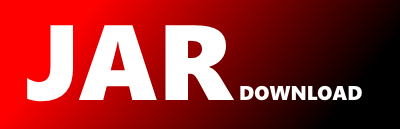
com.simplaex.bedrock.Pair Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bedrock Show documentation
Show all versions of bedrock Show documentation
Essential utilities for modern Java.
The newest version!
package com.simplaex.bedrock;
import com.simplaex.bedrock.hlist.C;
import com.simplaex.bedrock.hlist.HList;
import com.simplaex.bedrock.hlist.Nil;
import lombok.Value;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.Immutable;
import java.io.Serializable;
import java.util.AbstractList;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
/**
* An immutable tuple.
*
* @param The first component of the tuple.
* @param The second component of the tuple.
*/
@Value
@Immutable
public class Pair implements Map.Entry, Serializable, Comparable>, Tuple2 {
private final A first;
private final B second;
public A fst() {
return first;
}
public B snd() {
return second;
}
@Override
public A getKey() {
return first;
}
@Override
public B getValue() {
return second;
}
/**
* Since tuples are immutable this method will throw an UnsupportedOperationException.
* It is only defined to implement the Map.Entry interface.
*
* @param value No matter what you throw in, this method is not supported.
* @return Nothing, it throws an UnsupportedOperationException.
* @throws UnsupportedOperationException Thrown always.
*/
@Override
public B setValue(final B value) throws UnsupportedOperationException {
throw new UnsupportedOperationException();
}
@SuppressWarnings("unchecked")
@Override
public int compareTo(@Nonnull final Pair p) {
final int r;
if (first == null) {
if (p.first == null) {
r = 0;
} else {
return -1;
}
} else if (p.first == null) {
return 1;
} else {
r = ((Comparable) first).compareTo(p.first);
}
if (r != 0) {
return r;
}
if (second == null) {
if (p.second == null) {
return 0;
} else {
return -1;
}
} else if (p.second == null) {
return 1;
} else {
return ((Comparable) second).compareTo(p.second);
}
}
@Nonnull
public static Pair pair(final A a, final B b) {
return new Pair<>(a, b);
}
@Nonnull
public static Pair of(final A a, final B b) {
return new Pair<>(a, b);
}
@Nonnull
public static Pair of(final Map.Entry entry) {
if (entry instanceof Pair) {
return (Pair) entry;
}
return new Pair<>(entry.getKey(), entry.getValue());
}
@Nonnull
public static List toList(@Nonnull final Tuple2 pair) {
return new AbstractList() {
@Override
public C get(final int index) {
switch (index) {
case 0:
return pair.getFirst();
case 1:
return pair.getSecond();
default:
throw new IndexOutOfBoundsException(Integer.toString(index));
}
}
@Override
public int size() {
return 2;
}
};
}
@Nonnull
public Pair swapped() {
return of(getSecond(), getFirst());
}
@Nonnull
public C> toHList() {
return toHList2();
}
public static > Pair fromHList(final C> hlist) {
return pair(hlist.getHead(), hlist.getTail().getHead());
}
@Nonnull
public static Seq toSeq(final Tuple2 pair) {
return Tuple2.toSeq(pair);
}
@Nonnull
public Pair map(final Function f, final Function g) {
return Pair.of(f.apply(fst()), g.apply(snd()));
}
@Nonnull
public Pair mapFirst(final Function f) {
return Pair.of(f.apply(fst()), snd());
}
@Nonnull
public Pair mapSecond(final Function f) {
return Pair.of(fst(), f.apply(snd()));
}
@Nonnull
public Pair withFirst(final C v) {
return Pair.of(v, snd());
}
@Nonnull
public Pair withSecond(final C v) {
return Pair.of(fst(), v);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy