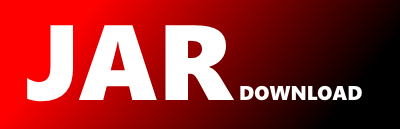
shz.spring.api.ApiMethodAndPathGetter Maven / Gradle / Ivy
package shz.spring.api;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PatchMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import shz.core.net.api.ApiMethodAndPath;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
public final class ApiMethodAndPathGetter {
private static final ApiMethodAndPath NULL = new ApiMethodAndPath();
private static final Map, Map> CACHE = new ConcurrentHashMap<>();
public static ApiMethodAndPath get(Class> cls, Method method) {
ApiMethodAndPath mp = CACHE.computeIfAbsent(cls, k -> new ConcurrentHashMap<>()).computeIfAbsent(method, k -> {
ApiMethodAndPath methodAndPath = methodAndPath(cls.getAnnotation(RequestMapping.class), a -> new Info(a.path(), a.value(), null));
String basePath = methodAndPath == null ? "" : methodAndPath.getPath();
methodAndPath = methodAndPath(method.getAnnotation(RequestMapping.class), a -> new Info(a.path(), a.value(), a.method().length == 0 ? "GET" : a.method()[0].name()));
if (methodAndPath == null)
methodAndPath = methodAndPath(method.getAnnotation(GetMapping.class), a -> new Info(a.path(), a.value(), "GET"));
if (methodAndPath == null)
methodAndPath = methodAndPath(method.getAnnotation(PostMapping.class), a -> new Info(a.path(), a.value(), "POST"));
if (methodAndPath == null)
methodAndPath = methodAndPath(method.getAnnotation(PutMapping.class), a -> new Info(a.path(), a.value(), "PUT"));
if (methodAndPath == null)
methodAndPath = methodAndPath(method.getAnnotation(PatchMapping.class), a -> new Info(a.path(), a.value(), "PATCH"));
if (methodAndPath == null)
methodAndPath = methodAndPath(method.getAnnotation(DeleteMapping.class), a -> new Info(a.path(), a.value(), "DELETE"));
if (methodAndPath != null) return methodAndPath.jointBasePath(basePath);
return NULL;
});
return mp == NULL ? null : mp;
}
private static final class Info {
String[] path;
String[] value;
String method;
Info(String[] path, String[] value, String method) {
this.path = path;
this.value = value;
this.method = method;
}
}
private static ApiMethodAndPath methodAndPath(A a, Function func) {
if (a == null) return null;
Info info = func.apply(a);
ApiMethodAndPath methodAndPath = new ApiMethodAndPath();
methodAndPath.setMethod(info.method);
methodAndPath.setPath(info.path.length != 0 ? info.path[0] : info.value.length != 0 ? info.value[0] : "");
return methodAndPath;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy