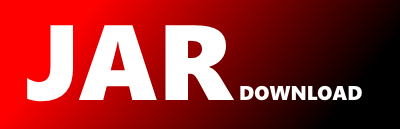
com.shedhack.thread.context.handler.JsonThreadContextHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thread-context-handler Show documentation
Show all versions of thread-context-handler Show documentation
Context enabler, for helping with debugging/logging
package com.shedhack.thread.context.handler;
import com.google.gson.Gson;
import com.shedhack.thread.context.model.ThreadContextModel;
import com.shedhack.thread.context.model.DefaultThreadContextModel;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
/**
* Sets/Gets the thread context as a {@link com.shedhack.thread.context.model.DefaultThreadContextModel}.
* {@link com.google.gson.Gson} is used to convert the object to a JSON string and back.
*
* @author imamchishty
*/
public class JsonThreadContextHandler implements ThreadContextHandler {
private static Gson GSON = new Gson();
private final List afterHandlers;
public JsonThreadContextHandler() {
this.afterHandlers = Collections.EMPTY_LIST;
}
public JsonThreadContextHandler(List afterHandlers) {
this.afterHandlers = afterHandlers;
}
/**
* {@inheritDoc}
*/
public void setThreadContext(ThreadContextModel model) {
if(model != null) {
String converted = GSON.toJson(model);
Thread.currentThread().setName(converted);
// call the after setting handler
this.afterSettingThreadContext(converted, afterHandlers);
}
}
/**
* Returns the {@link com.shedhack.thread.context.model.DefaultThreadContextModel}.
* If for some reason the object cannot be hydrated using GSON then an null {@link java.util.Optional}
* object is returned.
*/
public Optional getThreadContext() {
return convertFromString(Thread.currentThread().getName());
}
/**
* {@inheritDoc}
*/
public Optional convertFromString(String value) {
try {
return Optional.ofNullable(GSON.fromJson(value, DefaultThreadContextModel.class));
}
catch (Exception ex) {
return Optional.empty();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy