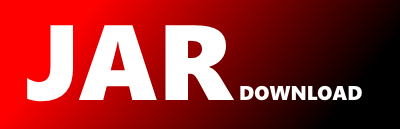
shz.core.io.ZipHelp Maven / Gradle / Ivy
package shz.core.io;
import shz.core.NullHelp;
import shz.core.PRException;
import shz.core.type.TypeHelp;
import java.io.*;
import java.net.JarURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.nio.MappedByteBuffer;
import java.nio.channels.FileChannel;
import java.nio.charset.Charset;
import java.nio.file.Path;
import java.nio.file.StandardOpenOption;
import java.util.*;
import java.util.function.*;
import java.util.jar.*;
import java.util.zip.*;
import static shz.core.io.IOHelp.DEFAULT_DATA_SIZE;
public final class ZipHelp {
private ZipHelp() {
throw new IllegalStateException();
}
public static void compress(Set srcs, File des, Function streamFunc, Consumer entrySetter) {
try (ZipOutputStream zos = streamFunc == null ? IOHelp.newZipOutputStream(des.toPath()) : streamFunc.apply(des)) {
srcs.stream().filter(Objects::nonNull).filter(f -> f.exists() && f.canRead()).forEach(f -> compress(zos, entrySetter, "", f));
} catch (IOException e) {
throw PRException.of(e);
}
}
public static void compress(ZipOutputStream zos, Consumer entrySetter, String entryName, File... files) {
Arrays.stream(files).forEach(f -> {
if (f.isFile()) {
BufferedInputStream bis = IOHelp.newBufferedInputStream(f.toPath(), StandardOpenOption.READ);
try {
ZipEntry entry = new ZipEntry(entryName + f.getName());
if (entrySetter != null) entrySetter.accept(entry);
zos.putNextEntry(entry);
} catch (IOException e) {
throw PRException.of(e);
}
IOHelp.read(bis, zos, DEFAULT_DATA_SIZE, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
} else {
File[] fs = f.listFiles();
if (NullHelp.isEmpty(fs)) try {
zos.putNextEntry(new ZipEntry(entryName + f.getName() + "/"));
} catch (IOException e) {
throw PRException.of(e);
}
else compress(zos, entrySetter, entryName + "/" + f.getName(), fs);
}
});
}
public static void compress(Set srcs, File des) {
compress(srcs, des, null, null);
}
public static void decompress(File src, File des, Consumer entrySetter) {
if (src == null) return;
FileHelp.checkCopyDir(des);
ZipFile zip;
try {
zip = new ZipFile(src);
} catch (IOException e) {
throw PRException.of(e);
}
Enumeration extends ZipEntry> entries = zip.entries();
InputStream is = null;
try {
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
if (entry.isDirectory()) continue;
File f = new File(des, entry.getName());
FileHelp.checkWriteFile(f);
if (entrySetter != null) entrySetter.accept(entry);
is = zip.getInputStream(entry);
IOHelp.read(is, IOHelp.newBufferedOutputStream(f.toPath()), DEFAULT_DATA_SIZE, null, (i, o) -> IOHelp.close(o));
}
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(is);
}
}
public static void decompress(File src, File des) {
decompress(src, des, null);
}
public static InputStream getIsFromZis(ZipInputStream zis, String entryName) {
if (zis == null || NullHelp.isBlank(entryName)) return null;
ZipEntry entry;
try {
while ((entry = zis.getNextEntry()) != null)
if (entry.getName().equals(entryName))
return new ByteArrayInputStream(IOHelp.read(zis, DEFAULT_DATA_SIZE, null));
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static InputStream getIsFromZipFile(ZipFile zip, String entryName) {
if (zip == null || NullHelp.isBlank(entryName)) return null;
Enumeration extends ZipEntry> entries = zip.entries();
InputStream is;
try {
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
if (entry.getName().equals(entryName)) {
is = zip.getInputStream(entry);
return new ByteArrayInputStream(IOHelp.read(is, DEFAULT_DATA_SIZE, null));
}
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static InputStream getIsFromJis(JarInputStream jis, String entryName) {
if (jis == null || NullHelp.isBlank(entryName)) return null;
JarEntry entry;
try {
while ((entry = (JarEntry) jis.getNextEntry()) != null) {
if (entry.getName().equals(entryName))
return new ByteArrayInputStream(IOHelp.read(jis, DEFAULT_DATA_SIZE, null));
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static InputStream getIsFromJarFile(JarFile jar, String entryName) {
if (jar == null || NullHelp.isBlank(entryName)) return null;
Enumeration entries = jar.entries();
InputStream is;
try {
while (entries.hasMoreElements()) {
JarEntry entry = entries.nextElement();
if (entry.getName().equals(entryName)) {
is = jar.getInputStream(entry);
return new ByteArrayInputStream(IOHelp.read(is, DEFAULT_DATA_SIZE, null));
}
}
} catch (IOException e) {
throw PRException.of(e);
}
return null;
}
public static File copyFromJarByClass(Class> cls, String entryName, File dir) {
File file;
if (dir != null) file = new File(dir, entryName);
else file = new File(System.getProperty("user.dir"), entryName);
File parentFile = file.getParentFile();
if (!parentFile.mkdirs() && !parentFile.exists()) return null;
URL url = cls.getResource(entryName);
if (url == null) return null;
try {
URLConnection conn = url.openConnection();
InputStream is;
if (conn instanceof JarURLConnection) {
JarFile jarFile = ((JarURLConnection) conn).getJarFile();
is = getIsFromJarFile(jarFile, entryName);
} else is = conn.getInputStream();
IOHelp.read(is, IOHelp.newBufferedOutputStream(file.toPath()));
} catch (IOException e) {
throw PRException.of(e);
}
return file;
}
public static File copyFromJarByClass(Class> cls, String entryName) {
return copyFromJarByClass(cls, entryName, null);
}
public static File copyFromJarByClass(Class> cls, File dir) {
return copyFromJarByClass(cls, "/" + cls.getName().replace('.', '/') + ".class", null);
}
public static File copyFromJarByClass(Class> cls) {
return copyFromJarByClass(cls, (File) null);
}
public static JarFile getJarFile(URL url) {
if (url == null) return null;
try {
URLConnection conn = url.openConnection();
if (conn instanceof JarURLConnection) return ((JarURLConnection) conn).getJarFile();
return new JarFile(url.getPath());
} catch (IOException e) {
throw PRException.of(e);
}
}
public static JarFile getJarFile(Class> cls) {
return getJarFile(TypeHelp.toUrl(cls));
}
public static void makeJar(File file, JarOutputStream jos) {
File[] files = file.listFiles();
if (NullHelp.nonEmpty(files)) try {
int idx = file.getAbsolutePath().replace("\\\\", "/").lastIndexOf("/") + 1;
for (File f : files) makeJar0(f, jos, idx);
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(jos);
}
}
private static void makeJar0(File file, JarOutputStream jos, int idx) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (NullHelp.nonEmpty(files)) for (File f : files) makeJar0(f, jos, idx);
} else {
BufferedInputStream bis = IOHelp.newBufferedInputStream(file.toPath());
jos.putNextEntry(new JarEntry(file.getAbsolutePath().replace("\\\\", "/").substring(idx)));
IOHelp.read(bis, jos, DEFAULT_DATA_SIZE, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(i);
}
});
}
}
public static void makeRunnableJar(JarInputStream jis, JarOutputStream jos) {
JarEntry entry;
try {
while ((entry = jis.getNextJarEntry()) != null) {
if (JarFile.MANIFEST_NAME.equals(entry.getName())) continue;
jos.putNextEntry(entry);
IOHelp.read(jis, jos, DEFAULT_DATA_SIZE, null, (i, o) -> {
try {
o.closeEntry();
} catch (IOException e) {
throw PRException.of(e);
}
});
}
} catch (IOException e) {
throw PRException.of(e);
} finally {
IOHelp.close(jos, jis);
}
}
public static void makeRunnableJar(JarInputStream jis, File newJar, Manifest manifest) {
makeRunnableJar(jis, IOHelp.newJarOutputStream(newJar.toPath(), manifest));
}
public static void makeRunnableJar(JarInputStream jis, File newJar, String mainClass, String version) {
Manifest manifest = jis.getManifest();
if (manifest == null) manifest = new Manifest();
Attributes a = manifest.getMainAttributes();
String oldMainClass = a.putValue("Main-Class", mainClass);
if (oldMainClass != null) {
IOHelp.close(jis);
return;
}
if (version == null) {
version = (String) a.get("Manifest-Version");
if (version == null) version = (String) a.get("Signature-Version");
}
a.putValue("Manifest-Version", version);
makeRunnableJar(jis, IOHelp.newJarOutputStream(newJar.toPath(), manifest));
}
public static String read(InputStream is, Charset charset) {
try {
return IOHelp.read(new BufferedReader(new InputStreamReader(new GZIPInputStream(new ByteArrayInputStream(IOHelp.read(is))), charset)));
} catch (IOException e) {
throw PRException.of(e);
}
}
/////////////////////////////////////////////CRC32
public static long checkSumInputStream(InputStream is) {
CRC32 crc = new CRC32();
try {
if (is.available() > 0) {
int c;
while ((c = is.read()) != -1) crc.update(c);
}
} catch (IOException e) {
throw PRException.of(e);
}
return crc.getValue();
}
public static long checkSumRandomAccessFile(Path filename) {
CRC32 crc = new CRC32();
try (RandomAccessFile file = new RandomAccessFile(filename.toFile(), "r")) {
long len = file.length();
for (long i = 0; i < len; ++i) {
file.seek(i);
crc.update(file.readByte());
}
} catch (IOException e) {
throw PRException.of(e);
}
return crc.getValue();
}
public static long checkSumMappedFile(Path filename) {
CRC32 crc = new CRC32();
try (FileChannel channel = FileChannel.open(filename)) {
int len = (int) channel.size();
MappedByteBuffer buffer = channel.map(FileChannel.MapMode.READ_ONLY, 0, len);
for (int i = 0; i < len; ++i) crc.update(buffer.get(i));
} catch (IOException e) {
throw PRException.of(e);
}
return crc.getValue();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy