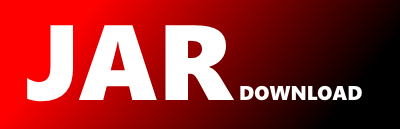
shz.core.ArrayHelp Maven / Gradle / Ivy
package shz.core;
import shz.core.constant.ArrayConstant;
import shz.core.hash.MdHash;
import java.util.function.Predicate;
public final class ArrayHelp {
private ArrayHelp() {
throw new IllegalStateException();
}
private static final char[] ZERO = {'0'};
private static final char[] MIN_VALUE = {'1', '0'};
public static char[] toBinaryChars(int i) {
if (i == 0) return ZERO;
boolean lt0 = i < 0;
if (lt0) {
if (i == Integer.MIN_VALUE) return MIN_VALUE;
else i = -i;
}
int len = Math.max(Integer.SIZE - Integer.numberOfLeadingZeros(i), 1);
char[] chars = new char[len + 1];
chars[0] = lt0 ? '1' : '0';
do {
chars[len--] = ArrayConstant.CHAR_DIGIT_LOWERCASE[i & 1];
i >>>= 1;
} while (i != 0 && len > 0);
return chars;
}
public static char[] toBinaryChars(long j) {
if (j == 0) return ZERO;
boolean lt0 = j < 0L;
if (lt0) {
if (j == Long.MIN_VALUE) return MIN_VALUE;
else j = -j;
}
int len = Math.max(Long.SIZE - Long.numberOfLeadingZeros(j), 1);
char[] chars = new char[len + 1];
chars[0] = lt0 ? '1' : '0';
do {
chars[len--] = ArrayConstant.CHAR_DIGIT_LOWERCASE[((int) j) & 1];
j >>>= 1;
} while (j != 0 && len > 0);
return chars;
}
public static int binaryCharsToInt(char[] a) {
int len;
if ((len = NullHelp.length(a)) == 0) throw new IllegalStateException();
else if (len == 1) {
if (a[0] != '0') throw new IllegalArgumentException();
return 0;
} else if (len == 2 && a[1] == '0') {
if (a[0] != '1') throw new IllegalArgumentException();
return Integer.MIN_VALUE;
} else if (len > Integer.SIZE) throw new IllegalStateException();
int num = 0;
for (int i = 1; i < len; ++i) if (a[i] == '1') num += 1 << len - 1 - i;
return a[0] == '1' ? -num : num;
}
public static long binaryCharsToLong(char[] a) {
int len;
if ((len = NullHelp.length(a)) == 0) throw new IllegalStateException();
else if (len == 1) {
if (a[0] != '0') throw new IllegalArgumentException();
return 0L;
} else if (len == 2 && a[1] == '0') {
if (a[0] != '1') throw new IllegalArgumentException();
return Long.MIN_VALUE;
} else if (len > Long.SIZE) throw new IllegalStateException();
long num = 0;
for (int i = 1; i < len; ++i) if (a[i] == '1') num += 1L << len - 1 - i;
return a[0] == '1' ? -num : num;
}
public static long toLong(byte[] bytes) {
if (NullHelp.isEmpty(bytes)) return 0L;
bytes = MdHash.SHA1.hash(bytes);
long result = 0L;
for (int i = bytes.length - 1; i >= bytes.length - Long.BYTES; --i) {
result <<= Long.BYTES;
result |= bytes[i] & 0xff;
}
return result;
}
public static long toUnsignedLong(byte[] bytes) {
if (NullHelp.isEmpty(bytes)) return 0L;
bytes = MdHash.SHA1.hash(bytes);
long result = 0L;
for (int i = bytes.length - 1; i > bytes.length - Long.BYTES; --i) {
result <<= Long.BYTES;
result |= bytes[i] & 0xff;
}
result <<= Long.BYTES - 1;
result |= bytes[bytes.length - Long.BYTES] & 0x7f;
return result;
}
public static int idx(T[] array, Predicate filter) {
for (int i = 0; i < array.length; ++i) if (filter.test(array[i])) return i;
return -1;
}
public static boolean contains(T[] array, Predicate filter) {
return idx(array, filter) != -1;
}
@SafeVarargs
public static T[] of(T... array) {
return array;
}
public static byte[][] split(byte[] bytes, int blockSize) {
if (NullHelp.isEmpty(bytes)) return new byte[][]{};
if (bytes.length <= blockSize) return new byte[][]{bytes};
int len = (bytes.length - 1) / blockSize + 1;
int minLen = bytes.length / blockSize;
byte[][] result = new byte[len][];
for (int i = 0; i < minLen; ++i) {
byte[] block = new byte[blockSize];
System.arraycopy(bytes, blockSize * i, block, 0, blockSize);
result[i] = block;
}
if (minLen < len) {
byte[] block = new byte[bytes.length - blockSize * minLen];
System.arraycopy(bytes, blockSize * minLen, block, 0, block.length);
result[minLen] = block;
}
return result;
}
public static void reverse(boolean[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(boolean[] a, int i, int j) {
boolean t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(byte[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(byte[] a, int i, int j) {
byte t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(char[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(char[] a, int i, int j) {
char t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(short[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(short[] a, int i, int j) {
short t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(int[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(int[] a, int i, int j) {
int t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(long[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(long[] a, int i, int j) {
long t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(float[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(float[] a, int i, int j) {
float t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(double[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(double[] a, int i, int j) {
double t = a[i];
a[i] = a[j];
a[j] = t;
}
public static void reverse(T[] a, int start, int len) {
if (start < 0 || len <= 1 || NullHelp.isEmpty(a)) return;
NullHelp.requireNon(start + len > a.length);
for (int i = start, j = start + len - 1; i < j; ++i, --j) swap(a, i, j);
}
public static void swap(T[] a, int i, int j) {
T t = a[i];
a[i] = a[j];
a[j] = t;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy