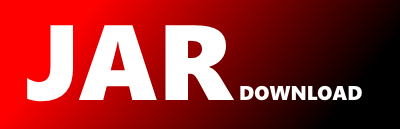
org.vaadin.viritin.util.BrowserCookie Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jvxvaadin-server Show documentation
Show all versions of jvxvaadin-server Show documentation
Vaadin UI implementation for JVx
The newest version!
/*
* Copyright 2015 SIB Visions GmbH
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of
* the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
*
* History
*
* 15.04.2013 - [JR] - creation
*/
package org.vaadin.viritin.util;
import java.time.ZoneId;
import java.time.ZoneOffset;
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
import java.util.Calendar;
import java.util.Date;
import java.util.UUID;
import javax.servlet.http.Cookie;
import com.vaadin.ui.JavaScript;
import com.vaadin.ui.JavaScriptFunction;
import elemental.json.JsonArray;
/**
* A helper that provides access to browser cookies.
*
* @author Matti Tahvonen
*/
public final class BrowserCookie
{
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Initialization
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* Invisible constructor because BrowserCookie
is a utility
* class.
*/
private BrowserCookie()
{
}
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// User-defined methods
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* Sets a cookie via {@link JavaScript} call.
*
* @param pName the cookie name
* @param pValue the cookie value
*/
public static void setCookie(String pName, String pValue)
{
setCookie(pName, pValue, "/", null);
}
/**
* Sets a cookie via {@link JavaScript} call.
*
* @param pName the cookie name
* @param pValue the cookie value
* @param pExpiration the expiration
*/
public static void setCookie(String pName, String pValue, Date pExpiration)
{
setCookie(pName, pValue, "/", pExpiration);
}
/**
* Sets a cookie via {@link JavaScript} call.
*
* @param pName the cookie name
* @param pValue the cookie value
* @param pPath the cookie path
*/
public static void setCookie(String pName, String pValue, String pPath)
{
setCookie(pName, pValue, pPath, null);
}
/**
* Sets a cookie via {@link JavaScript} call.
*
* @param pCookie the cookie
*/
public static void setCookie(Cookie pCookie)
{
if (pCookie.getMaxAge() == 0)
{
setCookie(pCookie.getName(), null, null, null);
}
else
{
Calendar c = Calendar.getInstance();
c.add(Calendar.SECOND, pCookie.getMaxAge());
setCookie(pCookie.getName(), pCookie.getValue(), pCookie.getPath(), c.getTime());
}
}
/**
* Sets a cookie via {@link JavaScript} call.
*
* @param pName the cookie name
* @param pValue the cookie value
* @param pPath the cookie path
* @param pExpiration the expiration
*/
public static void setCookie(String pName, String pValue, String pPath, Date pExpiration)
{
StringBuilder sb = new StringBuilder();
if (pValue == null)
{
sb.append("date = new Date();date.setDate(date.getDate() -1); ");
}
sb.append("document.cookie = \"");
sb.append(pName);
sb.append("=");
if (pValue == null)
{
sb.append("-");
sb.append("; expires=\" + date;");
}
else
{
sb.append(pValue);
sb.append(";");
if (pPath != null)
{
sb.append("path=");
sb.append(pPath);
sb.append(";");
}
if (pExpiration != null)
{
sb.append("expires=");
sb.append(toCookieGMTDate(pExpiration));
sb.append(";");
}
sb.append("\";");
}
JavaScript.getCurrent().execute(sb.toString());
}
/**
* Converts the given date to RFC_1123_DATE_TIME (UTC) formatted string .
*
* @param pExpiration the expiration
* @return the fomatted string
*/
private static String toCookieGMTDate(Date pExpiration)
{
ZonedDateTime zdt = ZonedDateTime.of(pExpiration.toInstant().atZone(ZoneId.systemDefault()).toLocalDateTime(), ZoneOffset.UTC);
return zdt.format(DateTimeFormatter.RFC_1123_DATE_TIME);
}
/**
* Gets a cookie value via {@link JavaScript} call.
*
* @param pName the cookie name
* @param pCallback the callback for the async result
*/
public static void detectCookieValue(String pName, final Callback pCallback)
{
final String sCallbackId = "viritincookiecb" + UUID.randomUUID().toString().substring(0, 8);
JavaScript.getCurrent().addFunction(sCallbackId, new JavaScriptFunction()
{
private static final long serialVersionUID = -3426072590182105863L;
@Override
public void call(JsonArray arguments)
{
JavaScript.getCurrent().removeFunction(sCallbackId);
if (arguments.length() == 0)
{
pCallback.onValueDetected(null);
}
else
{
pCallback.onValueDetected(arguments.getString(0));
}
}
});
JavaScript.getCurrent().execute(String.format("var nameEQ = \"%2$s=\";var ca = document.cookie.split(';');" +
"for(var i=0;i < ca.length;i++) {var c = ca[i];while (c.charAt(0)==' ') c = c.substring(1,c.length); " +
"if (c.indexOf(nameEQ) == 0) {%1$s( c.substring(nameEQ.length,c.length)); return;};} %1$s();",
sCallbackId, pName));
}
//****************************************************************
// Subclass definition
//****************************************************************
/**
* The Callback
is the interface for async results.
*/
public interface Callback
{
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
// Method definitions
//~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
/**
* Will be invoked if value was detected.
*
* @param pValue the detected value
*/
public void onValueDetected(String pValue);
}
} // BrowserCookie
© 2015 - 2025 Weber Informatics LLC | Privacy Policy