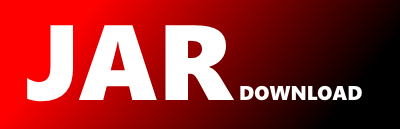
com.simiacryptus.diff.IterativePatchUtil.md Maven / Gradle / Ivy
The `IterativePatchUtil` Kotlin object implements a sophisticated algorithm for generating and applying patches between two versions of textual content, such as source code files. This algorithm is designed to identify differences, categorize them, and efficiently apply those differences to produce the updated text. Below is a comprehensive breakdown of how this patching algorithm operates, including its key components and step-by-step processes.
## **1. Overview**
The `IterativePatchUtil` serves two primary functions:
1. **Generating a Patch**: Comparing an original ("old") version of text with a modified ("new") version to produce a patch that encapsulates the differences.
2. **Applying a Patch**: Taking an original text and a patch to produce the updated text by applying the changes encapsulated in the patch.
The algorithm emphasizes maintaining the structural integrity of the text, especially regarding bracket nesting (parentheses, square brackets, curly braces), and optimizes the patch by minimizing unnecessary changes.
## **2. Core Components**
### **a. Data Structures**
- **`LineType` Enum**: Categorizes each line as one of three types:
- `CONTEXT`: Lines that are unchanged between versions.
- `ADD`: Lines that have been added in the new version.
- `DELETE`: Lines that have been removed from the old version.
- **`LineMetrics` Data Class**: Tracks the nesting depth of different types of brackets within a line:
- `parenthesesDepth`: Depth of `()` brackets.
- `squareBracketsDepth`: Depth of `[]` brackets.
- `curlyBracesDepth`: Depth of `{}` brackets.
- **`LineRecord` Data Class**: Represents a single line in either the source or patch text, containing:
- `index`: Line number.
- `line`: The actual text of the line.
- `previousLine` & `nextLine`: Pointers to adjacent lines.
- `matchingLine`: Links to the corresponding `LineRecord` in the other version.
- `type`: Categorization (`CONTEXT`, `ADD`, `DELETE`).
- `metrics`: Bracket nesting metrics for the line.
### **b. Logging**
Utilizes `SLF4J` (`LoggerFactory`) for logging various stages and actions within the algorithm, aiding in debugging and tracking the patching process.
## **3. Patch Generation Process (`generatePatch`)**
The `generatePatch` function orchestrates the creation of a patch by comparing the old and new versions of the text. Here's a detailed step-by-step breakdown:
### **Step 1: Parsing the Texts**
- **`parseLines` Function**:
- Splits the input text into individual lines.
- Creates `LineRecord` instances for each line.
- Establishes `previousLine` and `nextLine` links between consecutive lines.
- Calculates bracket metrics (`parenthesesDepth`, `squareBracketsDepth`, `curlyBracesDepth`) for each line using the `calculateLineMetrics` function.
### **Step 2: Linking Lines Between Source and New Texts**
- **`link` Function**:
- **Step 1: Linking Unique Exact Matches** (`linkUniqueMatchingLines`):
- Groups lines from both source and new texts based on their normalized content (whitespace removed).
- Identifies lines that appear uniquely and match exactly between both versions.
- Links such lines by setting their `matchingLine` references.
- **Step 2: Linking Adjacent Exact Matches** (`linkAdjacentMatchingLines`):
- Iteratively scans for lines adjacent to already linked lines that match exactly.
- Links these adjacent lines to extend the set of matching lines.
- **Step 3: Subsequence Linking** (`subsequenceLinking`):
- Recursively attempts to link remaining unmatched lines, potentially using Levenshtein distance for lines that are similar but not identical.
- This step ensures that even moved blocks or slightly altered lines are recognized and correctly linked.
### **Step 3: Marking Moved Lines**
- **`markMovedLines` Function**:
- Iterates through the new lines to identify lines that have been moved rather than added or deleted.
- Detects discrepancies in the order of linked lines between source and new texts.
- Marks such lines appropriately by changing their `LineType` to `ADD` or `DELETE` to reflect the movement.
### **Step 4: Generating the Diff**
- **`newToPatch` Function**:
- Traverses through the new lines to construct a preliminary list of differences (`diff`), categorizing each as `ADD`, `DELETE`, or `CONTEXT`.
- For lines with no matching counterpart in the source, marks them as `ADD`.
- For matched lines, checks surrounding lines to identify any `DELETE` operations required.
### **Step 5: Truncating Context**
- **`truncateContext` Function**:
- Limits the number of unchanged (`CONTEXT`) lines around changes to reduce patch size and improve readability.
- Uses a `contextSize` parameter (set to 3) to determine how many context lines to retain before and after changes.
- If there are more context lines than the specified `contextSize`, replaces the excess with an ellipsis (`...`).
### **Step 6: Fixing Patch Line Order**
- **`fixPatchLineOrder` Function**:
- Ensures that `DELETE` lines precede `ADD` lines when they are adjacent in the diff.
- Iteratively swaps lines in the diff to maintain this order, which is essential for properly applying the patch.
### **Step 7: Annihilating No-Op Line Pairs**
- **`annihilateNoopLinePairs` Function**:
- Removes pairs of lines where a line is deleted and then immediately added without any actual change in content.
- Helps in cleaning up the diff by eliminating unnecessary operations that cancel each other out.
### **Step 8: Generating the Final Patch Text**
- Iterates through the processed `shortDiff` list to build the final patch string.
- Formats each line based on its `LineType`:
- `CONTEXT`: Prefixes the line with two spaces (` `).
- `ADD`: Prefixes the line with a plus sign (`+`).
- `DELETE`: Prefixes the line with a minus sign (`-`).
- Joins all lines into a single string, trimming any trailing whitespace.
## **4. Patch Application Process (`applyPatch`)**
The `applyPatch` function applies a previously generated patch to an original text to produce the updated text. Here's how it works:
### **Step 1: Parsing the Source and Patch Texts**
- **Parsing Source Text**:
- Uses `parseLines` to parse the original text into `LineRecord` instances, similar to the patch generation process.
- **Parsing Patch Text**:
- Uses `parsePatchLines` to parse the patch.
- **`parsePatchLines` Function**:
- Splits the patch into lines.
- Identifies the type of each line (`ADD`, `DELETE`, `CONTEXT`) based on prefixes (`+`, `-`, or none).
- Filters out metadata lines (e.g., lines starting with `+++`, `---`, `@@`).
- Establishes `previousLine` and `nextLine` links.
- Calculates bracket metrics.
### **Step 2: Linking Source and Patch Lines**
- **`link` Function**:
- Links lines from the source and patch texts using the same linking steps as in the patch generation process.
- In this context, it uses the `LevenshteinDistance` algorithm to accommodate minor differences between lines, aiding in more accurate linking.
### **Step 3: Filtering Patch Lines**
- Removes any lines in the patch that consist solely of whitespace to prevent unnecessary alterations during patch application.
### **Step 4: Generating the Patched Text**
- **`generatePatchedText` Function**:
- Iterates through the source lines and applies changes based on the linked patch lines.
- Handles different scenarios:
- **Deletion**: Skips lines marked as `DELETE`.
- **Addition**: Inserts lines marked as `ADD`.
- **Context**: Retains lines that haven't changed.
- Ensures that inserted lines are placed correctly by checking for inserts before and after matched lines.
- Reconstructs the updated text by compiling the processed lines into a final string.
## **5. Supporting Functions and Utilities**
### **a. Normalization**
- **`normalizeLine` Function**:
- Removes all whitespace characters from a line to facilitate accurate comparison between lines.
- Helps in identifying lines that are functionally the same but may differ in formatting.
### **b. Line Linking and Matching**
- **`linkUniqueMatchingLines` Function**:
- Links lines that are uniquely identical between source and patch texts.
- Prevents multiple matches by ensuring that only lines with the same count in both texts are linked.
- **`linkAdjacentMatchingLines` Function**:
- Extends existing links by connecting adjacent lines that match, enhancing the continuity of matched blocks.
- **`isMatch` Function**:
- Determines if two lines match exactly or are similar based on the Levenshtein distance.
- Allows for minor discrepancies, improving the algorithm's robustness.
### **c. Bracket Metrics Calculation**
- **`calculateLineMetrics` Function**:
- Analyzes each line to determine the nesting depth of different brackets.
- Useful for understanding the structural context of code or text, aiding in more intelligent patching decisions.
- **`lineMetrics` Extension Function**:
- Provides a convenient way to calculate bracket metrics for individual strings.
## **6. Handling Edge Cases and Optimizations**
- **Recursion Depth Control**:
- The `subsequenceLinking` function limits recursion depth to prevent excessive processing (`depth > 10`), ensuring the algorithm remains efficient.
- **Preventing Infinite Loops**:
- Utilizes `require` statements to ensure that lines are not inadvertently linked to themselves, safeguarding against potential infinite loops during linking.
- **Context Truncation**:
- By limiting the number of context lines, the algorithm reduces patch size and focuses on relevant changes, enhancing readability and manageability.
- **No-Op Pair Removal**:
- Eliminates redundant add-delete pairs, streamlining the patch and avoiding unnecessary modifications.
## **7. Logging and Debugging**
Throughout the algorithm, extensive logging is implemented to track the progress and internal state at various stages:
- **Informational Logs** (`log.info`): Indicate the commencement and completion of major processes like patch generation and application.
- **Debug Logs** (`log.debug`): Provide detailed insights into intermediate steps, such as the number of lines parsed, linked, matched, and any modifications made during processing.
- **Error Logs** (`log.error`): Capture and report any exceptions or critical issues encountered during execution, aiding in troubleshooting.
## **8. Summary of Workflow**
1. **Parsing**: Both the original and new texts are parsed into line-by-line `LineRecord` objects with bracket metrics calculated.
2. **Linking**: The algorithm attempts to link corresponding lines between the original and new texts using exact matches, adjacency, and subsequence strategies, incorporating Levenshtein distance for similar lines.
3. **Marking Movements**: Detects and marks lines that have been moved rather than added or deleted.
4. **Generating Diff**: Creates a preliminary diff list categorizing each line as `ADD`, `DELETE`, or `CONTEXT`.
5. **Optimizing Patch**: Truncates excess context, fixes the order of add/delete operations, and removes no-op pairs to refine the patch.
6. **Finalizing Patch**: Constructs the final patch string by formatting each line based on its type.
7. **Applying Patch**: Repeats parsing and linking steps to apply the patch, reconstructing the updated text by integrating additions, deletions, and retaining unchanged lines.
## **9. Example Scenario**
Consider two versions of a simple source code file:
### **Original (`oldCode`)**
```kotlin
fun add(a: Int, b: Int): Int {
return a + b
}
```
### **Modified (`newCode`)**
```kotlin
fun addNumbers(a: Int, b: Int): Int {
val sum = a + b
return sum
}
```
**Patch Generation (`generatePatch`)** would perform the following:
1. **Identify Changes**:
- Function name changed from `add` to `addNumbers` (`CONTEXT` vs. `ADD`).
- Added a new line declaring `val sum`.
- Modified the return statement to `return sum` (`DELETE` and `ADD`).
2. **Generate Diff**:
- Categorize each line accordingly.
- Truncate any excessive unchanged lines for brevity.
3. **Produce Patch**:
```diff
- fun add(a: Int, b: Int): Int {
+ fun addNumbers(a: Int, b: Int): Int {
+ val sum = a + b
return a + b
- return a + b
+ return sum
}
```
**Applying Patch (`applyPatch`)** would take the original code and the above patch to produce the modified code as shown in `newCode`.
## **10. Advantages and Considerations**
### **Advantages**:
- **Accuracy**: By using multiple linking strategies and considering bracket metrics, the algorithm accurately identifies and applies changes.
- **Efficiency**: Optimizations like context truncation and no-op pair removal reduce patch size and processing time.
- **Robustness**: Incorporation of Levenshtein distance allows for flexibility in handling minor discrepancies or typos.
- **Maintainability**: Clear data structures and modular functions facilitate easy maintenance and potential future enhancements.
### **Considerations**:
- **Complexity**: The algorithm's multiple steps and recursive linking can introduce complexity, making it essential to thoroughly test and debug.
- **Performance**: For very large files, the recursive `subsequenceLinking` and extensive logging might impact performance. Optimizations or limitations on recursion depth help mitigate this.
- **Whitespace Sensitivity**: Normalizing lines by removing all whitespace may lead to unintended matches if significant changes are solely based on formatting.
## **Conclusion**
The `IterativePatchUtil` implements a comprehensive and intelligent patching algorithm that meticulously compares and applies changes between two versions of text. Its multi-faceted approach, incorporating exact matches, adjacency analysis, subsequence linking, bracket metrics, and Levenshtein distance, ensures high accuracy and efficiency in generating and applying patches. While its complexity necessitates careful management and testing, the algorithm offers robust capabilities for handling nuanced changes in textual content, making it highly suitable for applications like version control systems, code editors, and collaborative document editing tools.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy