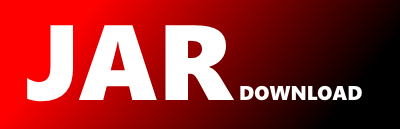
com.sinch.sdk.domains.sms.WebHooksService Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
package com.sinch.sdk.domains.sms;
import com.sinch.sdk.core.exceptions.ApiMappingException;
import com.sinch.sdk.domains.sms.models.BaseDeliveryReport;
import com.sinch.sdk.domains.sms.models.Inbound;
/**
* WebHooks
*
* Callbacks
*
*
A callback is an HTTP POST request with a notification made by the Sinch SMS REST API to a URI
* of your choosing.
*
*
The REST API expects the receiving server to respond with a response code within the 2xx
*
success range. For 5xx
the callback will be retried. For 429
* the callback will be retried and the throughput will be lowered. For other status codes in the
* 4xx
range the callback will not be retried. The first initial retry will happen 5
* seconds after the first try. The next attempt is after 10 seconds, then after 20 seconds, after
* 40 seconds, after 80 seconds, doubling on every attempt. The last retry will be at 81920 seconds
* (or 22 hours 45 minutes) after the initial failed attempt.
*
*
The SMS REST API offers the following callback options which can be configured for your
* account upon request to your account manager.
*
*
* - Callback with mutual authentication over TLS (HTTPS) connection by provisioning the
* callback URL with client keystore and password.
*
- Callback with basic authentication by provisioning the callback URL with username and
* password.
*
- Callback with OAuth 2.0 by provisioning the callback URL with username, password and the
* URL to fetch OAuth access token.
*
- Callback using AWS SNS by provisioning the callback URL with an Access Key ID, Secret Key
* and Region.
*
*
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Webhooks/
* @since 1.0
*/
public interface WebHooksService {
/**
* Incoming SMS WebHook
*
* An inbound message is a message sent to one of your short codes or long numbers from a
* mobile phone. To receive inbound message callbacks, a URL needs to be added to your REST API.
* This URL can be specified in your Dashboard.
*
* @param jsonPayload The incoming message to your sinch number
* @return Decoded payload
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Webhooks/#tag/Webhooks/operation/incomingSMS
* @since 1.0
*/
Inbound> incomingSMS(String jsonPayload) throws ApiMappingException;
/**
* Delivery Report WebHook
*
*
A delivery report contains the status and status code for each recipient of a batch. To get
* a delivery report callback for a message or batch of messages, set the delivery_report
*
field accordingly when creating a batch.
*
*
The following is provided so you can better understand our webhooks/callbacks. Configuration
* of both webhooks and the type of delivery report requested happens when sending a batch.
*
*
Callback URL
*
*
The callback URL can either be provided for each batch or provisioned globally for your
* account in your Sinch Customer
* Dashboard. Learn how to configure a webhook/callback here
*
*
Type
*
*
The type
is the type of delivery report webhook. The response will vary
* depending on the webhook delivery report you selected when the batch was sent, so choose the
* appropriate selection under "One of".
*
*
* - The
delivery_report_sms
and delivery_report_mms
types are
* documented under Delivery report. These are reports containing either
* a full report or summary report, depending on your selection at the time the batch
* was sent.
* - The
recipient_delivery_report_sms
and recipient_delivery_report_mms
*
delivery report types are documented under Recipient delivery report.
* These are delivery reports for recipient phone numbers. If you set per_recipient
*
for the delivery_report
parameter when sending the batch, a
* recipient report gets sent to you for each status change for each recipient in your
* batch. If you set per_recipient_final
, a recipient report gets sent to you
* for the final status of each recipient in your batch.
*
*
* @param jsonPayload The incoming delivery report
* @return Decoded payload
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Webhooks/#tag/Webhooks/operation/deliveryReport
* @since 1.0
*/
BaseDeliveryReport deliveryReport(String jsonPayload) throws ApiMappingException;
}