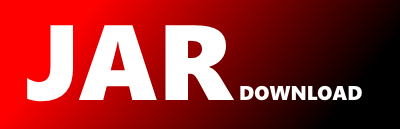
com.signalfx.shaded.apache.commons.io.comparator.package-info Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signalfx-java Show documentation
Show all versions of signalfx-java Show documentation
Bare minimum core library needed to sending metrics to SignalFx from Java clients
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* Provides various {@link java.util.Comparator} implementations
* for {@link java.io.File}s and {@link java.nio.file.Path}.
* Sorting
*
* All the comparators include convenience utility sort(File...)
and
* sort(List)
methods.
*
*
* For example, to sort the files in a directory by name:
*
*
* File[] files = dir.listFiles();
* NameFileComparator.NAME_COMPARATOR.sort(files);
*
*
* ...alternatively you can do this in one line:
*
*
* File[] files = NameFileComparator.NAME_COMPARATOR.sort(dir.listFiles());
*
* Composite Comparator
*
* The CompositeFileComparator can be used
* to compare (and sort lists or arrays of files) by combining a number of other comparators.
*
*
* For example, to sort an array of files by type (i.e. directory or file)
* and then by name:
*
*
* CompositeFileComparator comparator =
* new CompositeFileComparator(
* DirectoryFileComparator.DIRECTORY_COMPARATOR,
* NameFileComparator.NAME_COMPARATOR);
* File[] files = dir.listFiles();
* comparator.sort(files);
*
* Singleton Instances (thread-safe)
*
* The {@link java.util.Comparator} implementations have some convenience
* singleton(thread-safe) instances ready to use:
*
*
* - DefaultFileComparator - default file compare:
*
* - DEFAULT_COMPARATOR
* - Compare using
File.compareTo(File)
method.
*
* - DEFAULT_REVERSE
* - Reverse compare of
File.compareTo(File)
method.
*
*
*
* - DirectoryFileComparator - compare by type (directory or file):
*
* - DIRECTORY_COMPARATOR
* - Compare using
File.isDirectory()
method (directories < files).
*
* - DIRECTORY_REVERSE
* - Reverse compare of
File.isDirectory()
method (directories >files).
*
*
*
* - ExtensionFileComparator - compare file extensions:
*
* - EXTENSION_COMPARATOR
* - Compare using
FilenameUtils.getExtension(String)
method.
*
* - EXTENSION_REVERSE
* - Reverse compare of
FilenameUtils.getExtension(String)
method.
*
* - EXTENSION_INSENSITIVE_COMPARATOR
* - Case-insensitive compare using
FilenameUtils.getExtension(String)
method.
*
* - EXTENSION_INSENSITIVE_REVERSE
* - Reverse case-insensitive compare of
FilenameUtils.getExtension(String)
method.
*
* - EXTENSION_SYSTEM_COMPARATOR
* - System sensitive compare using
FilenameUtils.getExtension(String)
method.
*
* - EXTENSION_SYSTEM_REVERSE
* - Reverse system sensitive compare of
FilenameUtils.getExtension(String)
method.
*
*
*
* - LastModifiedFileComparator
* - compare the file's last modified date/time:
*
* - LASTMODIFIED_COMPARATOR
* - Compare using
File.lastModified()
method.
*
* - LASTMODIFIED_REVERSE
* - Reverse compare of
File.lastModified()
method.
*
*
*
* - NameFileComparator - compare file names:
*
* - NAME_COMPARATOR
* - Compare using
File.getName()
method.
*
* - NAME_REVERSE
* - Reverse compare of
File.getName()
method.
*
* - NAME_INSENSITIVE_COMPARATOR
* - Case-insensitive compare using
File.getName()
method.
*
* - NAME_INSENSITIVE_REVERSE
* - Reverse case-insensitive compare of
File.getName()
method.
*
* - NAME_SYSTEM_COMPARATOR
* - System sensitive compare using
File.getName()
method.
*
* - NAME_SYSTEM_REVERSE
* - Reverse system sensitive compare of
File.getName()
method.
*
*
*
* - PathFileComparator - compare file paths:
*
* - PATH_COMPARATOR
* - Compare using
File.getPath()
method.
*
* - PATH_REVERSE
* - Reverse compare of
File.getPath()
method.
*
* - PATH_INSENSITIVE_COMPARATOR
* - Case-insensitive compare using
File.getPath()
method.
*
* - PATH_INSENSITIVE_REVERSE
* - Reverse case-insensitive compare of
File.getPath()
method.
*
* - PATH_SYSTEM_COMPARATOR
* - System sensitive compare using
File.getPath()
method.
*
* - PATH_SYSTEM_REVERSE
* - Reverse system sensitive compare of
File.getPath()
method.
*
*
*
* - SizeFileComparator - compare the file's size:
*
* - SIZE_COMPARATOR
* - Compare using
File.length()
method (directories treated as zero length).
*
* - LASTMODIFIED_REVERSE
* - Reverse compare of
File.length()
method (directories treated as zero length).
*
* - SIZE_SUMDIR_COMPARATOR
* - Compare using
FileUtils.sizeOfDirectory(File)
method
* (sums the size of a directory's contents).
*
* - SIZE_SUMDIR_REVERSE
* - Reverse compare of
FileUtils.sizeOfDirectory(File)
method
* (sums the size of a directory's contents).
*
*
*
*
*/
package com.signalfx.shaded.apache.commons.io.comparator;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy