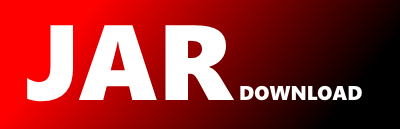
com.simiacryptus.jopenai.util.ListWrapper.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jo-penai Show documentation
Show all versions of jo-penai Show documentation
A Java client for OpenAI's API
package com.simiacryptus.jopenai.util
import com.fasterxml.jackson.core.JsonGenerator
import com.fasterxml.jackson.core.JsonParser
import com.fasterxml.jackson.databind.*
import com.fasterxml.jackson.databind.annotation.JsonDeserialize
import com.fasterxml.jackson.databind.annotation.JsonSerialize
import com.fasterxml.jackson.databind.node.ArrayNode
import com.fasterxml.jackson.module.kotlin.jacksonObjectMapper
@JsonDeserialize(using = ListWrapper.ListWrapperDeserializer::class)
@JsonSerialize(using = ListWrapper.ListWrapperSerializer::class)
open class ListWrapper(
items: List = emptyList()
) : List by items {
open fun deepClone(): ListWrapper? {
return ListWrapper(this.map { it })
}
override fun equals(other: Any?): Boolean {
if (this === other) return true
if (javaClass != other?.javaClass) return false
other as ListWrapper<*>
if (this.size != other.size) return false
return indices.all {
this[it] == other[it]
}
}
override fun hashCode(): Int {
var result = 1
forEach {
result = 31 * result + (it?.hashCode() ?: 0)
}
return result
}
override fun toString(): String {
return joinToString(", ", prefix = "[", postfix = "]")
}
class ListWrapperDeserializer : JsonDeserializer>() {
override fun deserialize(p: JsonParser, ctxt: DeserializationContext): ListWrapper {
val javaType = JsonUtil._initForReading.get()
val node = p.codec.readTree(p)
if (null == node) {
return ListWrapper()
} else if (node.isArray) {
val contextualType = ctxt.contextualType
val contentType = contextualType?.containedType(0) ?: javaType.let {
if(it?.isCollectionLikeType == true) javaType?.containedType(0)
else javaType
}
val objectMapper = JsonUtil.objectMapper()
val items = (node as ArrayNode).toList().map { jsonElement ->
val jsonString = jsonElement.toString()
try {
val readValue = objectMapper.readValue(jsonString, contentType)
readValue
} catch (e: Throwable) {
e.printStackTrace()
null
}
}.filterNotNull()
return ListWrapper(items)
}
// If the node is an object, we assume it's a wrapper object with a single field
val items = jacksonObjectMapper().convertValue(node.fields().next().value, List::class.java)
return ListWrapper(items as List)
}
}
class ListWrapperSerializer : JsonSerializer>() {
override fun serialize(value: ListWrapper, gen: JsonGenerator, serializers: SerializerProvider) {
gen.writeStartArray()
value.forEach {
gen.writeObject(it)
}
gen.writeEndArray()
}
}
}
// object : TypeReference() { override fun getType() = kType.javaType }
© 2015 - 2025 Weber Informatics LLC | Privacy Policy