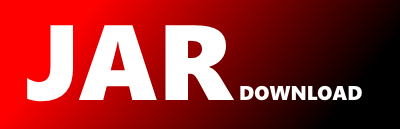
com.shinesolutions.swaggeraem4j.api.SlingApi Maven / Gradle / Ivy
/*
* Adobe Experience Manager (AEM) API
* Swagger AEM is an OpenAPI specification for Adobe Experience Manager (AEM) API
*
* OpenAPI spec version: 2.0.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.shinesolutions.swaggeraem4j.api;
import com.shinesolutions.swaggeraem4j.ApiCallback;
import com.shinesolutions.swaggeraem4j.ApiClient;
import com.shinesolutions.swaggeraem4j.ApiException;
import com.shinesolutions.swaggeraem4j.ApiResponse;
import com.shinesolutions.swaggeraem4j.Configuration;
import com.shinesolutions.swaggeraem4j.Pair;
import com.shinesolutions.swaggeraem4j.ProgressRequestBody;
import com.shinesolutions.swaggeraem4j.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import java.math.BigDecimal;
import java.io.File;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class SlingApi {
private ApiClient apiClient;
public SlingApi() {
this(Configuration.getDefaultApiClient());
}
public SlingApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Build call for deleteAgent
* @param runmode (required)
* @param name (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteAgentCall(String runmode, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/replication/agents.{runmode}/{name}"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteAgentValidateBeforeCall(String runmode, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling deleteAgent(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling deleteAgent(Async)");
}
com.squareup.okhttp.Call call = deleteAgentCall(runmode, name, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteAgent(String runmode, String name) throws ApiException {
deleteAgentWithHttpInfo(runmode, name);
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteAgentWithHttpInfo(String runmode, String name) throws ApiException {
com.squareup.okhttp.Call call = deleteAgentValidateBeforeCall(runmode, name, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param name (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteAgentAsync(String runmode, String name, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteAgentValidateBeforeCall(runmode, name, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for deleteNode
* @param path (required)
* @param name (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call deleteNodeCall(String path, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{path}/{name}"
.replaceAll("\\{" + "path" + "\\}", apiClient.escapeString(path.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call deleteNodeValidateBeforeCall(String path, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling deleteNode(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling deleteNode(Async)");
}
com.squareup.okhttp.Call call = deleteNodeCall(path, name, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param name (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void deleteNode(String path, String name) throws ApiException {
deleteNodeWithHttpInfo(path, name);
}
/**
*
*
* @param path (required)
* @param name (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteNodeWithHttpInfo(String path, String name) throws ApiException {
com.squareup.okhttp.Call call = deleteNodeValidateBeforeCall(path, name, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param path (required)
* @param name (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call deleteNodeAsync(String path, String name, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = deleteNodeValidateBeforeCall(path, name, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getAgent
* @param runmode (required)
* @param name (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getAgentCall(String runmode, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/replication/agents.{runmode}/{name}"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getAgentValidateBeforeCall(String runmode, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling getAgent(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling getAgent(Async)");
}
com.squareup.okhttp.Call call = getAgentCall(runmode, name, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void getAgent(String runmode, String name) throws ApiException {
getAgentWithHttpInfo(runmode, name);
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAgentWithHttpInfo(String runmode, String name) throws ApiException {
com.squareup.okhttp.Call call = getAgentValidateBeforeCall(runmode, name, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param name (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getAgentAsync(String runmode, String name, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getAgentValidateBeforeCall(runmode, name, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getAgents
* @param runmode (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getAgentsCall(String runmode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/replication/agents.{runmode}.-1.json"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getAgentsValidateBeforeCall(String runmode, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling getAgents(Async)");
}
com.squareup.okhttp.Call call = getAgentsCall(runmode, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String getAgents(String runmode) throws ApiException {
ApiResponse resp = getAgentsWithHttpInfo(runmode);
return resp.getData();
}
/**
*
*
* @param runmode (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getAgentsWithHttpInfo(String runmode) throws ApiException {
com.squareup.okhttp.Call call = getAgentsValidateBeforeCall(runmode, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getAgentsAsync(String runmode, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getAgentsValidateBeforeCall(runmode, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getNode
* @param path (required)
* @param name (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getNodeCall(String path, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{path}/{name}"
.replaceAll("\\{" + "path" + "\\}", apiClient.escapeString(path.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getNodeValidateBeforeCall(String path, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling getNode(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling getNode(Async)");
}
com.squareup.okhttp.Call call = getNodeCall(path, name, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param name (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void getNode(String path, String name) throws ApiException {
getNodeWithHttpInfo(path, name);
}
/**
*
*
* @param path (required)
* @param name (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getNodeWithHttpInfo(String path, String name) throws ApiException {
com.squareup.okhttp.Call call = getNodeValidateBeforeCall(path, name, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param path (required)
* @param name (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getNodeAsync(String path, String name, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getNodeValidateBeforeCall(path, name, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for getPackage
* @param group (required)
* @param name (required)
* @param version (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getPackageCall(String group, String name, String version, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/packages/{group}/{name}-{version}.zip"
.replaceAll("\\{" + "group" + "\\}", apiClient.escapeString(group.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()))
.replaceAll("\\{" + "version" + "\\}", apiClient.escapeString(version.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/octet-stream"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getPackageValidateBeforeCall(String group, String name, String version, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'group' is set
if (group == null) {
throw new ApiException("Missing the required parameter 'group' when calling getPackage(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling getPackage(Async)");
}
// verify the required parameter 'version' is set
if (version == null) {
throw new ApiException("Missing the required parameter 'version' when calling getPackage(Async)");
}
com.squareup.okhttp.Call call = getPackageCall(group, name, version, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param group (required)
* @param name (required)
* @param version (required)
* @return File
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public File getPackage(String group, String name, String version) throws ApiException {
ApiResponse resp = getPackageWithHttpInfo(group, name, version);
return resp.getData();
}
/**
*
*
* @param group (required)
* @param name (required)
* @param version (required)
* @return ApiResponse<File>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPackageWithHttpInfo(String group, String name, String version) throws ApiException {
com.squareup.okhttp.Call call = getPackageValidateBeforeCall(group, name, version, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param group (required)
* @param name (required)
* @param version (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getPackageAsync(String group, String name, String version, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getPackageValidateBeforeCall(group, name, version, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getPackageFilter
* @param group (required)
* @param name (required)
* @param version (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getPackageFilterCall(String group, String name, String version, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/packages/{group}/{name}-{version}.zip/jcr:content/vlt:definition/filter.tidy.2.json"
.replaceAll("\\{" + "group" + "\\}", apiClient.escapeString(group.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()))
.replaceAll("\\{" + "version" + "\\}", apiClient.escapeString(version.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getPackageFilterValidateBeforeCall(String group, String name, String version, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'group' is set
if (group == null) {
throw new ApiException("Missing the required parameter 'group' when calling getPackageFilter(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling getPackageFilter(Async)");
}
// verify the required parameter 'version' is set
if (version == null) {
throw new ApiException("Missing the required parameter 'version' when calling getPackageFilter(Async)");
}
com.squareup.okhttp.Call call = getPackageFilterCall(group, name, version, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param group (required)
* @param name (required)
* @param version (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String getPackageFilter(String group, String name, String version) throws ApiException {
ApiResponse resp = getPackageFilterWithHttpInfo(group, name, version);
return resp.getData();
}
/**
*
*
* @param group (required)
* @param name (required)
* @param version (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getPackageFilterWithHttpInfo(String group, String name, String version) throws ApiException {
com.squareup.okhttp.Call call = getPackageFilterValidateBeforeCall(group, name, version, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param group (required)
* @param name (required)
* @param version (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getPackageFilterAsync(String group, String name, String version, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getPackageFilterValidateBeforeCall(group, name, version, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for getQuery
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call getQueryCall(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/bin/querybuilder.json";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (path != null)
localVarQueryParams.addAll(apiClient.parameterToPair("path", path));
if (pLimit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("p.limit", pLimit));
if (_1Property != null)
localVarQueryParams.addAll(apiClient.parameterToPair("1_property", _1Property));
if (_1PropertyValue != null)
localVarQueryParams.addAll(apiClient.parameterToPair("1_property.value", _1PropertyValue));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call getQueryValidateBeforeCall(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling getQuery(Async)");
}
// verify the required parameter 'pLimit' is set
if (pLimit == null) {
throw new ApiException("Missing the required parameter 'pLimit' when calling getQuery(Async)");
}
// verify the required parameter '_1Property' is set
if (_1Property == null) {
throw new ApiException("Missing the required parameter '_1Property' when calling getQuery(Async)");
}
// verify the required parameter '_1PropertyValue' is set
if (_1PropertyValue == null) {
throw new ApiException("Missing the required parameter '_1PropertyValue' when calling getQuery(Async)");
}
com.squareup.okhttp.Call call = getQueryCall(path, pLimit, _1Property, _1PropertyValue, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String getQuery(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue) throws ApiException {
ApiResponse resp = getQueryWithHttpInfo(path, pLimit, _1Property, _1PropertyValue);
return resp.getData();
}
/**
*
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getQueryWithHttpInfo(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue) throws ApiException {
com.squareup.okhttp.Call call = getQueryValidateBeforeCall(path, pLimit, _1Property, _1PropertyValue, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getQueryAsync(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getQueryValidateBeforeCall(path, pLimit, _1Property, _1PropertyValue, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for postAgent
* @param runmode (required)
* @param name (required)
* @param jcrcontentcqdistribute (optional)
* @param jcrcontentcqdistributeTypeHint (optional)
* @param jcrcontentcqname (optional)
* @param jcrcontentcqtemplate (optional)
* @param jcrcontentenabled (optional)
* @param jcrcontentjcrdescription (optional)
* @param jcrcontentjcrlastModified (optional)
* @param jcrcontentjcrlastModifiedBy (optional)
* @param jcrcontentjcrmixinTypes (optional)
* @param jcrcontentjcrtitle (optional)
* @param jcrcontentlogLevel (optional)
* @param jcrcontentnoStatusUpdate (optional)
* @param jcrcontentnoVersioning (optional)
* @param jcrcontentprotocolConnectTimeout (optional)
* @param jcrcontentprotocolHTTPConnectionClosed (optional)
* @param jcrcontentprotocolHTTPExpired (optional)
* @param jcrcontentprotocolHTTPHeaders (optional)
* @param jcrcontentprotocolHTTPHeadersTypeHint (optional)
* @param jcrcontentprotocolHTTPMethod (optional)
* @param jcrcontentprotocolHTTPSRelaxed (optional)
* @param jcrcontentprotocolInterface (optional)
* @param jcrcontentprotocolSocketTimeout (optional)
* @param jcrcontentprotocolVersion (optional)
* @param jcrcontentproxyNTLMDomain (optional)
* @param jcrcontentproxyNTLMHost (optional)
* @param jcrcontentproxyHost (optional)
* @param jcrcontentproxyPassword (optional)
* @param jcrcontentproxyPort (optional)
* @param jcrcontentproxyUser (optional)
* @param jcrcontentqueueBatchMaxSize (optional)
* @param jcrcontentqueueBatchMode (optional)
* @param jcrcontentqueueBatchWaitTime (optional)
* @param jcrcontentretryDelay (optional)
* @param jcrcontentreverseReplication (optional)
* @param jcrcontentserializationType (optional)
* @param jcrcontentslingresourceType (optional)
* @param jcrcontentssl (optional)
* @param jcrcontenttransportNTLMDomain (optional)
* @param jcrcontenttransportNTLMHost (optional)
* @param jcrcontenttransportPassword (optional)
* @param jcrcontenttransportUri (optional)
* @param jcrcontenttransportUser (optional)
* @param jcrcontenttriggerDistribute (optional)
* @param jcrcontenttriggerModified (optional)
* @param jcrcontenttriggerOnOffTime (optional)
* @param jcrcontenttriggerReceive (optional)
* @param jcrcontenttriggerSpecific (optional)
* @param jcrcontentuserId (optional)
* @param jcrprimaryType (optional)
* @param operation (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postAgentCall(String runmode, String name, Boolean jcrcontentcqdistribute, String jcrcontentcqdistributeTypeHint, String jcrcontentcqname, String jcrcontentcqtemplate, Boolean jcrcontentenabled, String jcrcontentjcrdescription, String jcrcontentjcrlastModified, String jcrcontentjcrlastModifiedBy, String jcrcontentjcrmixinTypes, String jcrcontentjcrtitle, String jcrcontentlogLevel, Boolean jcrcontentnoStatusUpdate, Boolean jcrcontentnoVersioning, BigDecimal jcrcontentprotocolConnectTimeout, Boolean jcrcontentprotocolHTTPConnectionClosed, String jcrcontentprotocolHTTPExpired, List jcrcontentprotocolHTTPHeaders, String jcrcontentprotocolHTTPHeadersTypeHint, String jcrcontentprotocolHTTPMethod, Boolean jcrcontentprotocolHTTPSRelaxed, String jcrcontentprotocolInterface, BigDecimal jcrcontentprotocolSocketTimeout, String jcrcontentprotocolVersion, String jcrcontentproxyNTLMDomain, String jcrcontentproxyNTLMHost, String jcrcontentproxyHost, String jcrcontentproxyPassword, BigDecimal jcrcontentproxyPort, String jcrcontentproxyUser, BigDecimal jcrcontentqueueBatchMaxSize, String jcrcontentqueueBatchMode, BigDecimal jcrcontentqueueBatchWaitTime, String jcrcontentretryDelay, Boolean jcrcontentreverseReplication, String jcrcontentserializationType, String jcrcontentslingresourceType, String jcrcontentssl, String jcrcontenttransportNTLMDomain, String jcrcontenttransportNTLMHost, String jcrcontenttransportPassword, String jcrcontenttransportUri, String jcrcontenttransportUser, Boolean jcrcontenttriggerDistribute, Boolean jcrcontenttriggerModified, Boolean jcrcontenttriggerOnOffTime, Boolean jcrcontenttriggerReceive, Boolean jcrcontenttriggerSpecific, String jcrcontentuserId, String jcrprimaryType, String operation, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/replication/agents.{runmode}/{name}"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (jcrcontentcqdistribute != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/cq:distribute", jcrcontentcqdistribute));
if (jcrcontentcqdistributeTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/cq:distribute@TypeHint", jcrcontentcqdistributeTypeHint));
if (jcrcontentcqname != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/cq:name", jcrcontentcqname));
if (jcrcontentcqtemplate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/cq:template", jcrcontentcqtemplate));
if (jcrcontentenabled != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/enabled", jcrcontentenabled));
if (jcrcontentjcrdescription != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/jcr:description", jcrcontentjcrdescription));
if (jcrcontentjcrlastModified != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/jcr:lastModified", jcrcontentjcrlastModified));
if (jcrcontentjcrlastModifiedBy != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/jcr:lastModifiedBy", jcrcontentjcrlastModifiedBy));
if (jcrcontentjcrmixinTypes != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/jcr:mixinTypes", jcrcontentjcrmixinTypes));
if (jcrcontentjcrtitle != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/jcr:title", jcrcontentjcrtitle));
if (jcrcontentlogLevel != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/logLevel", jcrcontentlogLevel));
if (jcrcontentnoStatusUpdate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/noStatusUpdate", jcrcontentnoStatusUpdate));
if (jcrcontentnoVersioning != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/noVersioning", jcrcontentnoVersioning));
if (jcrcontentprotocolConnectTimeout != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolConnectTimeout", jcrcontentprotocolConnectTimeout));
if (jcrcontentprotocolHTTPConnectionClosed != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolHTTPConnectionClosed", jcrcontentprotocolHTTPConnectionClosed));
if (jcrcontentprotocolHTTPExpired != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolHTTPExpired", jcrcontentprotocolHTTPExpired));
if (jcrcontentprotocolHTTPHeaders != null)
localVarCollectionQueryParams.addAll(apiClient.parameterToPairs("multi", "jcr:content/protocolHTTPHeaders", jcrcontentprotocolHTTPHeaders));
if (jcrcontentprotocolHTTPHeadersTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolHTTPHeaders@TypeHint", jcrcontentprotocolHTTPHeadersTypeHint));
if (jcrcontentprotocolHTTPMethod != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolHTTPMethod", jcrcontentprotocolHTTPMethod));
if (jcrcontentprotocolHTTPSRelaxed != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolHTTPSRelaxed", jcrcontentprotocolHTTPSRelaxed));
if (jcrcontentprotocolInterface != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolInterface", jcrcontentprotocolInterface));
if (jcrcontentprotocolSocketTimeout != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolSocketTimeout", jcrcontentprotocolSocketTimeout));
if (jcrcontentprotocolVersion != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/protocolVersion", jcrcontentprotocolVersion));
if (jcrcontentproxyNTLMDomain != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyNTLMDomain", jcrcontentproxyNTLMDomain));
if (jcrcontentproxyNTLMHost != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyNTLMHost", jcrcontentproxyNTLMHost));
if (jcrcontentproxyHost != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyHost", jcrcontentproxyHost));
if (jcrcontentproxyPassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyPassword", jcrcontentproxyPassword));
if (jcrcontentproxyPort != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyPort", jcrcontentproxyPort));
if (jcrcontentproxyUser != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/proxyUser", jcrcontentproxyUser));
if (jcrcontentqueueBatchMaxSize != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/queueBatchMaxSize", jcrcontentqueueBatchMaxSize));
if (jcrcontentqueueBatchMode != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/queueBatchMode", jcrcontentqueueBatchMode));
if (jcrcontentqueueBatchWaitTime != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/queueBatchWaitTime", jcrcontentqueueBatchWaitTime));
if (jcrcontentretryDelay != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/retryDelay", jcrcontentretryDelay));
if (jcrcontentreverseReplication != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/reverseReplication", jcrcontentreverseReplication));
if (jcrcontentserializationType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/serializationType", jcrcontentserializationType));
if (jcrcontentslingresourceType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/sling:resourceType", jcrcontentslingresourceType));
if (jcrcontentssl != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/ssl", jcrcontentssl));
if (jcrcontenttransportNTLMDomain != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/transportNTLMDomain", jcrcontenttransportNTLMDomain));
if (jcrcontenttransportNTLMHost != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/transportNTLMHost", jcrcontenttransportNTLMHost));
if (jcrcontenttransportPassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/transportPassword", jcrcontenttransportPassword));
if (jcrcontenttransportUri != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/transportUri", jcrcontenttransportUri));
if (jcrcontenttransportUser != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/transportUser", jcrcontenttransportUser));
if (jcrcontenttriggerDistribute != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/triggerDistribute", jcrcontenttriggerDistribute));
if (jcrcontenttriggerModified != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/triggerModified", jcrcontenttriggerModified));
if (jcrcontenttriggerOnOffTime != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/triggerOnOffTime", jcrcontenttriggerOnOffTime));
if (jcrcontenttriggerReceive != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/triggerReceive", jcrcontenttriggerReceive));
if (jcrcontenttriggerSpecific != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/triggerSpecific", jcrcontenttriggerSpecific));
if (jcrcontentuserId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:content/userId", jcrcontentuserId));
if (jcrprimaryType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:primaryType", jcrprimaryType));
if (operation != null)
localVarQueryParams.addAll(apiClient.parameterToPair(":operation", operation));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postAgentValidateBeforeCall(String runmode, String name, Boolean jcrcontentcqdistribute, String jcrcontentcqdistributeTypeHint, String jcrcontentcqname, String jcrcontentcqtemplate, Boolean jcrcontentenabled, String jcrcontentjcrdescription, String jcrcontentjcrlastModified, String jcrcontentjcrlastModifiedBy, String jcrcontentjcrmixinTypes, String jcrcontentjcrtitle, String jcrcontentlogLevel, Boolean jcrcontentnoStatusUpdate, Boolean jcrcontentnoVersioning, BigDecimal jcrcontentprotocolConnectTimeout, Boolean jcrcontentprotocolHTTPConnectionClosed, String jcrcontentprotocolHTTPExpired, List jcrcontentprotocolHTTPHeaders, String jcrcontentprotocolHTTPHeadersTypeHint, String jcrcontentprotocolHTTPMethod, Boolean jcrcontentprotocolHTTPSRelaxed, String jcrcontentprotocolInterface, BigDecimal jcrcontentprotocolSocketTimeout, String jcrcontentprotocolVersion, String jcrcontentproxyNTLMDomain, String jcrcontentproxyNTLMHost, String jcrcontentproxyHost, String jcrcontentproxyPassword, BigDecimal jcrcontentproxyPort, String jcrcontentproxyUser, BigDecimal jcrcontentqueueBatchMaxSize, String jcrcontentqueueBatchMode, BigDecimal jcrcontentqueueBatchWaitTime, String jcrcontentretryDelay, Boolean jcrcontentreverseReplication, String jcrcontentserializationType, String jcrcontentslingresourceType, String jcrcontentssl, String jcrcontenttransportNTLMDomain, String jcrcontenttransportNTLMHost, String jcrcontenttransportPassword, String jcrcontenttransportUri, String jcrcontenttransportUser, Boolean jcrcontenttriggerDistribute, Boolean jcrcontenttriggerModified, Boolean jcrcontenttriggerOnOffTime, Boolean jcrcontenttriggerReceive, Boolean jcrcontenttriggerSpecific, String jcrcontentuserId, String jcrprimaryType, String operation, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling postAgent(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling postAgent(Async)");
}
com.squareup.okhttp.Call call = postAgentCall(runmode, name, jcrcontentcqdistribute, jcrcontentcqdistributeTypeHint, jcrcontentcqname, jcrcontentcqtemplate, jcrcontentenabled, jcrcontentjcrdescription, jcrcontentjcrlastModified, jcrcontentjcrlastModifiedBy, jcrcontentjcrmixinTypes, jcrcontentjcrtitle, jcrcontentlogLevel, jcrcontentnoStatusUpdate, jcrcontentnoVersioning, jcrcontentprotocolConnectTimeout, jcrcontentprotocolHTTPConnectionClosed, jcrcontentprotocolHTTPExpired, jcrcontentprotocolHTTPHeaders, jcrcontentprotocolHTTPHeadersTypeHint, jcrcontentprotocolHTTPMethod, jcrcontentprotocolHTTPSRelaxed, jcrcontentprotocolInterface, jcrcontentprotocolSocketTimeout, jcrcontentprotocolVersion, jcrcontentproxyNTLMDomain, jcrcontentproxyNTLMHost, jcrcontentproxyHost, jcrcontentproxyPassword, jcrcontentproxyPort, jcrcontentproxyUser, jcrcontentqueueBatchMaxSize, jcrcontentqueueBatchMode, jcrcontentqueueBatchWaitTime, jcrcontentretryDelay, jcrcontentreverseReplication, jcrcontentserializationType, jcrcontentslingresourceType, jcrcontentssl, jcrcontenttransportNTLMDomain, jcrcontenttransportNTLMHost, jcrcontenttransportPassword, jcrcontenttransportUri, jcrcontenttransportUser, jcrcontenttriggerDistribute, jcrcontenttriggerModified, jcrcontenttriggerOnOffTime, jcrcontenttriggerReceive, jcrcontenttriggerSpecific, jcrcontentuserId, jcrprimaryType, operation, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @param jcrcontentcqdistribute (optional)
* @param jcrcontentcqdistributeTypeHint (optional)
* @param jcrcontentcqname (optional)
* @param jcrcontentcqtemplate (optional)
* @param jcrcontentenabled (optional)
* @param jcrcontentjcrdescription (optional)
* @param jcrcontentjcrlastModified (optional)
* @param jcrcontentjcrlastModifiedBy (optional)
* @param jcrcontentjcrmixinTypes (optional)
* @param jcrcontentjcrtitle (optional)
* @param jcrcontentlogLevel (optional)
* @param jcrcontentnoStatusUpdate (optional)
* @param jcrcontentnoVersioning (optional)
* @param jcrcontentprotocolConnectTimeout (optional)
* @param jcrcontentprotocolHTTPConnectionClosed (optional)
* @param jcrcontentprotocolHTTPExpired (optional)
* @param jcrcontentprotocolHTTPHeaders (optional)
* @param jcrcontentprotocolHTTPHeadersTypeHint (optional)
* @param jcrcontentprotocolHTTPMethod (optional)
* @param jcrcontentprotocolHTTPSRelaxed (optional)
* @param jcrcontentprotocolInterface (optional)
* @param jcrcontentprotocolSocketTimeout (optional)
* @param jcrcontentprotocolVersion (optional)
* @param jcrcontentproxyNTLMDomain (optional)
* @param jcrcontentproxyNTLMHost (optional)
* @param jcrcontentproxyHost (optional)
* @param jcrcontentproxyPassword (optional)
* @param jcrcontentproxyPort (optional)
* @param jcrcontentproxyUser (optional)
* @param jcrcontentqueueBatchMaxSize (optional)
* @param jcrcontentqueueBatchMode (optional)
* @param jcrcontentqueueBatchWaitTime (optional)
* @param jcrcontentretryDelay (optional)
* @param jcrcontentreverseReplication (optional)
* @param jcrcontentserializationType (optional)
* @param jcrcontentslingresourceType (optional)
* @param jcrcontentssl (optional)
* @param jcrcontenttransportNTLMDomain (optional)
* @param jcrcontenttransportNTLMHost (optional)
* @param jcrcontenttransportPassword (optional)
* @param jcrcontenttransportUri (optional)
* @param jcrcontenttransportUser (optional)
* @param jcrcontenttriggerDistribute (optional)
* @param jcrcontenttriggerModified (optional)
* @param jcrcontenttriggerOnOffTime (optional)
* @param jcrcontenttriggerReceive (optional)
* @param jcrcontenttriggerSpecific (optional)
* @param jcrcontentuserId (optional)
* @param jcrprimaryType (optional)
* @param operation (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postAgent(String runmode, String name, Boolean jcrcontentcqdistribute, String jcrcontentcqdistributeTypeHint, String jcrcontentcqname, String jcrcontentcqtemplate, Boolean jcrcontentenabled, String jcrcontentjcrdescription, String jcrcontentjcrlastModified, String jcrcontentjcrlastModifiedBy, String jcrcontentjcrmixinTypes, String jcrcontentjcrtitle, String jcrcontentlogLevel, Boolean jcrcontentnoStatusUpdate, Boolean jcrcontentnoVersioning, BigDecimal jcrcontentprotocolConnectTimeout, Boolean jcrcontentprotocolHTTPConnectionClosed, String jcrcontentprotocolHTTPExpired, List jcrcontentprotocolHTTPHeaders, String jcrcontentprotocolHTTPHeadersTypeHint, String jcrcontentprotocolHTTPMethod, Boolean jcrcontentprotocolHTTPSRelaxed, String jcrcontentprotocolInterface, BigDecimal jcrcontentprotocolSocketTimeout, String jcrcontentprotocolVersion, String jcrcontentproxyNTLMDomain, String jcrcontentproxyNTLMHost, String jcrcontentproxyHost, String jcrcontentproxyPassword, BigDecimal jcrcontentproxyPort, String jcrcontentproxyUser, BigDecimal jcrcontentqueueBatchMaxSize, String jcrcontentqueueBatchMode, BigDecimal jcrcontentqueueBatchWaitTime, String jcrcontentretryDelay, Boolean jcrcontentreverseReplication, String jcrcontentserializationType, String jcrcontentslingresourceType, String jcrcontentssl, String jcrcontenttransportNTLMDomain, String jcrcontenttransportNTLMHost, String jcrcontenttransportPassword, String jcrcontenttransportUri, String jcrcontenttransportUser, Boolean jcrcontenttriggerDistribute, Boolean jcrcontenttriggerModified, Boolean jcrcontenttriggerOnOffTime, Boolean jcrcontenttriggerReceive, Boolean jcrcontenttriggerSpecific, String jcrcontentuserId, String jcrprimaryType, String operation) throws ApiException {
postAgentWithHttpInfo(runmode, name, jcrcontentcqdistribute, jcrcontentcqdistributeTypeHint, jcrcontentcqname, jcrcontentcqtemplate, jcrcontentenabled, jcrcontentjcrdescription, jcrcontentjcrlastModified, jcrcontentjcrlastModifiedBy, jcrcontentjcrmixinTypes, jcrcontentjcrtitle, jcrcontentlogLevel, jcrcontentnoStatusUpdate, jcrcontentnoVersioning, jcrcontentprotocolConnectTimeout, jcrcontentprotocolHTTPConnectionClosed, jcrcontentprotocolHTTPExpired, jcrcontentprotocolHTTPHeaders, jcrcontentprotocolHTTPHeadersTypeHint, jcrcontentprotocolHTTPMethod, jcrcontentprotocolHTTPSRelaxed, jcrcontentprotocolInterface, jcrcontentprotocolSocketTimeout, jcrcontentprotocolVersion, jcrcontentproxyNTLMDomain, jcrcontentproxyNTLMHost, jcrcontentproxyHost, jcrcontentproxyPassword, jcrcontentproxyPort, jcrcontentproxyUser, jcrcontentqueueBatchMaxSize, jcrcontentqueueBatchMode, jcrcontentqueueBatchWaitTime, jcrcontentretryDelay, jcrcontentreverseReplication, jcrcontentserializationType, jcrcontentslingresourceType, jcrcontentssl, jcrcontenttransportNTLMDomain, jcrcontenttransportNTLMHost, jcrcontenttransportPassword, jcrcontenttransportUri, jcrcontenttransportUser, jcrcontenttriggerDistribute, jcrcontenttriggerModified, jcrcontenttriggerOnOffTime, jcrcontenttriggerReceive, jcrcontenttriggerSpecific, jcrcontentuserId, jcrprimaryType, operation);
}
/**
*
*
* @param runmode (required)
* @param name (required)
* @param jcrcontentcqdistribute (optional)
* @param jcrcontentcqdistributeTypeHint (optional)
* @param jcrcontentcqname (optional)
* @param jcrcontentcqtemplate (optional)
* @param jcrcontentenabled (optional)
* @param jcrcontentjcrdescription (optional)
* @param jcrcontentjcrlastModified (optional)
* @param jcrcontentjcrlastModifiedBy (optional)
* @param jcrcontentjcrmixinTypes (optional)
* @param jcrcontentjcrtitle (optional)
* @param jcrcontentlogLevel (optional)
* @param jcrcontentnoStatusUpdate (optional)
* @param jcrcontentnoVersioning (optional)
* @param jcrcontentprotocolConnectTimeout (optional)
* @param jcrcontentprotocolHTTPConnectionClosed (optional)
* @param jcrcontentprotocolHTTPExpired (optional)
* @param jcrcontentprotocolHTTPHeaders (optional)
* @param jcrcontentprotocolHTTPHeadersTypeHint (optional)
* @param jcrcontentprotocolHTTPMethod (optional)
* @param jcrcontentprotocolHTTPSRelaxed (optional)
* @param jcrcontentprotocolInterface (optional)
* @param jcrcontentprotocolSocketTimeout (optional)
* @param jcrcontentprotocolVersion (optional)
* @param jcrcontentproxyNTLMDomain (optional)
* @param jcrcontentproxyNTLMHost (optional)
* @param jcrcontentproxyHost (optional)
* @param jcrcontentproxyPassword (optional)
* @param jcrcontentproxyPort (optional)
* @param jcrcontentproxyUser (optional)
* @param jcrcontentqueueBatchMaxSize (optional)
* @param jcrcontentqueueBatchMode (optional)
* @param jcrcontentqueueBatchWaitTime (optional)
* @param jcrcontentretryDelay (optional)
* @param jcrcontentreverseReplication (optional)
* @param jcrcontentserializationType (optional)
* @param jcrcontentslingresourceType (optional)
* @param jcrcontentssl (optional)
* @param jcrcontenttransportNTLMDomain (optional)
* @param jcrcontenttransportNTLMHost (optional)
* @param jcrcontenttransportPassword (optional)
* @param jcrcontenttransportUri (optional)
* @param jcrcontenttransportUser (optional)
* @param jcrcontenttriggerDistribute (optional)
* @param jcrcontenttriggerModified (optional)
* @param jcrcontenttriggerOnOffTime (optional)
* @param jcrcontenttriggerReceive (optional)
* @param jcrcontenttriggerSpecific (optional)
* @param jcrcontentuserId (optional)
* @param jcrprimaryType (optional)
* @param operation (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postAgentWithHttpInfo(String runmode, String name, Boolean jcrcontentcqdistribute, String jcrcontentcqdistributeTypeHint, String jcrcontentcqname, String jcrcontentcqtemplate, Boolean jcrcontentenabled, String jcrcontentjcrdescription, String jcrcontentjcrlastModified, String jcrcontentjcrlastModifiedBy, String jcrcontentjcrmixinTypes, String jcrcontentjcrtitle, String jcrcontentlogLevel, Boolean jcrcontentnoStatusUpdate, Boolean jcrcontentnoVersioning, BigDecimal jcrcontentprotocolConnectTimeout, Boolean jcrcontentprotocolHTTPConnectionClosed, String jcrcontentprotocolHTTPExpired, List jcrcontentprotocolHTTPHeaders, String jcrcontentprotocolHTTPHeadersTypeHint, String jcrcontentprotocolHTTPMethod, Boolean jcrcontentprotocolHTTPSRelaxed, String jcrcontentprotocolInterface, BigDecimal jcrcontentprotocolSocketTimeout, String jcrcontentprotocolVersion, String jcrcontentproxyNTLMDomain, String jcrcontentproxyNTLMHost, String jcrcontentproxyHost, String jcrcontentproxyPassword, BigDecimal jcrcontentproxyPort, String jcrcontentproxyUser, BigDecimal jcrcontentqueueBatchMaxSize, String jcrcontentqueueBatchMode, BigDecimal jcrcontentqueueBatchWaitTime, String jcrcontentretryDelay, Boolean jcrcontentreverseReplication, String jcrcontentserializationType, String jcrcontentslingresourceType, String jcrcontentssl, String jcrcontenttransportNTLMDomain, String jcrcontenttransportNTLMHost, String jcrcontenttransportPassword, String jcrcontenttransportUri, String jcrcontenttransportUser, Boolean jcrcontenttriggerDistribute, Boolean jcrcontenttriggerModified, Boolean jcrcontenttriggerOnOffTime, Boolean jcrcontenttriggerReceive, Boolean jcrcontenttriggerSpecific, String jcrcontentuserId, String jcrprimaryType, String operation) throws ApiException {
com.squareup.okhttp.Call call = postAgentValidateBeforeCall(runmode, name, jcrcontentcqdistribute, jcrcontentcqdistributeTypeHint, jcrcontentcqname, jcrcontentcqtemplate, jcrcontentenabled, jcrcontentjcrdescription, jcrcontentjcrlastModified, jcrcontentjcrlastModifiedBy, jcrcontentjcrmixinTypes, jcrcontentjcrtitle, jcrcontentlogLevel, jcrcontentnoStatusUpdate, jcrcontentnoVersioning, jcrcontentprotocolConnectTimeout, jcrcontentprotocolHTTPConnectionClosed, jcrcontentprotocolHTTPExpired, jcrcontentprotocolHTTPHeaders, jcrcontentprotocolHTTPHeadersTypeHint, jcrcontentprotocolHTTPMethod, jcrcontentprotocolHTTPSRelaxed, jcrcontentprotocolInterface, jcrcontentprotocolSocketTimeout, jcrcontentprotocolVersion, jcrcontentproxyNTLMDomain, jcrcontentproxyNTLMHost, jcrcontentproxyHost, jcrcontentproxyPassword, jcrcontentproxyPort, jcrcontentproxyUser, jcrcontentqueueBatchMaxSize, jcrcontentqueueBatchMode, jcrcontentqueueBatchWaitTime, jcrcontentretryDelay, jcrcontentreverseReplication, jcrcontentserializationType, jcrcontentslingresourceType, jcrcontentssl, jcrcontenttransportNTLMDomain, jcrcontenttransportNTLMHost, jcrcontenttransportPassword, jcrcontenttransportUri, jcrcontenttransportUser, jcrcontenttriggerDistribute, jcrcontenttriggerModified, jcrcontenttriggerOnOffTime, jcrcontenttriggerReceive, jcrcontenttriggerSpecific, jcrcontentuserId, jcrprimaryType, operation, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param name (required)
* @param jcrcontentcqdistribute (optional)
* @param jcrcontentcqdistributeTypeHint (optional)
* @param jcrcontentcqname (optional)
* @param jcrcontentcqtemplate (optional)
* @param jcrcontentenabled (optional)
* @param jcrcontentjcrdescription (optional)
* @param jcrcontentjcrlastModified (optional)
* @param jcrcontentjcrlastModifiedBy (optional)
* @param jcrcontentjcrmixinTypes (optional)
* @param jcrcontentjcrtitle (optional)
* @param jcrcontentlogLevel (optional)
* @param jcrcontentnoStatusUpdate (optional)
* @param jcrcontentnoVersioning (optional)
* @param jcrcontentprotocolConnectTimeout (optional)
* @param jcrcontentprotocolHTTPConnectionClosed (optional)
* @param jcrcontentprotocolHTTPExpired (optional)
* @param jcrcontentprotocolHTTPHeaders (optional)
* @param jcrcontentprotocolHTTPHeadersTypeHint (optional)
* @param jcrcontentprotocolHTTPMethod (optional)
* @param jcrcontentprotocolHTTPSRelaxed (optional)
* @param jcrcontentprotocolInterface (optional)
* @param jcrcontentprotocolSocketTimeout (optional)
* @param jcrcontentprotocolVersion (optional)
* @param jcrcontentproxyNTLMDomain (optional)
* @param jcrcontentproxyNTLMHost (optional)
* @param jcrcontentproxyHost (optional)
* @param jcrcontentproxyPassword (optional)
* @param jcrcontentproxyPort (optional)
* @param jcrcontentproxyUser (optional)
* @param jcrcontentqueueBatchMaxSize (optional)
* @param jcrcontentqueueBatchMode (optional)
* @param jcrcontentqueueBatchWaitTime (optional)
* @param jcrcontentretryDelay (optional)
* @param jcrcontentreverseReplication (optional)
* @param jcrcontentserializationType (optional)
* @param jcrcontentslingresourceType (optional)
* @param jcrcontentssl (optional)
* @param jcrcontenttransportNTLMDomain (optional)
* @param jcrcontenttransportNTLMHost (optional)
* @param jcrcontenttransportPassword (optional)
* @param jcrcontenttransportUri (optional)
* @param jcrcontenttransportUser (optional)
* @param jcrcontenttriggerDistribute (optional)
* @param jcrcontenttriggerModified (optional)
* @param jcrcontenttriggerOnOffTime (optional)
* @param jcrcontenttriggerReceive (optional)
* @param jcrcontenttriggerSpecific (optional)
* @param jcrcontentuserId (optional)
* @param jcrprimaryType (optional)
* @param operation (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postAgentAsync(String runmode, String name, Boolean jcrcontentcqdistribute, String jcrcontentcqdistributeTypeHint, String jcrcontentcqname, String jcrcontentcqtemplate, Boolean jcrcontentenabled, String jcrcontentjcrdescription, String jcrcontentjcrlastModified, String jcrcontentjcrlastModifiedBy, String jcrcontentjcrmixinTypes, String jcrcontentjcrtitle, String jcrcontentlogLevel, Boolean jcrcontentnoStatusUpdate, Boolean jcrcontentnoVersioning, BigDecimal jcrcontentprotocolConnectTimeout, Boolean jcrcontentprotocolHTTPConnectionClosed, String jcrcontentprotocolHTTPExpired, List jcrcontentprotocolHTTPHeaders, String jcrcontentprotocolHTTPHeadersTypeHint, String jcrcontentprotocolHTTPMethod, Boolean jcrcontentprotocolHTTPSRelaxed, String jcrcontentprotocolInterface, BigDecimal jcrcontentprotocolSocketTimeout, String jcrcontentprotocolVersion, String jcrcontentproxyNTLMDomain, String jcrcontentproxyNTLMHost, String jcrcontentproxyHost, String jcrcontentproxyPassword, BigDecimal jcrcontentproxyPort, String jcrcontentproxyUser, BigDecimal jcrcontentqueueBatchMaxSize, String jcrcontentqueueBatchMode, BigDecimal jcrcontentqueueBatchWaitTime, String jcrcontentretryDelay, Boolean jcrcontentreverseReplication, String jcrcontentserializationType, String jcrcontentslingresourceType, String jcrcontentssl, String jcrcontenttransportNTLMDomain, String jcrcontenttransportNTLMHost, String jcrcontenttransportPassword, String jcrcontenttransportUri, String jcrcontenttransportUser, Boolean jcrcontenttriggerDistribute, Boolean jcrcontenttriggerModified, Boolean jcrcontenttriggerOnOffTime, Boolean jcrcontenttriggerReceive, Boolean jcrcontenttriggerSpecific, String jcrcontentuserId, String jcrprimaryType, String operation, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postAgentValidateBeforeCall(runmode, name, jcrcontentcqdistribute, jcrcontentcqdistributeTypeHint, jcrcontentcqname, jcrcontentcqtemplate, jcrcontentenabled, jcrcontentjcrdescription, jcrcontentjcrlastModified, jcrcontentjcrlastModifiedBy, jcrcontentjcrmixinTypes, jcrcontentjcrtitle, jcrcontentlogLevel, jcrcontentnoStatusUpdate, jcrcontentnoVersioning, jcrcontentprotocolConnectTimeout, jcrcontentprotocolHTTPConnectionClosed, jcrcontentprotocolHTTPExpired, jcrcontentprotocolHTTPHeaders, jcrcontentprotocolHTTPHeadersTypeHint, jcrcontentprotocolHTTPMethod, jcrcontentprotocolHTTPSRelaxed, jcrcontentprotocolInterface, jcrcontentprotocolSocketTimeout, jcrcontentprotocolVersion, jcrcontentproxyNTLMDomain, jcrcontentproxyNTLMHost, jcrcontentproxyHost, jcrcontentproxyPassword, jcrcontentproxyPort, jcrcontentproxyUser, jcrcontentqueueBatchMaxSize, jcrcontentqueueBatchMode, jcrcontentqueueBatchWaitTime, jcrcontentretryDelay, jcrcontentreverseReplication, jcrcontentserializationType, jcrcontentslingresourceType, jcrcontentssl, jcrcontenttransportNTLMDomain, jcrcontenttransportNTLMHost, jcrcontenttransportPassword, jcrcontenttransportUri, jcrcontenttransportUser, jcrcontenttriggerDistribute, jcrcontenttriggerModified, jcrcontenttriggerOnOffTime, jcrcontenttriggerReceive, jcrcontenttriggerSpecific, jcrcontentuserId, jcrprimaryType, operation, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postAuthorizables
* @param authorizableId (required)
* @param intermediatePath (required)
* @param createUser (optional)
* @param createGroup (optional)
* @param reppassword (optional)
* @param profilegivenName (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postAuthorizablesCall(String authorizableId, String intermediatePath, String createUser, String createGroup, String reppassword, String profilegivenName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/libs/granite/security/post/authorizables";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (authorizableId != null)
localVarQueryParams.addAll(apiClient.parameterToPair("authorizableId", authorizableId));
if (intermediatePath != null)
localVarQueryParams.addAll(apiClient.parameterToPair("intermediatePath", intermediatePath));
if (createUser != null)
localVarQueryParams.addAll(apiClient.parameterToPair("createUser", createUser));
if (createGroup != null)
localVarQueryParams.addAll(apiClient.parameterToPair("createGroup", createGroup));
if (reppassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("rep:password", reppassword));
if (profilegivenName != null)
localVarQueryParams.addAll(apiClient.parameterToPair("profile/givenName", profilegivenName));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/html"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postAuthorizablesValidateBeforeCall(String authorizableId, String intermediatePath, String createUser, String createGroup, String reppassword, String profilegivenName, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'authorizableId' is set
if (authorizableId == null) {
throw new ApiException("Missing the required parameter 'authorizableId' when calling postAuthorizables(Async)");
}
// verify the required parameter 'intermediatePath' is set
if (intermediatePath == null) {
throw new ApiException("Missing the required parameter 'intermediatePath' when calling postAuthorizables(Async)");
}
com.squareup.okhttp.Call call = postAuthorizablesCall(authorizableId, intermediatePath, createUser, createGroup, reppassword, profilegivenName, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param authorizableId (required)
* @param intermediatePath (required)
* @param createUser (optional)
* @param createGroup (optional)
* @param reppassword (optional)
* @param profilegivenName (optional)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String postAuthorizables(String authorizableId, String intermediatePath, String createUser, String createGroup, String reppassword, String profilegivenName) throws ApiException {
ApiResponse resp = postAuthorizablesWithHttpInfo(authorizableId, intermediatePath, createUser, createGroup, reppassword, profilegivenName);
return resp.getData();
}
/**
*
*
* @param authorizableId (required)
* @param intermediatePath (required)
* @param createUser (optional)
* @param createGroup (optional)
* @param reppassword (optional)
* @param profilegivenName (optional)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postAuthorizablesWithHttpInfo(String authorizableId, String intermediatePath, String createUser, String createGroup, String reppassword, String profilegivenName) throws ApiException {
com.squareup.okhttp.Call call = postAuthorizablesValidateBeforeCall(authorizableId, intermediatePath, createUser, createGroup, reppassword, profilegivenName, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param authorizableId (required)
* @param intermediatePath (required)
* @param createUser (optional)
* @param createGroup (optional)
* @param reppassword (optional)
* @param profilegivenName (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postAuthorizablesAsync(String authorizableId, String intermediatePath, String createUser, String createGroup, String reppassword, String profilegivenName, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postAuthorizablesValidateBeforeCall(authorizableId, intermediatePath, createUser, createGroup, reppassword, profilegivenName, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for postConfigApacheFelixJettyBasedHttpService
* @param runmode (required)
* @param orgApacheFelixHttpsNio (optional)
* @param orgApacheFelixHttpsNioTypeHint (optional)
* @param orgApacheFelixHttpsKeystore (optional)
* @param orgApacheFelixHttpsKeystoreTypeHint (optional)
* @param orgApacheFelixHttpsKeystorePassword (optional)
* @param orgApacheFelixHttpsKeystorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKey (optional)
* @param orgApacheFelixHttpsKeystoreKeyTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKeyPassword (optional)
* @param orgApacheFelixHttpsKeystoreKeyPasswordTypeHint (optional)
* @param orgApacheFelixHttpsTruststore (optional)
* @param orgApacheFelixHttpsTruststoreTypeHint (optional)
* @param orgApacheFelixHttpsTruststorePassword (optional)
* @param orgApacheFelixHttpsTruststorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsClientcertificate (optional)
* @param orgApacheFelixHttpsClientcertificateTypeHint (optional)
* @param orgApacheFelixHttpsEnable (optional)
* @param orgApacheFelixHttpsEnableTypeHint (optional)
* @param orgOsgiServiceHttpPortSecure (optional)
* @param orgOsgiServiceHttpPortSecureTypeHint (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postConfigApacheFelixJettyBasedHttpServiceCall(String runmode, Boolean orgApacheFelixHttpsNio, String orgApacheFelixHttpsNioTypeHint, String orgApacheFelixHttpsKeystore, String orgApacheFelixHttpsKeystoreTypeHint, String orgApacheFelixHttpsKeystorePassword, String orgApacheFelixHttpsKeystorePasswordTypeHint, String orgApacheFelixHttpsKeystoreKey, String orgApacheFelixHttpsKeystoreKeyTypeHint, String orgApacheFelixHttpsKeystoreKeyPassword, String orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, String orgApacheFelixHttpsTruststore, String orgApacheFelixHttpsTruststoreTypeHint, String orgApacheFelixHttpsTruststorePassword, String orgApacheFelixHttpsTruststorePasswordTypeHint, String orgApacheFelixHttpsClientcertificate, String orgApacheFelixHttpsClientcertificateTypeHint, Boolean orgApacheFelixHttpsEnable, String orgApacheFelixHttpsEnableTypeHint, String orgOsgiServiceHttpPortSecure, String orgOsgiServiceHttpPortSecureTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/apps/system/config/org.apache.felix.http"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (orgApacheFelixHttpsNio != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.nio", orgApacheFelixHttpsNio));
if (orgApacheFelixHttpsNioTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.nio@TypeHint", orgApacheFelixHttpsNioTypeHint));
if (orgApacheFelixHttpsKeystore != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore", orgApacheFelixHttpsKeystore));
if (orgApacheFelixHttpsKeystoreTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore@TypeHint", orgApacheFelixHttpsKeystoreTypeHint));
if (orgApacheFelixHttpsKeystorePassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.password", orgApacheFelixHttpsKeystorePassword));
if (orgApacheFelixHttpsKeystorePasswordTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.password@TypeHint", orgApacheFelixHttpsKeystorePasswordTypeHint));
if (orgApacheFelixHttpsKeystoreKey != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.key", orgApacheFelixHttpsKeystoreKey));
if (orgApacheFelixHttpsKeystoreKeyTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.key@TypeHint", orgApacheFelixHttpsKeystoreKeyTypeHint));
if (orgApacheFelixHttpsKeystoreKeyPassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.key.password", orgApacheFelixHttpsKeystoreKeyPassword));
if (orgApacheFelixHttpsKeystoreKeyPasswordTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.keystore.key.password@TypeHint", orgApacheFelixHttpsKeystoreKeyPasswordTypeHint));
if (orgApacheFelixHttpsTruststore != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.truststore", orgApacheFelixHttpsTruststore));
if (orgApacheFelixHttpsTruststoreTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.truststore@TypeHint", orgApacheFelixHttpsTruststoreTypeHint));
if (orgApacheFelixHttpsTruststorePassword != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.truststore.password", orgApacheFelixHttpsTruststorePassword));
if (orgApacheFelixHttpsTruststorePasswordTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.truststore.password@TypeHint", orgApacheFelixHttpsTruststorePasswordTypeHint));
if (orgApacheFelixHttpsClientcertificate != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.clientcertificate", orgApacheFelixHttpsClientcertificate));
if (orgApacheFelixHttpsClientcertificateTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.clientcertificate@TypeHint", orgApacheFelixHttpsClientcertificateTypeHint));
if (orgApacheFelixHttpsEnable != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.enable", orgApacheFelixHttpsEnable));
if (orgApacheFelixHttpsEnableTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.apache.felix.https.enable@TypeHint", orgApacheFelixHttpsEnableTypeHint));
if (orgOsgiServiceHttpPortSecure != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.osgi.service.http.port.secure", orgOsgiServiceHttpPortSecure));
if (orgOsgiServiceHttpPortSecureTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("org.osgi.service.http.port.secure@TypeHint", orgOsgiServiceHttpPortSecureTypeHint));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postConfigApacheFelixJettyBasedHttpServiceValidateBeforeCall(String runmode, Boolean orgApacheFelixHttpsNio, String orgApacheFelixHttpsNioTypeHint, String orgApacheFelixHttpsKeystore, String orgApacheFelixHttpsKeystoreTypeHint, String orgApacheFelixHttpsKeystorePassword, String orgApacheFelixHttpsKeystorePasswordTypeHint, String orgApacheFelixHttpsKeystoreKey, String orgApacheFelixHttpsKeystoreKeyTypeHint, String orgApacheFelixHttpsKeystoreKeyPassword, String orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, String orgApacheFelixHttpsTruststore, String orgApacheFelixHttpsTruststoreTypeHint, String orgApacheFelixHttpsTruststorePassword, String orgApacheFelixHttpsTruststorePasswordTypeHint, String orgApacheFelixHttpsClientcertificate, String orgApacheFelixHttpsClientcertificateTypeHint, Boolean orgApacheFelixHttpsEnable, String orgApacheFelixHttpsEnableTypeHint, String orgOsgiServiceHttpPortSecure, String orgOsgiServiceHttpPortSecureTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling postConfigApacheFelixJettyBasedHttpService(Async)");
}
com.squareup.okhttp.Call call = postConfigApacheFelixJettyBasedHttpServiceCall(runmode, orgApacheFelixHttpsNio, orgApacheFelixHttpsNioTypeHint, orgApacheFelixHttpsKeystore, orgApacheFelixHttpsKeystoreTypeHint, orgApacheFelixHttpsKeystorePassword, orgApacheFelixHttpsKeystorePasswordTypeHint, orgApacheFelixHttpsKeystoreKey, orgApacheFelixHttpsKeystoreKeyTypeHint, orgApacheFelixHttpsKeystoreKeyPassword, orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, orgApacheFelixHttpsTruststore, orgApacheFelixHttpsTruststoreTypeHint, orgApacheFelixHttpsTruststorePassword, orgApacheFelixHttpsTruststorePasswordTypeHint, orgApacheFelixHttpsClientcertificate, orgApacheFelixHttpsClientcertificateTypeHint, orgApacheFelixHttpsEnable, orgApacheFelixHttpsEnableTypeHint, orgOsgiServiceHttpPortSecure, orgOsgiServiceHttpPortSecureTypeHint, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param orgApacheFelixHttpsNio (optional)
* @param orgApacheFelixHttpsNioTypeHint (optional)
* @param orgApacheFelixHttpsKeystore (optional)
* @param orgApacheFelixHttpsKeystoreTypeHint (optional)
* @param orgApacheFelixHttpsKeystorePassword (optional)
* @param orgApacheFelixHttpsKeystorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKey (optional)
* @param orgApacheFelixHttpsKeystoreKeyTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKeyPassword (optional)
* @param orgApacheFelixHttpsKeystoreKeyPasswordTypeHint (optional)
* @param orgApacheFelixHttpsTruststore (optional)
* @param orgApacheFelixHttpsTruststoreTypeHint (optional)
* @param orgApacheFelixHttpsTruststorePassword (optional)
* @param orgApacheFelixHttpsTruststorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsClientcertificate (optional)
* @param orgApacheFelixHttpsClientcertificateTypeHint (optional)
* @param orgApacheFelixHttpsEnable (optional)
* @param orgApacheFelixHttpsEnableTypeHint (optional)
* @param orgOsgiServiceHttpPortSecure (optional)
* @param orgOsgiServiceHttpPortSecureTypeHint (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postConfigApacheFelixJettyBasedHttpService(String runmode, Boolean orgApacheFelixHttpsNio, String orgApacheFelixHttpsNioTypeHint, String orgApacheFelixHttpsKeystore, String orgApacheFelixHttpsKeystoreTypeHint, String orgApacheFelixHttpsKeystorePassword, String orgApacheFelixHttpsKeystorePasswordTypeHint, String orgApacheFelixHttpsKeystoreKey, String orgApacheFelixHttpsKeystoreKeyTypeHint, String orgApacheFelixHttpsKeystoreKeyPassword, String orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, String orgApacheFelixHttpsTruststore, String orgApacheFelixHttpsTruststoreTypeHint, String orgApacheFelixHttpsTruststorePassword, String orgApacheFelixHttpsTruststorePasswordTypeHint, String orgApacheFelixHttpsClientcertificate, String orgApacheFelixHttpsClientcertificateTypeHint, Boolean orgApacheFelixHttpsEnable, String orgApacheFelixHttpsEnableTypeHint, String orgOsgiServiceHttpPortSecure, String orgOsgiServiceHttpPortSecureTypeHint) throws ApiException {
postConfigApacheFelixJettyBasedHttpServiceWithHttpInfo(runmode, orgApacheFelixHttpsNio, orgApacheFelixHttpsNioTypeHint, orgApacheFelixHttpsKeystore, orgApacheFelixHttpsKeystoreTypeHint, orgApacheFelixHttpsKeystorePassword, orgApacheFelixHttpsKeystorePasswordTypeHint, orgApacheFelixHttpsKeystoreKey, orgApacheFelixHttpsKeystoreKeyTypeHint, orgApacheFelixHttpsKeystoreKeyPassword, orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, orgApacheFelixHttpsTruststore, orgApacheFelixHttpsTruststoreTypeHint, orgApacheFelixHttpsTruststorePassword, orgApacheFelixHttpsTruststorePasswordTypeHint, orgApacheFelixHttpsClientcertificate, orgApacheFelixHttpsClientcertificateTypeHint, orgApacheFelixHttpsEnable, orgApacheFelixHttpsEnableTypeHint, orgOsgiServiceHttpPortSecure, orgOsgiServiceHttpPortSecureTypeHint);
}
/**
*
*
* @param runmode (required)
* @param orgApacheFelixHttpsNio (optional)
* @param orgApacheFelixHttpsNioTypeHint (optional)
* @param orgApacheFelixHttpsKeystore (optional)
* @param orgApacheFelixHttpsKeystoreTypeHint (optional)
* @param orgApacheFelixHttpsKeystorePassword (optional)
* @param orgApacheFelixHttpsKeystorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKey (optional)
* @param orgApacheFelixHttpsKeystoreKeyTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKeyPassword (optional)
* @param orgApacheFelixHttpsKeystoreKeyPasswordTypeHint (optional)
* @param orgApacheFelixHttpsTruststore (optional)
* @param orgApacheFelixHttpsTruststoreTypeHint (optional)
* @param orgApacheFelixHttpsTruststorePassword (optional)
* @param orgApacheFelixHttpsTruststorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsClientcertificate (optional)
* @param orgApacheFelixHttpsClientcertificateTypeHint (optional)
* @param orgApacheFelixHttpsEnable (optional)
* @param orgApacheFelixHttpsEnableTypeHint (optional)
* @param orgOsgiServiceHttpPortSecure (optional)
* @param orgOsgiServiceHttpPortSecureTypeHint (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postConfigApacheFelixJettyBasedHttpServiceWithHttpInfo(String runmode, Boolean orgApacheFelixHttpsNio, String orgApacheFelixHttpsNioTypeHint, String orgApacheFelixHttpsKeystore, String orgApacheFelixHttpsKeystoreTypeHint, String orgApacheFelixHttpsKeystorePassword, String orgApacheFelixHttpsKeystorePasswordTypeHint, String orgApacheFelixHttpsKeystoreKey, String orgApacheFelixHttpsKeystoreKeyTypeHint, String orgApacheFelixHttpsKeystoreKeyPassword, String orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, String orgApacheFelixHttpsTruststore, String orgApacheFelixHttpsTruststoreTypeHint, String orgApacheFelixHttpsTruststorePassword, String orgApacheFelixHttpsTruststorePasswordTypeHint, String orgApacheFelixHttpsClientcertificate, String orgApacheFelixHttpsClientcertificateTypeHint, Boolean orgApacheFelixHttpsEnable, String orgApacheFelixHttpsEnableTypeHint, String orgOsgiServiceHttpPortSecure, String orgOsgiServiceHttpPortSecureTypeHint) throws ApiException {
com.squareup.okhttp.Call call = postConfigApacheFelixJettyBasedHttpServiceValidateBeforeCall(runmode, orgApacheFelixHttpsNio, orgApacheFelixHttpsNioTypeHint, orgApacheFelixHttpsKeystore, orgApacheFelixHttpsKeystoreTypeHint, orgApacheFelixHttpsKeystorePassword, orgApacheFelixHttpsKeystorePasswordTypeHint, orgApacheFelixHttpsKeystoreKey, orgApacheFelixHttpsKeystoreKeyTypeHint, orgApacheFelixHttpsKeystoreKeyPassword, orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, orgApacheFelixHttpsTruststore, orgApacheFelixHttpsTruststoreTypeHint, orgApacheFelixHttpsTruststorePassword, orgApacheFelixHttpsTruststorePasswordTypeHint, orgApacheFelixHttpsClientcertificate, orgApacheFelixHttpsClientcertificateTypeHint, orgApacheFelixHttpsEnable, orgApacheFelixHttpsEnableTypeHint, orgOsgiServiceHttpPortSecure, orgOsgiServiceHttpPortSecureTypeHint, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param orgApacheFelixHttpsNio (optional)
* @param orgApacheFelixHttpsNioTypeHint (optional)
* @param orgApacheFelixHttpsKeystore (optional)
* @param orgApacheFelixHttpsKeystoreTypeHint (optional)
* @param orgApacheFelixHttpsKeystorePassword (optional)
* @param orgApacheFelixHttpsKeystorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKey (optional)
* @param orgApacheFelixHttpsKeystoreKeyTypeHint (optional)
* @param orgApacheFelixHttpsKeystoreKeyPassword (optional)
* @param orgApacheFelixHttpsKeystoreKeyPasswordTypeHint (optional)
* @param orgApacheFelixHttpsTruststore (optional)
* @param orgApacheFelixHttpsTruststoreTypeHint (optional)
* @param orgApacheFelixHttpsTruststorePassword (optional)
* @param orgApacheFelixHttpsTruststorePasswordTypeHint (optional)
* @param orgApacheFelixHttpsClientcertificate (optional)
* @param orgApacheFelixHttpsClientcertificateTypeHint (optional)
* @param orgApacheFelixHttpsEnable (optional)
* @param orgApacheFelixHttpsEnableTypeHint (optional)
* @param orgOsgiServiceHttpPortSecure (optional)
* @param orgOsgiServiceHttpPortSecureTypeHint (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postConfigApacheFelixJettyBasedHttpServiceAsync(String runmode, Boolean orgApacheFelixHttpsNio, String orgApacheFelixHttpsNioTypeHint, String orgApacheFelixHttpsKeystore, String orgApacheFelixHttpsKeystoreTypeHint, String orgApacheFelixHttpsKeystorePassword, String orgApacheFelixHttpsKeystorePasswordTypeHint, String orgApacheFelixHttpsKeystoreKey, String orgApacheFelixHttpsKeystoreKeyTypeHint, String orgApacheFelixHttpsKeystoreKeyPassword, String orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, String orgApacheFelixHttpsTruststore, String orgApacheFelixHttpsTruststoreTypeHint, String orgApacheFelixHttpsTruststorePassword, String orgApacheFelixHttpsTruststorePasswordTypeHint, String orgApacheFelixHttpsClientcertificate, String orgApacheFelixHttpsClientcertificateTypeHint, Boolean orgApacheFelixHttpsEnable, String orgApacheFelixHttpsEnableTypeHint, String orgOsgiServiceHttpPortSecure, String orgOsgiServiceHttpPortSecureTypeHint, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postConfigApacheFelixJettyBasedHttpServiceValidateBeforeCall(runmode, orgApacheFelixHttpsNio, orgApacheFelixHttpsNioTypeHint, orgApacheFelixHttpsKeystore, orgApacheFelixHttpsKeystoreTypeHint, orgApacheFelixHttpsKeystorePassword, orgApacheFelixHttpsKeystorePasswordTypeHint, orgApacheFelixHttpsKeystoreKey, orgApacheFelixHttpsKeystoreKeyTypeHint, orgApacheFelixHttpsKeystoreKeyPassword, orgApacheFelixHttpsKeystoreKeyPasswordTypeHint, orgApacheFelixHttpsTruststore, orgApacheFelixHttpsTruststoreTypeHint, orgApacheFelixHttpsTruststorePassword, orgApacheFelixHttpsTruststorePasswordTypeHint, orgApacheFelixHttpsClientcertificate, orgApacheFelixHttpsClientcertificateTypeHint, orgApacheFelixHttpsEnable, orgApacheFelixHttpsEnableTypeHint, orgOsgiServiceHttpPortSecure, orgOsgiServiceHttpPortSecureTypeHint, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postConfigApacheSlingDavExServlet
* @param runmode (required)
* @param alias (optional)
* @param aliasTypeHint (optional)
* @param davCreateAbsoluteUri (optional)
* @param davCreateAbsoluteUriTypeHint (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingDavExServletCall(String runmode, String alias, String aliasTypeHint, Boolean davCreateAbsoluteUri, String davCreateAbsoluteUriTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/apps/system/config/org.apache.sling.jcr.davex.impl.servlets.SlingDavExServlet"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (alias != null)
localVarQueryParams.addAll(apiClient.parameterToPair("alias", alias));
if (aliasTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("alias@TypeHint", aliasTypeHint));
if (davCreateAbsoluteUri != null)
localVarQueryParams.addAll(apiClient.parameterToPair("dav.create-absolute-uri", davCreateAbsoluteUri));
if (davCreateAbsoluteUriTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("dav.create-absolute-uri@TypeHint", davCreateAbsoluteUriTypeHint));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postConfigApacheSlingDavExServletValidateBeforeCall(String runmode, String alias, String aliasTypeHint, Boolean davCreateAbsoluteUri, String davCreateAbsoluteUriTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling postConfigApacheSlingDavExServlet(Async)");
}
com.squareup.okhttp.Call call = postConfigApacheSlingDavExServletCall(runmode, alias, aliasTypeHint, davCreateAbsoluteUri, davCreateAbsoluteUriTypeHint, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param alias (optional)
* @param aliasTypeHint (optional)
* @param davCreateAbsoluteUri (optional)
* @param davCreateAbsoluteUriTypeHint (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postConfigApacheSlingDavExServlet(String runmode, String alias, String aliasTypeHint, Boolean davCreateAbsoluteUri, String davCreateAbsoluteUriTypeHint) throws ApiException {
postConfigApacheSlingDavExServletWithHttpInfo(runmode, alias, aliasTypeHint, davCreateAbsoluteUri, davCreateAbsoluteUriTypeHint);
}
/**
*
*
* @param runmode (required)
* @param alias (optional)
* @param aliasTypeHint (optional)
* @param davCreateAbsoluteUri (optional)
* @param davCreateAbsoluteUriTypeHint (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postConfigApacheSlingDavExServletWithHttpInfo(String runmode, String alias, String aliasTypeHint, Boolean davCreateAbsoluteUri, String davCreateAbsoluteUriTypeHint) throws ApiException {
com.squareup.okhttp.Call call = postConfigApacheSlingDavExServletValidateBeforeCall(runmode, alias, aliasTypeHint, davCreateAbsoluteUri, davCreateAbsoluteUriTypeHint, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param alias (optional)
* @param aliasTypeHint (optional)
* @param davCreateAbsoluteUri (optional)
* @param davCreateAbsoluteUriTypeHint (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingDavExServletAsync(String runmode, String alias, String aliasTypeHint, Boolean davCreateAbsoluteUri, String davCreateAbsoluteUriTypeHint, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postConfigApacheSlingDavExServletValidateBeforeCall(runmode, alias, aliasTypeHint, davCreateAbsoluteUri, davCreateAbsoluteUriTypeHint, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postConfigApacheSlingGetServlet
* @param runmode (required)
* @param jsonMaximumresults (optional)
* @param jsonMaximumresultsTypeHint (optional)
* @param enableHtml (optional)
* @param enableHtmlTypeHint (optional)
* @param enableTxt (optional)
* @param enableTxtTypeHint (optional)
* @param enableXml (optional)
* @param enableXmlTypeHint (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingGetServletCall(String runmode, String jsonMaximumresults, String jsonMaximumresultsTypeHint, Boolean enableHtml, String enableHtmlTypeHint, Boolean enableTxt, String enableTxtTypeHint, Boolean enableXml, String enableXmlTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/apps/system/config/org.apache.sling.servlets.get.DefaultGetServlet"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (jsonMaximumresults != null)
localVarQueryParams.addAll(apiClient.parameterToPair("json.maximumresults", jsonMaximumresults));
if (jsonMaximumresultsTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("json.maximumresults@TypeHint", jsonMaximumresultsTypeHint));
if (enableHtml != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.html", enableHtml));
if (enableHtmlTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.html@TypeHint", enableHtmlTypeHint));
if (enableTxt != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.txt", enableTxt));
if (enableTxtTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.txt@TypeHint", enableTxtTypeHint));
if (enableXml != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.xml", enableXml));
if (enableXmlTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("enable.xml@TypeHint", enableXmlTypeHint));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postConfigApacheSlingGetServletValidateBeforeCall(String runmode, String jsonMaximumresults, String jsonMaximumresultsTypeHint, Boolean enableHtml, String enableHtmlTypeHint, Boolean enableTxt, String enableTxtTypeHint, Boolean enableXml, String enableXmlTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling postConfigApacheSlingGetServlet(Async)");
}
com.squareup.okhttp.Call call = postConfigApacheSlingGetServletCall(runmode, jsonMaximumresults, jsonMaximumresultsTypeHint, enableHtml, enableHtmlTypeHint, enableTxt, enableTxtTypeHint, enableXml, enableXmlTypeHint, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param jsonMaximumresults (optional)
* @param jsonMaximumresultsTypeHint (optional)
* @param enableHtml (optional)
* @param enableHtmlTypeHint (optional)
* @param enableTxt (optional)
* @param enableTxtTypeHint (optional)
* @param enableXml (optional)
* @param enableXmlTypeHint (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postConfigApacheSlingGetServlet(String runmode, String jsonMaximumresults, String jsonMaximumresultsTypeHint, Boolean enableHtml, String enableHtmlTypeHint, Boolean enableTxt, String enableTxtTypeHint, Boolean enableXml, String enableXmlTypeHint) throws ApiException {
postConfigApacheSlingGetServletWithHttpInfo(runmode, jsonMaximumresults, jsonMaximumresultsTypeHint, enableHtml, enableHtmlTypeHint, enableTxt, enableTxtTypeHint, enableXml, enableXmlTypeHint);
}
/**
*
*
* @param runmode (required)
* @param jsonMaximumresults (optional)
* @param jsonMaximumresultsTypeHint (optional)
* @param enableHtml (optional)
* @param enableHtmlTypeHint (optional)
* @param enableTxt (optional)
* @param enableTxtTypeHint (optional)
* @param enableXml (optional)
* @param enableXmlTypeHint (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postConfigApacheSlingGetServletWithHttpInfo(String runmode, String jsonMaximumresults, String jsonMaximumresultsTypeHint, Boolean enableHtml, String enableHtmlTypeHint, Boolean enableTxt, String enableTxtTypeHint, Boolean enableXml, String enableXmlTypeHint) throws ApiException {
com.squareup.okhttp.Call call = postConfigApacheSlingGetServletValidateBeforeCall(runmode, jsonMaximumresults, jsonMaximumresultsTypeHint, enableHtml, enableHtmlTypeHint, enableTxt, enableTxtTypeHint, enableXml, enableXmlTypeHint, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param jsonMaximumresults (optional)
* @param jsonMaximumresultsTypeHint (optional)
* @param enableHtml (optional)
* @param enableHtmlTypeHint (optional)
* @param enableTxt (optional)
* @param enableTxtTypeHint (optional)
* @param enableXml (optional)
* @param enableXmlTypeHint (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingGetServletAsync(String runmode, String jsonMaximumresults, String jsonMaximumresultsTypeHint, Boolean enableHtml, String enableHtmlTypeHint, Boolean enableTxt, String enableTxtTypeHint, Boolean enableXml, String enableXmlTypeHint, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postConfigApacheSlingGetServletValidateBeforeCall(runmode, jsonMaximumresults, jsonMaximumresultsTypeHint, enableHtml, enableHtmlTypeHint, enableTxt, enableTxtTypeHint, enableXml, enableXmlTypeHint, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postConfigApacheSlingReferrerFilter
* @param runmode (required)
* @param allowEmpty (optional)
* @param allowEmptyTypeHint (optional)
* @param allowHosts (optional)
* @param allowHostsTypeHint (optional)
* @param allowHostsRegexp (optional)
* @param allowHostsRegexpTypeHint (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingReferrerFilterCall(String runmode, Boolean allowEmpty, String allowEmptyTypeHint, String allowHosts, String allowHostsTypeHint, String allowHostsRegexp, String allowHostsRegexpTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/apps/system/config/org.apache.sling.security.impl.ReferrerFilter"
.replaceAll("\\{" + "runmode" + "\\}", apiClient.escapeString(runmode.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (allowEmpty != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.empty", allowEmpty));
if (allowEmptyTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.empty@TypeHint", allowEmptyTypeHint));
if (allowHosts != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.hosts", allowHosts));
if (allowHostsTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.hosts@TypeHint", allowHostsTypeHint));
if (allowHostsRegexp != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.hosts.regexp", allowHostsRegexp));
if (allowHostsRegexpTypeHint != null)
localVarQueryParams.addAll(apiClient.parameterToPair("allow.hosts.regexp@TypeHint", allowHostsRegexpTypeHint));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postConfigApacheSlingReferrerFilterValidateBeforeCall(String runmode, Boolean allowEmpty, String allowEmptyTypeHint, String allowHosts, String allowHostsTypeHint, String allowHostsRegexp, String allowHostsRegexpTypeHint, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'runmode' is set
if (runmode == null) {
throw new ApiException("Missing the required parameter 'runmode' when calling postConfigApacheSlingReferrerFilter(Async)");
}
com.squareup.okhttp.Call call = postConfigApacheSlingReferrerFilterCall(runmode, allowEmpty, allowEmptyTypeHint, allowHosts, allowHostsTypeHint, allowHostsRegexp, allowHostsRegexpTypeHint, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param runmode (required)
* @param allowEmpty (optional)
* @param allowEmptyTypeHint (optional)
* @param allowHosts (optional)
* @param allowHostsTypeHint (optional)
* @param allowHostsRegexp (optional)
* @param allowHostsRegexpTypeHint (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postConfigApacheSlingReferrerFilter(String runmode, Boolean allowEmpty, String allowEmptyTypeHint, String allowHosts, String allowHostsTypeHint, String allowHostsRegexp, String allowHostsRegexpTypeHint) throws ApiException {
postConfigApacheSlingReferrerFilterWithHttpInfo(runmode, allowEmpty, allowEmptyTypeHint, allowHosts, allowHostsTypeHint, allowHostsRegexp, allowHostsRegexpTypeHint);
}
/**
*
*
* @param runmode (required)
* @param allowEmpty (optional)
* @param allowEmptyTypeHint (optional)
* @param allowHosts (optional)
* @param allowHostsTypeHint (optional)
* @param allowHostsRegexp (optional)
* @param allowHostsRegexpTypeHint (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postConfigApacheSlingReferrerFilterWithHttpInfo(String runmode, Boolean allowEmpty, String allowEmptyTypeHint, String allowHosts, String allowHostsTypeHint, String allowHostsRegexp, String allowHostsRegexpTypeHint) throws ApiException {
com.squareup.okhttp.Call call = postConfigApacheSlingReferrerFilterValidateBeforeCall(runmode, allowEmpty, allowEmptyTypeHint, allowHosts, allowHostsTypeHint, allowHostsRegexp, allowHostsRegexpTypeHint, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param runmode (required)
* @param allowEmpty (optional)
* @param allowEmptyTypeHint (optional)
* @param allowHosts (optional)
* @param allowHostsTypeHint (optional)
* @param allowHostsRegexp (optional)
* @param allowHostsRegexpTypeHint (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postConfigApacheSlingReferrerFilterAsync(String runmode, Boolean allowEmpty, String allowEmptyTypeHint, String allowHosts, String allowHostsTypeHint, String allowHostsRegexp, String allowHostsRegexpTypeHint, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postConfigApacheSlingReferrerFilterValidateBeforeCall(runmode, allowEmpty, allowEmptyTypeHint, allowHosts, allowHostsTypeHint, allowHostsRegexp, allowHostsRegexpTypeHint, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postNodeRw
* @param path (required)
* @param name (required)
* @param addMembers (optional)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postNodeRwCall(String path, String name, String addMembers, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{path}/{name}.rw.html"
.replaceAll("\\{" + "path" + "\\}", apiClient.escapeString(path.toString()))
.replaceAll("\\{" + "name" + "\\}", apiClient.escapeString(name.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (addMembers != null)
localVarQueryParams.addAll(apiClient.parameterToPair("addMembers", addMembers));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postNodeRwValidateBeforeCall(String path, String name, String addMembers, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling postNodeRw(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling postNodeRw(Async)");
}
com.squareup.okhttp.Call call = postNodeRwCall(path, name, addMembers, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param name (required)
* @param addMembers (optional)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postNodeRw(String path, String name, String addMembers) throws ApiException {
postNodeRwWithHttpInfo(path, name, addMembers);
}
/**
*
*
* @param path (required)
* @param name (required)
* @param addMembers (optional)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postNodeRwWithHttpInfo(String path, String name, String addMembers) throws ApiException {
com.squareup.okhttp.Call call = postNodeRwValidateBeforeCall(path, name, addMembers, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param path (required)
* @param name (required)
* @param addMembers (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postNodeRwAsync(String path, String name, String addMembers, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postNodeRwValidateBeforeCall(path, name, addMembers, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postPath
* @param path (required)
* @param jcrprimaryType (required)
* @param name (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postPathCall(String path, String jcrprimaryType, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/{path}/"
.replaceAll("\\{" + "path" + "\\}", apiClient.escapeString(path.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (jcrprimaryType != null)
localVarQueryParams.addAll(apiClient.parameterToPair("jcr:primaryType", jcrprimaryType));
if (name != null)
localVarQueryParams.addAll(apiClient.parameterToPair(":name", name));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postPathValidateBeforeCall(String path, String jcrprimaryType, String name, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling postPath(Async)");
}
// verify the required parameter 'jcrprimaryType' is set
if (jcrprimaryType == null) {
throw new ApiException("Missing the required parameter 'jcrprimaryType' when calling postPath(Async)");
}
// verify the required parameter 'name' is set
if (name == null) {
throw new ApiException("Missing the required parameter 'name' when calling postPath(Async)");
}
com.squareup.okhttp.Call call = postPathCall(path, jcrprimaryType, name, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param jcrprimaryType (required)
* @param name (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postPath(String path, String jcrprimaryType, String name) throws ApiException {
postPathWithHttpInfo(path, jcrprimaryType, name);
}
/**
*
*
* @param path (required)
* @param jcrprimaryType (required)
* @param name (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postPathWithHttpInfo(String path, String jcrprimaryType, String name) throws ApiException {
com.squareup.okhttp.Call call = postPathValidateBeforeCall(path, jcrprimaryType, name, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param path (required)
* @param jcrprimaryType (required)
* @param name (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postPathAsync(String path, String jcrprimaryType, String name, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postPathValidateBeforeCall(path, jcrprimaryType, name, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/**
* Build call for postQuery
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postQueryCall(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/bin/querybuilder.json";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (path != null)
localVarQueryParams.addAll(apiClient.parameterToPair("path", path));
if (pLimit != null)
localVarQueryParams.addAll(apiClient.parameterToPair("p.limit", pLimit));
if (_1Property != null)
localVarQueryParams.addAll(apiClient.parameterToPair("1_property", _1Property));
if (_1PropertyValue != null)
localVarQueryParams.addAll(apiClient.parameterToPair("1_property.value", _1PropertyValue));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postQueryValidateBeforeCall(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling postQuery(Async)");
}
// verify the required parameter 'pLimit' is set
if (pLimit == null) {
throw new ApiException("Missing the required parameter 'pLimit' when calling postQuery(Async)");
}
// verify the required parameter '_1Property' is set
if (_1Property == null) {
throw new ApiException("Missing the required parameter '_1Property' when calling postQuery(Async)");
}
// verify the required parameter '_1PropertyValue' is set
if (_1PropertyValue == null) {
throw new ApiException("Missing the required parameter '_1PropertyValue' when calling postQuery(Async)");
}
com.squareup.okhttp.Call call = postQueryCall(path, pLimit, _1Property, _1PropertyValue, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @return String
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public String postQuery(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue) throws ApiException {
ApiResponse resp = postQueryWithHttpInfo(path, pLimit, _1Property, _1PropertyValue);
return resp.getData();
}
/**
*
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @return ApiResponse<String>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postQueryWithHttpInfo(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue) throws ApiException {
com.squareup.okhttp.Call call = postQueryValidateBeforeCall(path, pLimit, _1Property, _1PropertyValue, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param path (required)
* @param pLimit (required)
* @param _1Property (required)
* @param _1PropertyValue (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postQueryAsync(String path, BigDecimal pLimit, String _1Property, String _1PropertyValue, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postQueryValidateBeforeCall(path, pLimit, _1Property, _1PropertyValue, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/**
* Build call for postTreeActivation
* @param ignoredeactivated (required)
* @param onlymodified (required)
* @param path (required)
* @param progressListener Progress listener
* @param progressRequestListener Progress request listener
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public com.squareup.okhttp.Call postTreeActivationCall(Boolean ignoredeactivated, Boolean onlymodified, String path, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/etc/replication/treeactivation.html";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
if (ignoredeactivated != null)
localVarQueryParams.addAll(apiClient.parameterToPair("ignoredeactivated", ignoredeactivated));
if (onlymodified != null)
localVarQueryParams.addAll(apiClient.parameterToPair("onlymodified", onlymodified));
if (path != null)
localVarQueryParams.addAll(apiClient.parameterToPair("path", path));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"text/plain"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { "aemAuth" };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
@SuppressWarnings("rawtypes")
private com.squareup.okhttp.Call postTreeActivationValidateBeforeCall(Boolean ignoredeactivated, Boolean onlymodified, String path, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
// verify the required parameter 'ignoredeactivated' is set
if (ignoredeactivated == null) {
throw new ApiException("Missing the required parameter 'ignoredeactivated' when calling postTreeActivation(Async)");
}
// verify the required parameter 'onlymodified' is set
if (onlymodified == null) {
throw new ApiException("Missing the required parameter 'onlymodified' when calling postTreeActivation(Async)");
}
// verify the required parameter 'path' is set
if (path == null) {
throw new ApiException("Missing the required parameter 'path' when calling postTreeActivation(Async)");
}
com.squareup.okhttp.Call call = postTreeActivationCall(ignoredeactivated, onlymodified, path, progressListener, progressRequestListener);
return call;
}
/**
*
*
* @param ignoredeactivated (required)
* @param onlymodified (required)
* @param path (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void postTreeActivation(Boolean ignoredeactivated, Boolean onlymodified, String path) throws ApiException {
postTreeActivationWithHttpInfo(ignoredeactivated, onlymodified, path);
}
/**
*
*
* @param ignoredeactivated (required)
* @param onlymodified (required)
* @param path (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse postTreeActivationWithHttpInfo(Boolean ignoredeactivated, Boolean onlymodified, String path) throws ApiException {
com.squareup.okhttp.Call call = postTreeActivationValidateBeforeCall(ignoredeactivated, onlymodified, path, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param ignoredeactivated (required)
* @param onlymodified (required)
* @param path (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call postTreeActivationAsync(Boolean ignoredeactivated, Boolean onlymodified, String path, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = postTreeActivationValidateBeforeCall(ignoredeactivated, onlymodified, path, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy