayExpression) {
return reduceArrayExpression(reducer, (ArrayExpression) node);
} else if (node instanceof ArrowExpression) {
return reduceArrowExpression(reducer, (ArrowExpression) node);
} else if (node instanceof AssignmentExpression) {
return reduceAssignmentExpression(reducer, (AssignmentExpression) node);
} else if (node instanceof BinaryExpression) {
return reduceBinaryExpression(reducer, (BinaryExpression) node);
} else if (node instanceof CallExpression) {
return reduceCallExpression(reducer, (CallExpression) node);
} else if (node instanceof CompoundAssignmentExpression) {
return reduceCompoundAssignmentExpression(reducer, (CompoundAssignmentExpression) node);
} else if (node instanceof ConditionalExpression) {
return reduceConditionalExpression(reducer, (ConditionalExpression) node);
} else if (node instanceof FunctionExpression) {
return reduceFunctionExpression(reducer, (FunctionExpression) node);
} else if (node instanceof IdentifierExpression) {
return reduceIdentifierExpression(reducer, (IdentifierExpression) node);
} else if (node instanceof NewExpression) {
return reduceNewExpression(reducer, (NewExpression) node);
} else if (node instanceof NewTargetExpression) {
return reduceNewTargetExpression(reducer, (NewTargetExpression) node);
} else if (node instanceof ObjectExpression) {
return reduceObjectExpression(reducer, (ObjectExpression) node);
} else if (node instanceof UnaryExpression) {
return reduceUnaryExpression(reducer, (UnaryExpression) node);
} else if (node instanceof TemplateExpression) {
return reduceTemplateExpression(reducer, (TemplateExpression) node);
} else if (node instanceof ThisExpression) {
return reduceThisExpression(reducer, (ThisExpression) node);
} else if (node instanceof UpdateExpression) {
return reduceUpdateExpression(reducer, (UpdateExpression) node);
} else if (node instanceof YieldExpression) {
return reduceYieldExpression(reducer, (YieldExpression) node);
} else if (node instanceof YieldGeneratorExpression) {
return reduceYieldGeneratorExpression(reducer, (YieldGeneratorExpression) node);
} else if (node instanceof AwaitExpression) {
return reduceAwaitExpression(reducer, (AwaitExpression) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceExpressionStatement(
@Nonnull Reducer reducer,
@Nonnull ExpressionStatement node) {
return reducer.reduceExpressionStatement(node, reduceExpression(reducer, node.expression));
}
@Nonnull
public static State reduceExpressionSuper(
@Nonnull Reducer reducer,
@Nonnull ExpressionSuper node) {
if (node instanceof Expression) {
return reduceExpression(reducer, (Expression) node);
} else if (node instanceof Super) {
return reduceSuper(reducer, (Super) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceExpressionTemplateElement(
@Nonnull Reducer reducer,
@Nonnull ExpressionTemplateElement node) {
if (node instanceof Expression) {
return reduceExpression(reducer, (Expression) node);
} else if (node instanceof TemplateElement) {
return reduceTemplateElement(reducer, (TemplateElement) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceForAwaitStatement(
@Nonnull Reducer reducer,
@Nonnull ForAwaitStatement node) {
return reducer.reduceForAwaitStatement(node, reduceVariableDeclarationAssignmentTarget(reducer, node.left), reduceExpression(reducer, node.right), reduceStatement(reducer, node.body));
}
@Nonnull
public static State reduceForInStatement(
@Nonnull Reducer reducer,
@Nonnull ForInStatement node) {
return reducer.reduceForInStatement(node, reduceVariableDeclarationAssignmentTarget(reducer, node.left), reduceExpression(reducer, node.right), reduceStatement(reducer, node.body));
}
@Nonnull
public static State reduceForOfStatement(
@Nonnull Reducer reducer,
@Nonnull ForOfStatement node) {
return reducer.reduceForOfStatement(node, reduceVariableDeclarationAssignmentTarget(reducer, node.left), reduceExpression(reducer, node.right), reduceStatement(reducer, node.body));
}
@Nonnull
public static State reduceForStatement(
@Nonnull Reducer reducer,
@Nonnull ForStatement node) {
return reducer.reduceForStatement(node, reduceMaybeVariableDeclarationExpression(reducer, node.init), reduceMaybeExpression(reducer, node.test), reduceMaybeExpression(reducer, node.update), reduceStatement(reducer, node.body));
}
@Nonnull
public static State reduceFormalParameters(
@Nonnull Reducer reducer,
@Nonnull FormalParameters node) {
return reducer.reduceFormalParameters(node, reduceListParameter(reducer, node.items), reduceMaybeBinding(reducer, node.rest));
}
@Nonnull
public static State reduceFunctionBody(
@Nonnull Reducer reducer,
@Nonnull FunctionBody node) {
return reducer.reduceFunctionBody(node, reduceListDirective(reducer, node.directives), reduceListStatement(reducer, node.statements));
}
@Nonnull
public static State reduceFunctionBodyExpression(
@Nonnull Reducer reducer,
@Nonnull FunctionBodyExpression node) {
if (node instanceof FunctionBody) {
return reduceFunctionBody(reducer, (FunctionBody) node);
} else if (node instanceof Expression) {
return reduceExpression(reducer, (Expression) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceFunctionDeclaration(
@Nonnull Reducer reducer,
@Nonnull FunctionDeclaration node) {
return reducer.reduceFunctionDeclaration(node, reduceBindingIdentifier(reducer, node.name), reduceFormalParameters(reducer, node.params), reduceFunctionBody(reducer, node.body));
}
@Nonnull
public static State reduceFunctionDeclarationClassDeclarationExpression(
@Nonnull Reducer reducer,
@Nonnull FunctionDeclarationClassDeclarationExpression node) {
if (node instanceof FunctionDeclaration) {
return reduceFunctionDeclaration(reducer, (FunctionDeclaration) node);
} else if (node instanceof ClassDeclaration) {
return reduceClassDeclaration(reducer, (ClassDeclaration) node);
} else if (node instanceof Expression) {
return reduceExpression(reducer, (Expression) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceFunctionDeclarationClassDeclarationVariableDeclaration(
@Nonnull Reducer reducer,
@Nonnull FunctionDeclarationClassDeclarationVariableDeclaration node) {
if (node instanceof FunctionDeclaration) {
return reduceFunctionDeclaration(reducer, (FunctionDeclaration) node);
} else if (node instanceof ClassDeclaration) {
return reduceClassDeclaration(reducer, (ClassDeclaration) node);
} else if (node instanceof VariableDeclaration) {
return reduceVariableDeclaration(reducer, (VariableDeclaration) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceFunctionExpression(
@Nonnull Reducer reducer,
@Nonnull FunctionExpression node) {
return reducer.reduceFunctionExpression(node, reduceMaybeBindingIdentifier(reducer, node.name), reduceFormalParameters(reducer, node.params), reduceFunctionBody(reducer, node.body));
}
@Nonnull
public static State reduceGetter(
@Nonnull Reducer reducer,
@Nonnull Getter node) {
return reducer.reduceGetter(node, reducePropertyName(reducer, node.name), reduceFunctionBody(reducer, node.body));
}
@Nonnull
public static State reduceIdentifierExpression(
@Nonnull Reducer reducer,
@Nonnull IdentifierExpression node) {
return reducer.reduceIdentifierExpression(node);
}
@Nonnull
public static State reduceIfStatement(
@Nonnull Reducer reducer,
@Nonnull IfStatement node) {
return reducer.reduceIfStatement(node, reduceExpression(reducer, node.test), reduceStatement(reducer, node.consequent), reduceMaybeStatement(reducer, node.alternate));
}
@Nonnull
public static State reduceImport(
@Nonnull Reducer reducer,
@Nonnull Import node) {
return reducer.reduceImport(node, reduceMaybeBindingIdentifier(reducer, node.defaultBinding), reduceListImportSpecifier(reducer, node.namedImports));
}
@Nonnull
public static State reduceImportDeclaration(
@Nonnull Reducer reducer,
@Nonnull ImportDeclaration node) {
if (node instanceof Import) {
return reduceImport(reducer, (Import) node);
} else if (node instanceof ImportNamespace) {
return reduceImportNamespace(reducer, (ImportNamespace) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceImportDeclarationExportDeclarationStatement(
@Nonnull Reducer reducer,
@Nonnull ImportDeclarationExportDeclarationStatement node) {
if (node instanceof ImportDeclaration) {
return reduceImportDeclaration(reducer, (ImportDeclaration) node);
} else if (node instanceof ExportDeclaration) {
return reduceExportDeclaration(reducer, (ExportDeclaration) node);
} else if (node instanceof Statement) {
return reduceStatement(reducer, (Statement) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceImportNamespace(
@Nonnull Reducer reducer,
@Nonnull ImportNamespace node) {
return reducer.reduceImportNamespace(node, reduceMaybeBindingIdentifier(reducer, node.defaultBinding), reduceBindingIdentifier(reducer, node.namespaceBinding));
}
@Nonnull
public static State reduceImportSpecifier(
@Nonnull Reducer reducer,
@Nonnull ImportSpecifier node) {
return reducer.reduceImportSpecifier(node, reduceBindingIdentifier(reducer, node.binding));
}
@Nonnull
public static State reduceIterationStatement(
@Nonnull Reducer reducer,
@Nonnull IterationStatement node) {
if (node instanceof DoWhileStatement) {
return reduceDoWhileStatement(reducer, (DoWhileStatement) node);
} else if (node instanceof ForInStatement) {
return reduceForInStatement(reducer, (ForInStatement) node);
} else if (node instanceof ForOfStatement) {
return reduceForOfStatement(reducer, (ForOfStatement) node);
} else if (node instanceof ForAwaitStatement) {
return reduceForAwaitStatement(reducer, (ForAwaitStatement) node);
} else if (node instanceof ForStatement) {
return reduceForStatement(reducer, (ForStatement) node);
} else if (node instanceof WhileStatement) {
return reduceWhileStatement(reducer, (WhileStatement) node);
} else {
throw new RuntimeException("Not reached");
}
}
@Nonnull
public static State reduceLabeledStatement(
@Nonnull Reducer reducer,
@Nonnull LabeledStatement node) {
return reducer.reduceLabeledStatement(node, reduceStatement(reducer, node.body));
}
@Nonnull
public static ImmutableList reduceListAssignmentTargetProperty(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceAssignmentTargetProperty(reducer, x));
}
@Nonnull
public static ImmutableList reduceListBindingProperty(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceBindingProperty(reducer, x));
}
@Nonnull
public static ImmutableList reduceListClassElement(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceClassElement(reducer, x));
}
@Nonnull
public static ImmutableList reduceListDirective(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceDirective(reducer, x));
}
@Nonnull
public static ImmutableList reduceListExportFromSpecifier(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceExportFromSpecifier(reducer, x));
}
@Nonnull
public static ImmutableList reduceListExportLocalSpecifier(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceExportLocalSpecifier(reducer, x));
}
@Nonnull
public static ImmutableList reduceListExpressionTemplateElement(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceExpressionTemplateElement(reducer, x));
}
@Nonnull
public static ImmutableList reduceListImportDeclarationExportDeclarationStatement(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceImportDeclarationExportDeclarationStatement(reducer, x));
}
@Nonnull
public static ImmutableList reduceListImportSpecifier(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceImportSpecifier(reducer, x));
}
@Nonnull
public static ImmutableList> reduceListMaybeAssignmentTargetAssignmentTargetWithDefault(
@Nonnull Reducer reducer,
@Nonnull ImmutableList> list) {
return list.map(x -> reduceMaybeAssignmentTargetAssignmentTargetWithDefault(reducer, x));
}
@Nonnull
public static ImmutableList> reduceListMaybeBindingBindingWithDefault(
@Nonnull Reducer reducer,
@Nonnull ImmutableList> list) {
return list.map(x -> reduceMaybeBindingBindingWithDefault(reducer, x));
}
@Nonnull
public static ImmutableList> reduceListMaybeSpreadElementExpression(
@Nonnull Reducer reducer,
@Nonnull ImmutableList> list) {
return list.map(x -> reduceMaybeSpreadElementExpression(reducer, x));
}
@Nonnull
public static ImmutableList reduceListObjectProperty(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceObjectProperty(reducer, x));
}
@Nonnull
public static ImmutableList reduceListParameter(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceParameter(reducer, x));
}
@Nonnull
public static ImmutableList reduceListSpreadElementExpression(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceSpreadElementExpression(reducer, x));
}
@Nonnull
public static ImmutableList reduceListStatement(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceStatement(reducer, x));
}
@Nonnull
public static ImmutableList reduceListSwitchCase(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceSwitchCase(reducer, x));
}
@Nonnull
public static ImmutableList reduceListVariableDeclarator(
@Nonnull Reducer reducer,
@Nonnull ImmutableList list) {
return list.map(x -> reduceVariableDeclarator(reducer, x));
}
@Nonnull
public static State reduceLiteralBooleanExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralBooleanExpression node) {
return reducer.reduceLiteralBooleanExpression(node);
}
@Nonnull
public static State reduceLiteralInfinityExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralInfinityExpression node) {
return reducer.reduceLiteralInfinityExpression(node);
}
@Nonnull
public static State reduceLiteralNullExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralNullExpression node) {
return reducer.reduceLiteralNullExpression(node);
}
@Nonnull
public static State reduceLiteralNumericExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralNumericExpression node) {
return reducer.reduceLiteralNumericExpression(node);
}
@Nonnull
public static State reduceLiteralRegExpExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralRegExpExpression node) {
return reducer.reduceLiteralRegExpExpression(node);
}
@Nonnull
public static State reduceLiteralStringExpression(
@Nonnull Reducer reducer,
@Nonnull LiteralStringExpression node) {
return reducer.reduceLiteralStringExpression(node);
}
@Nonnull
public static Maybe reduceMaybeAssignmentTarget(
@Nonnull Reducer reducer,
@Nonnull Maybe maybe) {
return maybe.map(x -> reduceAssignmentTarget(reducer, x));
}
@Nonnull
public static Maybe reduceMaybeAssignmentTargetAssignmentTargetWithDefault(
@Nonnull Reducer reducer,
@Nonnull Maybe maybe) {
return maybe.map(x -> reduceAssignmentTargetAssignmentTargetWithDefault(reducer, x));
}
@Nonnull
public static Maybe reduceMaybeBinding(
@Nonnull Reducer reducer,
@Nonnull Maybe maybe) {
return maybe.map(x -> reduceBinding(reducer, x));
}
@Nonnull
public static Maybe reduceMaybeBindingBindingWithDefault(
@Nonnull Reducer reducer,
@Nonnull Maybe maybe) {
return maybe.map(x -> reduceBindingBindingWithDefault(reducer, x));
}
@Nonnull
public static