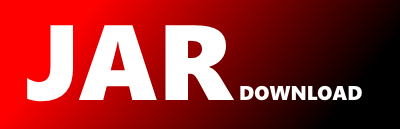
shz.core.cl.CustomClassLoader Maven / Gradle / Ivy
package shz.core.cl;
import shz.core.NullHelp;
import java.security.SecureClassLoader;
import java.util.function.Function;
public class CustomClassLoader extends SecureClassLoader {
protected final Function classByteFunc;
public CustomClassLoader(ClassLoader parent, Function classByteFunc) {
super(parent);
this.classByteFunc = classByteFunc;
}
public CustomClassLoader(Function classByteFunc) {
this.classByteFunc = classByteFunc;
}
public CustomClassLoader(ClassLoader parent) {
this(parent, null);
}
public CustomClassLoader() {
this((Function) null);
}
@Override
public Class> loadClass(String name) throws ClassNotFoundException {
if (NullHelp.nonBlank(name)) return super.loadClass(name, true);
return classByteFunc == null ? null : loadClass(classByteFunc.apply(null));
}
public Class> loadClass(byte[] bytes) {
if (NullHelp.isEmpty(bytes)) return null;
return defineClass(null, bytes, 0, bytes.length);
}
@Override
protected Class> findClass(String name) throws ClassNotFoundException {
byte[] bytes;
if (classByteFunc != null && NullHelp.nonEmpty(bytes = classByteFunc.apply(name)))
return defineClass(name, bytes, 0, bytes.length);
return super.findClass(name);
}
public static CustomClassLoader of(Function classByteFunc) {
return new CustomClassLoader(classByteFunc);
}
public static CustomClassLoader of(Function classByteFunc, Function modifier) {
return new CustomClassLoader(classByteFunc == null ? null : name -> modifier.apply(classByteFunc.apply(name)));
}
@SafeVarargs
public static CustomClassLoader of(Function classByteFunc, Function... modifiers) {
return new CustomClassLoader(classByteFunc == null ? null : name -> {
Function modifier = modifiers[0];
for (int i = 1; i < modifiers.length; ++i) modifier = modifier.andThen(modifiers[i]);
return modifier.apply(classByteFunc.apply(name));
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy