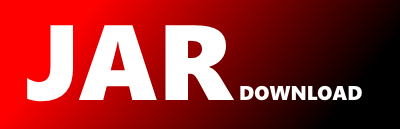
com.sibvisions.rad.ui.vaadin.impl.container.AbstractVaadinWindow Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jvxvaadin-server Show documentation
Show all versions of jvxvaadin-server Show documentation
Vaadin UI implementation for JVx
The newest version!
/*
* Copyright 2012 SIB Visions GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
*
* History
*
* 17.10.2012 - [CB] - creation
*/
package com.sibvisions.rad.ui.vaadin.impl.container;
import jvx.rad.ui.container.IWindow;
import jvx.rad.ui.event.UIWindowEvent;
import jvx.rad.ui.event.WindowHandler;
import jvx.rad.ui.event.type.window.IWindowActivatedListener;
import jvx.rad.ui.event.type.window.IWindowClosedListener;
import jvx.rad.ui.event.type.window.IWindowClosingListener;
import jvx.rad.ui.event.type.window.IWindowDeactivatedListener;
import jvx.rad.ui.event.type.window.IWindowDeiconifiedListener;
import jvx.rad.ui.event.type.window.IWindowIconifiedListener;
import jvx.rad.ui.event.type.window.IWindowOpenedListener;
import jvx.rad.util.TranslationMap;
import com.sibvisions.rad.ui.vaadin.impl.VaadinSingleComponentContainer;
import com.vaadin.ui.SingleComponentContainer;
/**
* The AbstractVaadinWindow
class is the vaadin implementation of {@link IWindow}.
*
* @author Benedikt Cermak
* @param an instance of {@link SingleComponentContainer}
*/
public abstract class AbstractVaadinWindow extends VaadinSingleComponentContainer
implements IWindow
{
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Class members
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/** EventHandler for windowOpened. */
protected WindowHandler eventWindowOpened = null;
/** EventHandler for windowClosing. */
protected WindowHandler eventWindowClosing = null;
/** EventHandler for windowClosed. */
protected WindowHandler eventWindowClosed = null;
/** EventHandler for windowActivated. */
protected WindowHandler eventWindowActivated = null;
/** EventHandler for windowDeactivated. */
protected WindowHandler eventWindowDeactivated = null;
/** EventHandler for windowIconified. */
protected WindowHandler eventWindowIconified = null;
/** EventHandler for windowDeiconified. */
protected WindowHandler eventWindowDeiconified = null;
/** the translation mapping. */
private TranslationMap translation;
/** Active Flag. */
private boolean active = false;
/** whether this frame is disposed. */
private boolean disposed = false;
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Initialization
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* Creates a new instance of AbstractVaadinWindow
.
*
* @param pContainer an instance of a {@link com.vaadin.ui.ComponentContainer}
*/
protected AbstractVaadinWindow(C pContainer)
{
super(pContainer);
setVisible(false);
}
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Interface implementation
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* {@inheritDoc}
*/
public void pack()
{
//Not supported
}
/**
* {@inheritDoc}
*/
public void dispose()
{
disposed = true;
}
/**
* {@inheritDoc}
*/
public boolean isDisposed()
{
return disposed;
}
/**
* {@inheritDoc}
*/
public void toBack()
{
//Not suppported
}
/**
* {@inheritDoc}
*/
public void setTranslation(TranslationMap pTranslation)
{
translation = pTranslation;
}
/**
* {@inheritDoc}
*/
public TranslationMap getTranslation()
{
return translation;
}
/**
* {@inheritDoc}
*/
public boolean isActive()
{
return active;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowOpened()
{
if (eventWindowOpened == null)
{
eventWindowOpened = new WindowHandler(IWindowOpenedListener.class);
}
return eventWindowOpened;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowClosing()
{
if (eventWindowClosing == null)
{
eventWindowClosing = new WindowHandler(IWindowClosingListener.class);
}
return eventWindowClosing;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowClosed()
{
if (eventWindowClosed == null)
{
eventWindowClosed = new WindowHandler(IWindowClosedListener.class);
}
return eventWindowClosed;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowIconified()
{
if (eventWindowIconified == null)
{
eventWindowIconified = new WindowHandler(IWindowIconifiedListener.class);
}
return eventWindowIconified;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowDeiconified()
{
if (eventWindowDeiconified == null)
{
eventWindowDeiconified = new WindowHandler(IWindowDeiconifiedListener.class);
}
return eventWindowDeiconified;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowActivated()
{
if (eventWindowActivated == null)
{
eventWindowActivated = new WindowHandler(IWindowActivatedListener.class);
}
return eventWindowActivated;
}
/**
* {@inheritDoc}
*/
public WindowHandler eventWindowDeactivated()
{
if (eventWindowDeactivated == null)
{
eventWindowDeactivated = new WindowHandler(IWindowDeactivatedListener.class);
}
return eventWindowDeactivated;
}
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// User-defined methods
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* Sets window active flag.
*
* @param pActive
* active flag
*/
public final void setActive(boolean pActive)
{
if (active != pActive)
{
active = pActive;
if (active)
{
if (eventWindowActivated != null)
{
getFactory().synchronizedDispatchEvent(eventWindowActivated, new UIWindowEvent(eventSource, UIWindowEvent.WINDOW_ACTIVATED,
System.currentTimeMillis(), 0));
}
}
else
{
if (eventWindowDeactivated != null)
{
getFactory().synchronizedDispatchEvent(eventWindowDeactivated, new UIWindowEvent(eventSource, UIWindowEvent.WINDOW_DEACTIVATED,
System.currentTimeMillis(), 0));
}
}
}
}
/**
* Sends the window closing event.
*/
public void performWindowClosing()
{
if (eventWindowClosing != null)
{
getFactory().synchronizedDispatchEvent(eventWindowClosing, new UIWindowEvent(eventSource, UIWindowEvent.WINDOW_CLOSING,
System.currentTimeMillis(), 0));
}
}
} // AbstractVaadinWindow
© 2015 - 2025 Weber Informatics LLC | Privacy Policy