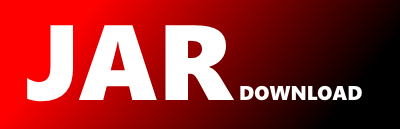
org.opencv.core.Algorithm Maven / Gradle / Ivy
Show all versions of sikulixapi Show documentation
// // This file is auto-generated. Please don't modify it! // package org.opencv.core; import java.lang.String; import java.util.ArrayList; import java.util.List; import org.opencv.utils.Converters; // C++: class Algorithm /** *
* *class CV_EXPORTS_W Algorithm
* *// C++ code:
* * *public:
* *Algorithm();
* *virtual ~Algorithm();
* *string name() const;
* *template
* *typename ParamType<_Tp>.member_type get(const string& * name) const; template
* *typename ParamType<_Tp>.member_type get(const char* * name) const; CV_WRAP int getInt(const string& name) const;
* *CV_WRAP double getDouble(const string& name) const;
* *CV_WRAP bool getBool(const string& name) const;
* *CV_WRAP string getString(const string& name) const;
* *CV_WRAP Mat getMat(const string& name) const;
* *CV_WRAP vector
* *getMatVector(const string& name) const; CV_WRAP Ptr
* *getAlgorithm(const string& name) const; void set(const string& name, int value);
* *void set(const string& name, double value);
* *void set(const string& name, bool value);
* *void set(const string& name, const string& value);
* *void set(const string& name, const Mat& value);
* *void set(const string& name, const vector
* *& value); void set(const string& name, const Ptr
* *& value); template
* *void set(const string& name, const Ptr<_Tp>& value); CV_WRAP void setInt(const string& name, int value);
* *CV_WRAP void setDouble(const string& name, double value);
* *CV_WRAP void setBool(const string& name, bool value);
* *CV_WRAP void setString(const string& name, const string& value);
* *CV_WRAP void setMat(const string& name, const Mat& value);
* *CV_WRAP void setMatVector(const string& name, const vector
* *& value); CV_WRAP void setAlgorithm(const string& name, const Ptr
* *& value); template
* *void setAlgorithm(const string& name, const Ptr<_Tp>& * value); void set(const char* name, int value);
* *void set(const char* name, double value);
* *void set(const char* name, bool value);
* *void set(const char* name, const string& value);
* *void set(const char* name, const Mat& value);
* *void set(const char* name, const vector
* *& value); void set(const char* name, const Ptr
* *& value); template
* *void set(const char* name, const Ptr<_Tp>& value); void setInt(const char* name, int value);
* *void setDouble(const char* name, double value);
* *void setBool(const char* name, bool value);
* *void setString(const char* name, const string& value);
* *void setMat(const char* name, const Mat& value);
* *void setMatVector(const char* name, const vector
* *& value); void setAlgorithm(const char* name, const Ptr
* *& value); template
* *void setAlgorithm(const char* name, const Ptr<_Tp>& * value); CV_WRAP string paramHelp(const string& name) const;
* *int paramType(const char* name) const;
* *CV_WRAP int paramType(const string& name) const;
* *CV_WRAP void getParams(CV_OUT vector
* *& names) const; virtual void write(FileStorage& fs) const;
* *virtual void read(const FileNode& fn);
* *typedef Algorithm* (*Constructor)(void);
* *typedef int (Algorithm.*Getter)() const;
* *typedef void (Algorithm.*Setter)(int);
* *CV_WRAP static void getList(CV_OUT vector
* *& algorithms); CV_WRAP static Ptr
* *_create(const string& name); template
* *static Ptr<_Tp> create(const string& name); virtual AlgorithmInfo* info() const / * TODO: make it = 0;* / { return 0; }
* *};
* *This is a base class for all more or less complex algorithms in OpenCV, * especially for classes of algorithms, for which there can be multiple * implementations. The examples are stereo correspondence (for which there are * algorithms like block matching, semi-global block matching, graph-cut etc.), * background subtraction (which can be done using mixture-of-gaussians models, * codebook-based algorithm etc.), optical flow (block matching, Lucas-Kanade, * Horn-Schunck etc.). *
The class provides the following features for all derived classes:
*
-
*
- so called "virtual constructor". That is, each Algorithm derivative is
* registered at program start and you can get the list of registered algorithms
* and create instance of a particular algorithm by its name (see
*
Algorithm.create
). If you plan to add your own algorithms, it * is good practice to add a unique prefix to your algorithms to distinguish * them from other algorithms. * - setting/retrieving algorithm parameters by name. If you used video
* capturing functionality from OpenCV highgui module, you are probably familar
* with
cvSetCaptureProperty()
,cvGetCaptureProperty()
, *VideoCapture.set()
andVideoCapture.get()
. *Algorithm
provides similar method where instead of integer id's * you specify the parameter names as text strings. SeeAlgorithm.set
* andAlgorithm.get
for details. * - reading and writing parameters from/to XML or YAML files. Every * Algorithm derivative can store all its parameters and then read them back. * There is no need to re-implement it each time. *
Here is example of SIFT use in your application via Algorithm interface:
*
// C++ code:
* *#include "opencv2/opencv.hpp"
* *#include "opencv2/nonfree/nonfree.hpp"...
* *initModule_nonfree(); // to load SURF/SIFT etc.
* *Ptr
FileStorage fs("sift_params.xml", FileStorage.READ);
* *if(fs.isOpened()) // if we have file with parameters, read them
* * *sift->read(fs["sift_params"]);
* *fs.release();
* * *else // else modify the parameters and store them; user can later edit the * file to use different parameters
* * *sift->set("contrastThreshold", 0.01f); // lower the contrast threshold, * compared to the default value
* * *WriteStructContext ws(fs, "sift_params", CV_NODE_MAP);
* *sift->write(fs);
* * * *Mat image = imread("myimage.png", 0), descriptors;
* *vector
(*sift)(image, noArray(), keypoints, descriptors);
* * @see org.opencv.core.Algorithm */ public class Algorithm { protected final long nativeObj; protected Algorithm(long addr) { nativeObj = addr; } // // C++: static Ptr_Algorithm Algorithm::_create(string name) // // Return type 'Ptr_Algorithm' is not supported, skipping the function // // C++: Ptr_Algorithm Algorithm::getAlgorithm(string name) // // Return type 'Ptr_Algorithm' is not supported, skipping the function // // C++: bool Algorithm::getBool(string name) // public boolean getBool(String name) { boolean retVal = getBool_0(nativeObj, name); return retVal; } // // C++: double Algorithm::getDouble(string name) // public double getDouble(String name) { double retVal = getDouble_0(nativeObj, name); return retVal; } // // C++: int Algorithm::getInt(string name) // public int getInt(String name) { int retVal = getInt_0(nativeObj, name); return retVal; } // // C++: static void Algorithm::getList(vector_string& algorithms) // // Unknown type 'vector_string' (O), skipping the function // // C++: Mat Algorithm::getMat(string name) // public Mat getMat(String name) { Mat retVal = new Mat(getMat_0(nativeObj, name)); return retVal; } // // C++: vector_Mat Algorithm::getMatVector(string name) // public List