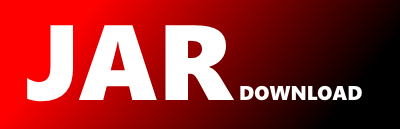
com.simplaex.bedrock.Function2 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bedrock Show documentation
Show all versions of bedrock Show documentation
Essential utilities for modern Java.
package com.simplaex.bedrock;
import javax.annotation.Nonnull;
import java.util.function.BiFunction;
@FunctionalInterface
public interface Function2 extends BiFunction {
@Override
R apply(final A a, final B b);
@Nonnull
default Function1 bind(final A a) {
return (b) -> Function2.this.apply(a, b);
}
@Nonnull
default Function0 bind(final A a, final B b) {
return () -> Function2.this.apply(a, b);
}
@Nonnull
default Function1, R> tupled() {
return (t) -> Function2.this.apply(t.getFirst(), t.getSecond());
}
@Nonnull
default Function1> curried() {
return a -> b -> this.apply(a, b);
}
@Nonnull
default Function2 flipped() {
return new Function2() {
@Override
public R apply(final B b, final A a) {
return Function2.this.apply(a, b);
}
@Override
public Function2 flipped() {
return Function2.this;
}
};
}
@Nonnull
static Function2 from(final BiFunction f) {
return f::apply;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy