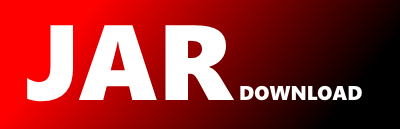
com.signalfx.signalflow.grammar.SignalFlowV2ParserVisitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalflow-grammar Show documentation
Show all versions of signalflow-grammar Show documentation
SignalFx SignalFlow language grammar
// Generated from grammar/SignalFlowV2Parser.g4 by ANTLR 4.5.2
package com.signalfx.signalflow.grammar;
import org.antlr.v4.runtime.tree.ParseTreeVisitor;
/**
* This interface defines a complete generic visitor for a parse tree produced
* by {@link SignalFlowV2Parser}.
*
* @param The return type of the visit operation. Use {@link Void} for
* operations with no return type.
*/
public interface SignalFlowV2ParserVisitor extends ParseTreeVisitor {
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#program}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitProgram(SignalFlowV2Parser.ProgramContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#eval_input}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitEval_input(SignalFlowV2Parser.Eval_inputContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#function_definition}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFunction_definition(SignalFlowV2Parser.Function_definitionContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#parameters}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitParameters(SignalFlowV2Parser.ParametersContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#var_args_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVar_args_list(SignalFlowV2Parser.Var_args_listContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#var_args_list_param_def}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVar_args_list_param_def(SignalFlowV2Parser.Var_args_list_param_defContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#var_args_list_param_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitVar_args_list_param_name(SignalFlowV2Parser.Var_args_list_param_nameContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitStatement(SignalFlowV2Parser.StatementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#simple_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSimple_statement(SignalFlowV2Parser.Simple_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#small_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSmall_statement(SignalFlowV2Parser.Small_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#expr_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpr_statement(SignalFlowV2Parser.Expr_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#id_list}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitId_list(SignalFlowV2Parser.Id_listContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#import_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImport_statement(SignalFlowV2Parser.Import_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#import_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImport_name(SignalFlowV2Parser.Import_nameContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#import_from}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImport_from(SignalFlowV2Parser.Import_fromContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#import_as_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImport_as_name(SignalFlowV2Parser.Import_as_nameContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#dotted_as_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDotted_as_name(SignalFlowV2Parser.Dotted_as_nameContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#import_as_names}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitImport_as_names(SignalFlowV2Parser.Import_as_namesContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#dotted_as_names}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDotted_as_names(SignalFlowV2Parser.Dotted_as_namesContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#dotted_name}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitDotted_name(SignalFlowV2Parser.Dotted_nameContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#return_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitReturn_statement(SignalFlowV2Parser.Return_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#flow_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFlow_statement(SignalFlowV2Parser.Flow_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#compound_statement}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitCompound_statement(SignalFlowV2Parser.Compound_statementContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#suite}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitSuite(SignalFlowV2Parser.SuiteContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#test}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTest(SignalFlowV2Parser.TestContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#lambdef}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitLambdef(SignalFlowV2Parser.LambdefContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#or_test}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitOr_test(SignalFlowV2Parser.Or_testContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#and_test}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAnd_test(SignalFlowV2Parser.And_testContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#not_test}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitNot_test(SignalFlowV2Parser.Not_testContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#comparison}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitComparison(SignalFlowV2Parser.ComparisonContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitExpr(SignalFlowV2Parser.ExprContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#term}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTerm(SignalFlowV2Parser.TermContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#factor}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitFactor(SignalFlowV2Parser.FactorContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#power}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitPower(SignalFlowV2Parser.PowerContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#atom_expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtom_expr(SignalFlowV2Parser.Atom_exprContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#atom}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitAtom(SignalFlowV2Parser.AtomContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#list_expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitList_expr(SignalFlowV2Parser.List_exprContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#tuple_expr}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTuple_expr(SignalFlowV2Parser.Tuple_exprContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#testlist}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTestlist(SignalFlowV2Parser.TestlistContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#trailer}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitTrailer(SignalFlowV2Parser.TrailerContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#actual_args}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitActual_args(SignalFlowV2Parser.Actual_argsContext ctx);
/**
* Visit a parse tree produced by {@link SignalFlowV2Parser#argument}.
* @param ctx the parse tree
* @return the visitor result
*/
T visitArgument(SignalFlowV2Parser.ArgumentContext ctx);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy