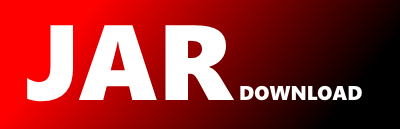
shz.core.structure.StringDigit Maven / Gradle / Ivy
package shz.core.structure;
import shz.core.constant.ArrayConstant;
import shz.core.reference.ZReference;
import shz.core.stack.a.CArrayStack;
import java.io.*;
import java.util.Arrays;
/**
* 原码: 二进制数
* 反码: 正数的反码就是自身,负数的反码除符号位外,其他各位求反
* 补码: 正数的补码还是自身,负数的补码为反码加1
*/
public final class StringDigit implements Serializable {
private static final long serialVersionUID = -2748414764831748398L;
public final char[] chars;
private transient CharIndex charIndex;
private StringDigit(char[] chars) {
this.chars = chars;
init();
}
private void init() {
charIndex = CharIndex.of(chars);
}
public static final StringDigit C2 = new StringDigit(ArrayConstant.CHAR_ARRAY_2);
public static final StringDigit C8 = new StringDigit(ArrayConstant.CHAR_ARRAY_8);
public static final StringDigit C10 = new StringDigit(ArrayConstant.CHAR_ARRAY_10);
public static final StringDigit C16 = new StringDigit(ArrayConstant.CHAR_ARRAY_16);
public static final StringDigit C94 = new StringDigit(ArrayConstant.CHAR_ARRAY_94);
public static final StringDigit UPPERCASE = new StringDigit(ArrayConstant.CHAR_UPPERCASE);
public static final StringDigit LOWERCASE = new StringDigit(ArrayConstant.CHAR_LOWERCASE);
public static final StringDigit SYMBOL = new StringDigit(ArrayConstant.CHAR_SYMBOL);
public static final StringDigit DIGIT_UPPERCASE = new StringDigit(ArrayConstant.CHAR_DIGIT_UPPERCASE);
public static final StringDigit DIGIT_LOWERCASE = new StringDigit(ArrayConstant.CHAR_DIGIT_LOWERCASE);
public static final StringDigit DIGIT_ALPHABET = new StringDigit(ArrayConstant.CHAR_DIGIT_ALPHABET);
public static final StringDigit IP_V4 = new StringDigit(ArrayConstant.CHAR_IP_V4);
public static final StringDigit IP_V6 = new StringDigit(ArrayConstant.CHAR_IP_V6);
public static final StringDigit URL = new StringDigit(ArrayConstant.CHAR_URL);
public static StringDigit of(char[] chars) {
if (chars == null || chars.length == 0) throw new NullPointerException();
if (Arrays.equals(chars, ArrayConstant.CHAR_ARRAY_2)) return C2;
if (Arrays.equals(chars, ArrayConstant.CHAR_ARRAY_8)) return C8;
if (Arrays.equals(chars, ArrayConstant.CHAR_ARRAY_10)) return C10;
if (Arrays.equals(chars, ArrayConstant.CHAR_ARRAY_16)) return C16;
if (Arrays.equals(chars, ArrayConstant.CHAR_ARRAY_94)) return C94;
if (Arrays.equals(chars, ArrayConstant.CHAR_UPPERCASE)) return UPPERCASE;
if (Arrays.equals(chars, ArrayConstant.CHAR_LOWERCASE)) return LOWERCASE;
if (Arrays.equals(chars, ArrayConstant.CHAR_SYMBOL)) return SYMBOL;
if (Arrays.equals(chars, ArrayConstant.CHAR_DIGIT_UPPERCASE)) return DIGIT_UPPERCASE;
if (Arrays.equals(chars, ArrayConstant.CHAR_DIGIT_LOWERCASE)) return DIGIT_LOWERCASE;
if (Arrays.equals(chars, ArrayConstant.CHAR_DIGIT_ALPHABET)) return DIGIT_ALPHABET;
if (Arrays.equals(chars, ArrayConstant.CHAR_IP_V4)) return IP_V4;
if (Arrays.equals(chars, ArrayConstant.CHAR_IP_V6)) return IP_V6;
if (Arrays.equals(chars, ArrayConstant.CHAR_URL)) return URL;
return new StringDigit(chars);
}
public String increment(String s) {
int len;
if (s == null || (len = s.length()) == 0) return s;
int idx = len - 1;
char[] array = s.toCharArray();
int index;
while (true) {
index = charIndex.idx(array[idx]);
if (index != chars.length - 1) {
array[idx] = chars[index + 1];
return new String(array);
}
if (idx == 0) {
array[0] = chars[0];
return chars[1] + new String(array);
}
array[idx] = chars[0];
--idx;
}
}
public String decrement(String s) {
int len;
if (s == null || (len = s.length()) == 0) return s;
int idx = len - 1;
char[] array = s.toCharArray();
int index;
while (true) {
index = charIndex.idx(array[idx]);
if (index != 0) {
array[idx] = chars[index - 1];
return new String(array);
}
if (idx == 0) return new String(array, 1, len - 1);
--idx;
}
}
public int compare(String s1, String s2) {
if (s1 == null || s1.length() == 0) {
if (s2 == null || s2.length() == 0) return 0;
return -1;
}
if (s2 == null || s2.length() == 0) return 1;
int len = s1.length();
if (len - s2.length() != 0) return len - s2.length();
for (int i = 0; i < len; ++i) {
int delta = charIndex.idx(s1.charAt(i)) - charIndex.idx(s2.charAt(i));
if (delta != 0) return delta;
}
return 0;
}
public boolean gt(String s1, String s2) {
return compare(s1, s2) > 0;
}
public boolean ge(String s1, String s2) {
return compare(s1, s2) >= 0;
}
public boolean lt(String s1, String s2) {
return !ge(s1, s2);
}
public boolean le(String s1, String s2) {
return !gt(s1, s2);
}
public String plus(String s1, String s2) {
if (s1 == null || s1.length() == 0) return s2;
if (s2 == null || s2.length() == 0) return s1;
if (s1.length() < s2.length()) {
String temp = s1;
s1 = s2;
s2 = temp;
}
char[] lmax = s1.toCharArray();
char[] lmin = s2.toCharArray();
int i = lmax.length - 1, j = lmin.length - 1;
ZReference carry = new ZReference();
while (j >= 0) {
plus0(lmax, i, lmin[j], carry);
--i;
--j;
}
return carry.get() ? chars[1] + new String(lmax) : new String(lmax);
}
private void plus0(char[] lmax, int i, char c, ZReference carry) {
if (i == -1) {
carry.set(true);
return;
}
int idx = charIndex.idx(lmax[i]) + charIndex.idx(c);
if (idx <= chars.length - 1) lmax[i] = chars[idx];
else {
lmax[i] = chars[idx - chars.length];
plus0(lmax, --i, chars[1], carry);
}
}
public String minus(String s1, String s2) {
if (s1 == null || s1.length() == 0) return s2;
if (s2 == null || s2.length() == 0) return s1;
if (s1.length() < s2.length()) {
String temp = s1;
s1 = s2;
s2 = temp;
} else if (s1.length() == s2.length() && compare(s1, s2) < 0) {
String temp = s1;
s1 = s2;
s2 = temp;
}
char[] lmax = s1.toCharArray();
char[] lmin = s2.toCharArray();
int i = lmax.length - 1, j = lmin.length - 1;
while (j >= 0) {
minus0(lmax, i, lmin[j]);
--i;
--j;
}
int L = 0;
for (i = 0; i < lmax.length; ++i)
if (charIndex.idx(lmax[i]) == 0) ++L;
else break;
if (L == 0) return new String(lmax);
if (L == lmax.length) return chars[0] + "";
return new String(lmax, L, lmax.length - L);
}
private void minus0(char[] lmax, int i, char c) {
int idx = charIndex.idx(lmax[i]) - charIndex.idx(c);
if (idx >= 0) lmax[i] = chars[idx];
else {
lmax[i] = chars[idx + chars.length];
minus0(lmax, --i, chars[1]);
}
}
/**
* 正整数
*/
public long toLong(String s) {
int len;
if (s == null || (len = s.length()) == 0) return -1L;
int idx = len - 1;
int bit = chars.length;
long sum = 0;
while (idx >= 0) {
sum += charIndex.idx(s.charAt(idx)) * Math.pow(bit, len - 1 - idx);
--idx;
}
return sum;
}
/**
* 正整数
*/
public String fromLong(long value) {
int bit = chars.length;
if (value < bit) return "" + (value <= 0L ? chars[0] : chars[(int) value]);
if (value == bit) return "" + chars[1] + chars[0];
CArrayStack stack = CArrayStack.of();
long m;
while ((m = value / bit) > 0L) {
stack.push(chars[(int) (value % bit)]);
value = m;
}
StringBuilder sb = new StringBuilder(stack.size() + 1);
sb.append(chars[(int) (value % bit)]);
while (!stack.isEmpty()) sb.append(stack.pop());
return sb.toString();
}
private void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject();
}
private void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject();
init();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy