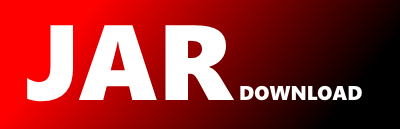
shz.spring.session.Session Maven / Gradle / Ivy
package shz.spring.session;
import java.io.Serializable;
import java.time.LocalDateTime;
public final class Session implements Serializable {
private static final long serialVersionUID = -7173552685161154145L;
/**
* 主键
*/
private Long id;
/**
* 轨迹编号
*/
private String traceNo;
/**
* 应用程序名
*/
private String appName;
/**
* 创建时间
*/
private LocalDateTime createTime;
/**
* 销毁时间
*/
private LocalDateTime destroyedTime;
/**
* 耗时(ms)
*/
private Long elapsedTime;
/**
* 是否登入
*/
private Boolean login;
/**
* 登入账号id
*/
private Long userid;
/**
* 登入角色id
*/
private Long roleId;
/**
* ip地址
*/
private String ip;
/**
* mac地址
*/
private String mac;
/**
* 访问路径
*/
private String path;
/**
* 请求方法
*/
private String method;
/**
* 链接来源
*/
private String referer;
/**
* 是否异常
*/
private Boolean exception;
/**
* 异常编码
*/
private Integer exceptionCode;
/**
* 异常信息
*/
private String exceptionMsg;
/**
* 浏览器
*/
private String browser;
/**
* 浏览器版本
*/
private String browserVersion;
/**
* 操作系统
*/
private String os;
/**
* 是否dba
*/
private boolean dba;
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getTraceNo() {
return traceNo;
}
public void setTraceNo(String traceNo) {
this.traceNo = traceNo;
}
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public LocalDateTime getCreateTime() {
return createTime;
}
public void setCreateTime(LocalDateTime createTime) {
this.createTime = createTime;
}
public LocalDateTime getDestroyedTime() {
return destroyedTime;
}
public void setDestroyedTime(LocalDateTime destroyedTime) {
this.destroyedTime = destroyedTime;
}
public Long getElapsedTime() {
return elapsedTime;
}
public void setElapsedTime(Long elapsedTime) {
this.elapsedTime = elapsedTime;
}
public Boolean getLogin() {
return login;
}
public void setLogin(Boolean login) {
this.login = login;
}
public Long getUserid() {
return userid;
}
public void setUserid(Long userid) {
this.userid = userid;
}
public Long getRoleId() {
return roleId;
}
public void setRoleId(Long roleId) {
this.roleId = roleId;
}
public String getIp() {
return ip;
}
public void setIp(String ip) {
this.ip = ip;
}
public String getMac() {
return mac;
}
public void setMac(String mac) {
this.mac = mac;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getMethod() {
return method;
}
public void setMethod(String method) {
this.method = method;
}
public String getReferer() {
return referer;
}
public void setReferer(String referer) {
this.referer = referer;
}
public Boolean getException() {
return exception;
}
public void setException(Boolean exception) {
this.exception = exception;
}
public Integer getExceptionCode() {
return exceptionCode;
}
public void setExceptionCode(Integer exceptionCode) {
this.exceptionCode = exceptionCode;
}
public String getExceptionMsg() {
return exceptionMsg;
}
public void setExceptionMsg(String exceptionMsg) {
this.exceptionMsg = exceptionMsg;
}
public String getBrowser() {
return browser;
}
public void setBrowser(String browser) {
this.browser = browser;
}
public String getBrowserVersion() {
return browserVersion;
}
public void setBrowserVersion(String browserVersion) {
this.browserVersion = browserVersion;
}
public String getOs() {
return os;
}
public void setOs(String os) {
this.os = os;
}
public boolean isDba() {
return dba;
}
public void setDba(boolean dba) {
this.dba = dba;
}
@Override
public String toString() {
return "Session{" +
"id=" + id +
", traceNo='" + traceNo + '\'' +
", appName='" + appName + '\'' +
", createTime=" + createTime +
", destroyedTime=" + destroyedTime +
", elapsedTime=" + elapsedTime +
", login=" + login +
", userid=" + userid +
", roleId=" + roleId +
", ip='" + ip + '\'' +
", mac='" + mac + '\'' +
", path='" + path + '\'' +
", method='" + method + '\'' +
", referer='" + referer + '\'' +
", exception=" + exception +
", exceptionCode=" + exceptionCode +
", exceptionMsg='" + exceptionMsg + '\'' +
", browser='" + browser + '\'' +
", browserVersion='" + browserVersion + '\'' +
", os='" + os + '\'' +
", dba=" + dba +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy