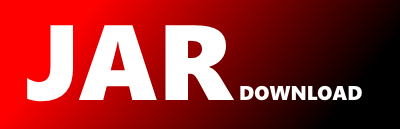
shz.spring.cron.CronDelayQueue Maven / Gradle / Ivy
package shz.spring.cron;
import org.springframework.scheduling.support.CronExpression;
import shz.core.accept.DelayQueue;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.sql.Timestamp;
import java.time.LocalDateTime;
public class CronDelayQueue extends DelayQueue {
public abstract static class CronDelayEntry implements DelayQueue.Entry {
private static final long serialVersionUID = 2333247068228520768L;
private final String expression;
private transient CronExpression cronExpression;
private long nextTimestamp;
protected CronDelayEntry(String expression) {
this.expression = expression;
cronExpression = CronExpression.parse(expression);
nextTimestamp = nextTimestamp();
if (nextTimestamp == 0L) throw new IllegalArgumentException("不能执行的cron表达式");
}
protected long nextTimestamp() {
LocalDateTime next = cronExpression.next(LocalDateTime.now());
return next == null ? 0L : Timestamp.valueOf(next).getTime();
}
@Override
public final long getTimestamp() {
return nextTimestamp;
}
@Override
public final void setTimestamp(long timestamp) {
nextTimestamp = timestamp;
}
protected abstract void action0();
@Override
public final long action() {
action0();
return nextTimestamp();
}
public final String getExpression() {
return expression;
}
public final CronExpression getCronExpression() {
return cronExpression;
}
protected void writeObject(ObjectOutputStream oos) throws IOException {
oos.defaultWriteObject();
}
protected void readObject(ObjectInputStream ois) throws IOException, ClassNotFoundException {
ois.defaultReadObject();
cronExpression = CronExpression.parse(expression);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy