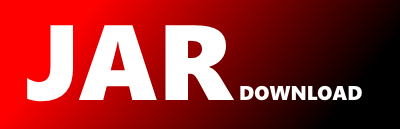
shz.core.structure.config.MapConfig Maven / Gradle / Ivy
package shz.core.structure.config;
import shz.core.structure.FilterContainer;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
public class MapConfig implements FilterContainer, Serializable {
private static final long serialVersionUID = -8791708757295811301L;
protected final Map configs = new HashMap<>();
public final Map getConfigs() {
return configs;
}
public boolean has(int value) {
if (value <= 0) return true;
Long config = configs.get(value / Long.SIZE);
return config != null && (config & 1L << value % Long.SIZE) != 0;
}
public final boolean nonHas(int value) {
return !has(value);
}
public boolean hasAny(int... values) {
for (int value : values) if (has(value)) return true;
return false;
}
public final boolean nonHasAny(int... values) {
return !hasAny(values);
}
public boolean hasAll(int... values) {
for (int value : values) if (nonHas(value)) return false;
return true;
}
public final boolean nonHasAll(int... values) {
return !hasAll(values);
}
public void add(int value) {
if (value <= 0) return;
configs.merge(value / Long.SIZE, 1L << value % Long.SIZE, (a, b) -> a | b);
}
public void add(int... values) {
for (int value : values) add(value);
}
public void remove(int value) {
if (value <= 0) return;
int idx = value / Long.SIZE;
Long config = configs.get(idx);
if (config == null || config == 0L) return;
configs.put(idx, config & ~(1L << value % Long.SIZE));
}
public void remove(int... values) {
for (int value : values) remove(value);
}
public boolean contains(MapConfig other) {
if (other == null || other == NULL) return true;
if (other == ALL) return false;
if (other.configs.isEmpty()) return true;
if (other.configs.size() > configs.size()) return false;
for (Map.Entry entry : other.configs.entrySet()) {
Long value = configs.get(entry.getKey());
if (value == null) return false;
long otherValue = entry.getValue();
if (otherValue > 0L && (otherValue ^ (value & otherValue)) != 0L) return false;
}
return true;
}
public final boolean nonContains(MapConfig other) {
return !contains(other);
}
public boolean eq(MapConfig other) {
if (other == null || other == NULL || other == ALL) return false;
if (other.configs.isEmpty()) return configs.isEmpty();
if (other.configs.size() != configs.size()) return false;
for (Map.Entry entry : other.configs.entrySet()) {
Long value = configs.get(entry.getKey());
if (value == null) return false;
long otherValue = entry.getValue();
if (otherValue != value) return false;
}
return true;
}
public boolean gt(MapConfig other) {
if (other == null || other == NULL) return true;
if (other == ALL) return false;
if (other.configs.isEmpty()) return !configs.isEmpty();
if (other.configs.size() > configs.size()) return false;
boolean gt = false;
for (Map.Entry entry : other.configs.entrySet()) {
Long value = configs.get(entry.getKey());
if (value == null) return false;
long otherValue = entry.getValue();
if (otherValue > 0L && (otherValue ^ (value & otherValue)) != 0L) return false;
if (!gt) gt = otherValue != value;
}
return gt;
}
public MapConfig join(MapConfig other) {
if (other == null || other == NULL || other == ALL || other.configs.isEmpty()) return this;
other.configs.forEach((idx, otherValue) -> {
if (otherValue == 0L) return;
configs.merge(idx, otherValue, (a, b) -> a | b);
});
return this;
}
public MapConfig clear(MapConfig other) {
if (other == null || other == NULL || other == ALL || other.configs.isEmpty()) return this;
other.configs.forEach((idx, otherValue) -> {
if (otherValue == 0L) return;
Long value = configs.get(idx);
if (value == null || value == 0L) return;
configs.put(idx, value ^ (value & otherValue));
});
return this;
}
public static final MapConfig ALL = new MapConfig() {
private static final long serialVersionUID = -2806897414461908299L;
@Override
public boolean has(int value) {
return true;
}
@Override
public boolean hasAny(int... values) {
return true;
}
@Override
public boolean hasAll(int... values) {
return true;
}
@Override
public void add(int value) {
}
@Override
public void add(int... values) {
}
@Override
public void remove(int value) {
}
@Override
public void remove(int... values) {
}
@Override
public boolean contains(MapConfig other) {
return other != this;
}
@Override
public boolean eq(MapConfig other) {
return other == this;
}
@Override
public boolean gt(MapConfig other) {
return other != this;
}
@Override
public MapConfig join(MapConfig other) {
return this;
}
@Override
public MapConfig clear(MapConfig other) {
return this;
}
@Override
public void push(Object key) {
}
@Override
public void push(Object... keys) {
}
@Override
public boolean contain(Object key) {
return true;
}
@Override
public boolean contain(Object... keys) {
return true;
}
};
public static final MapConfig NULL = new MapConfig() {
private static final long serialVersionUID = 1702531257188956778L;
@Override
public boolean has(int value) {
return false;
}
@Override
public boolean hasAny(int... values) {
return false;
}
@Override
public boolean hasAll(int... values) {
return false;
}
@Override
public void add(int value) {
}
@Override
public void add(int... values) {
}
@Override
public void remove(int value) {
}
@Override
public void remove(int... values) {
}
@Override
public boolean contains(MapConfig other) {
return false;
}
@Override
public boolean eq(MapConfig other) {
return other == this;
}
@Override
public boolean gt(MapConfig other) {
return false;
}
@Override
public MapConfig join(MapConfig other) {
return this;
}
@Override
public MapConfig clear(MapConfig other) {
return this;
}
@Override
public void push(Object key) {
}
@Override
public void push(Object... keys) {
}
@Override
public boolean contain(Object key) {
return false;
}
@Override
public boolean contain(Object... keys) {
return false;
}
};
@Override
public void push(Object key) {
if (key instanceof Integer) add((int) key);
}
@Override
public void push(Object... keys) {
for (Object key : keys) push(key);
}
@Override
public boolean contain(Object key) {
return (key instanceof Integer) && has((int) key);
}
@Override
public boolean contain(Object... keys) {
for (Object key : keys) if (!contain(key)) return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy