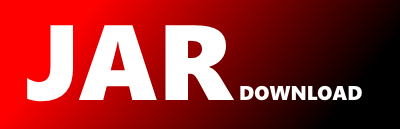
com.simiacryptus.mindseye.lang.cudnn.CudaSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mindseye-cudnn Show documentation
Show all versions of mindseye-cudnn Show documentation
CuDNN Neural Network Components
/*
* Copyright (c) 2019 by Andrew Charneski.
*
* The author licenses this file to you under the
* Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance
* with the License. You may obtain a copy
* of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package com.simiacryptus.mindseye.lang.cudnn;
import com.simiacryptus.lang.Settings;
import com.simiacryptus.lang.ref.PersistanceMode;
import com.simiacryptus.util.JsonUtil;
import com.simiacryptus.util.LocalAppSettings;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.util.HashMap;
public class CudaSettings implements Settings {
private static final Logger logger = LoggerFactory.getLogger(CudaSettings.class);
private static transient CudaSettings INSTANCE = null;
public final String defaultDevices;
public final PersistanceMode memoryCacheMode;
private final long maxTotalMemory;
private final long maxAllocSize;
private final double maxIoElements;
private final long convolutionWorkspaceSizeLimit;
private final boolean disable;
private final boolean forceSingleGpu;
private final long maxFilterElements;
private final boolean conv_para_2;
private final boolean conv_para_1;
private final boolean conv_para_3;
private final long maxDeviceMemory;
private final boolean logStack;
private final boolean profileMemoryIO;
private final boolean asyncFree;
private final boolean enableManaged;
private final boolean syncBeforeFree;
private final int memoryCacheTTL;
private final boolean convolutionCache;
public Precision defaultPrecision;
public boolean allDense;
private int handlesPerDevice;
private CudaSettings() {
HashMap appSettings = LocalAppSettings.read();
String spark_home = System.getenv("SPARK_HOME");
File sparkHomeFile = new File(spark_home == null ? "." : spark_home);
if (sparkHomeFile.exists()) appSettings.putAll(LocalAppSettings.read(sparkHomeFile));
if (appSettings.containsKey("worker.index")) System.setProperty("CUDA_DEVICES", appSettings.get("worker.index"));
maxTotalMemory = (long) Settings.get("MAX_TOTAL_MEMORY", 7.5 * CudaMemory.GiB);
maxDeviceMemory = (long) Settings.get("MAX_DEVICE_MEMORY", 7.5 * CudaMemory.GiB);
maxAllocSize = (long) Settings.get("MAX_ALLOC_SIZE", (double) Precision.Double.size * (Integer.MAX_VALUE / 2 - 1L));
maxFilterElements = (long) Settings.get("MAX_FILTER_ELEMENTS", (double) 512 * CudaMemory.MiB);
maxIoElements = Settings.get("MAX_IO_ELEMENTS", (double) 128 * CudaMemory.MiB);
convolutionWorkspaceSizeLimit = (long) Settings.get("CONVOLUTION_WORKSPACE_SIZE_LIMIT", (double) 512 * CudaMemory.MiB);
disable = Settings.get("DISABLE_CUDNN", false);
forceSingleGpu = Settings.get("FORCE_SINGLE_GPU", true);
conv_para_1 = Settings.get("CONV_PARA_1", false);
conv_para_2 = Settings.get("CONV_PARA_2", false);
conv_para_3 = Settings.get("CONV_PARA_3", false);
memoryCacheMode = Settings.get("CUDA_CACHE_MODE", PersistanceMode.WEAK);
logStack = Settings.get("CUDA_LOG_STACK", false);
profileMemoryIO = Settings.get("CUDA_PROFILE_MEM_IO", false);
enableManaged = false;
asyncFree = true;
syncBeforeFree = false;
memoryCacheTTL = 5;
convolutionCache = true;
defaultDevices = Settings.get("CUDA_DEVICES", "");
this.handlesPerDevice = Settings.get("CUDA_HANDLES_PER_DEVICE", 8);
defaultPrecision = Precision.valueOf(Settings.get("CUDA_DEFAULT_PRECISION", Precision.Float.name()));
allDense = false;
}
public static CudaSettings INSTANCE() {
if (null == INSTANCE) {
synchronized (CudaSettings.class) {
if (null == INSTANCE) {
INSTANCE = new CudaSettings();
logger.info(String.format("Initialized %s = %s", INSTANCE.getClass().getSimpleName(), JsonUtil.toJson(INSTANCE)));
}
}
}
return INSTANCE;
}
public double getMaxTotalMemory() {
return maxTotalMemory;
}
public double getMaxAllocSize() {
return maxAllocSize;
}
public double getMaxIoElements() {
return maxIoElements;
}
public long getConvolutionWorkspaceSizeLimit() {
return convolutionWorkspaceSizeLimit;
}
public boolean isDisable() {
return disable;
}
public boolean isForceSingleGpu() {
return forceSingleGpu;
}
public long getMaxFilterElements() {
return maxFilterElements;
}
public boolean isConv_para_2() {
return conv_para_2;
}
public boolean isConv_para_1() {
return conv_para_1;
}
public boolean isConv_para_3() {
return conv_para_3;
}
public double getMaxDeviceMemory() {
return maxDeviceMemory;
}
public boolean isLogStack() {
return logStack;
}
public boolean isProfileMemoryIO() {
return profileMemoryIO;
}
public boolean isAsyncFree() {
return asyncFree;
}
public boolean isEnableManaged() {
return enableManaged;
}
public boolean isSyncBeforeFree() {
return syncBeforeFree;
}
public int getMemoryCacheTTL() {
return memoryCacheTTL;
}
public boolean isConvolutionCache() {
return convolutionCache;
}
public int getHandlesPerDevice() {
return handlesPerDevice;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy