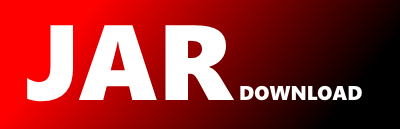
shz.core.cache.LocalCache Maven / Gradle / Ivy
package shz.core.cache;
import java.lang.ref.Reference;
import java.lang.ref.ReferenceQueue;
import java.lang.ref.SoftReference;
import java.lang.ref.WeakReference;
import java.util.Collection;
import java.util.function.Supplier;
abstract class LocalCache implements Cache {
private final RefType refType;
protected final ReferenceQueue queue;
protected LocalCache(RefType refType, ReferenceQueue queue) {
this.refType = refType;
this.queue = queue;
}
protected final Reference getReference(V val) {
return refType == RefType.WEAK ? new WeakReference<>(val, queue) : new SoftReference<>(val, queue);
}
@Override
public final V get(K key, Supplier> supplier) {
return Cache.super.get(key, supplier);
}
@Override
public final void set(K key, V val) {
Cache.super.set(key, val);
}
@Override
public final void delete(Collection keys) {
Cache.super.delete(keys);
}
@Override
public final boolean expireAt(K key, long timestamp) {
return Cache.super.expireAt(key, timestamp);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy