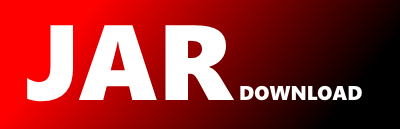
shz.core.hash.MacHash Maven / Gradle / Ivy
package shz.core.hash;
import shz.core.NullHelp;
import shz.core.PRException;
import shz.core.constant.ArrayConstant;
import shz.core.io.IOHelp;
import javax.crypto.Mac;
import javax.crypto.spec.SecretKeySpec;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.net.URL;
import java.nio.file.Path;
import java.security.InvalidKeyException;
import java.security.NoSuchAlgorithmException;
import java.util.function.BiConsumer;
public enum MacHash implements IMacHash {
SHA1("HmacSHA1"),
SHA224("HmacSHA224"),
SHA256("HmacSHA256"),
SHA384("HmacSHA384"),
SHA512("HmacSHA512"),
;
private final String algorithm;
MacHash(String algorithm) {
this.algorithm = algorithm;
}
private Mac mac(byte[] key) {
try {
Mac mac = Mac.getInstance(algorithm);
mac.init(new SecretKeySpec(key, algorithm));
return mac;
} catch (NoSuchAlgorithmException | InvalidKeyException e) {
throw PRException.of(e);
}
}
@Override
public byte[] hash(byte[] bytes, byte[] key) {
if (NullHelp.isEmpty(bytes)) return ArrayConstant.EMPTY_BYTE_ARRAY;
return mac(key).doFinal(bytes);
}
@Override
public byte[] hash(InputStream is, byte[] key) {
if (is == null) return ArrayConstant.EMPTY_BYTE_ARRAY;
Mac mac = mac(key);
IOHelp.read(is, (BiConsumer) (buffer, len) -> mac.update(buffer, 0, len));
return mac.doFinal();
}
@Override
public byte[] hash(URL url, byte[] key) {
if (url == null) return ArrayConstant.EMPTY_BYTE_ARRAY;
try {
return hash(url.openConnection().getInputStream(), key);
} catch (IOException e) {
throw PRException.of(e);
}
}
@Override
public byte[] hash(Path path, byte[] key) {
return hash(IOHelp.newBufferedInputStream(path), key);
}
@Override
public byte[] hash(File file, byte[] key) {
return hash(file.toPath(), key);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy