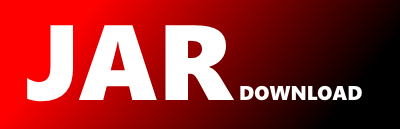
com.sinch.sdk.domains.sms.models.DeliveryReportStatusDetails Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.sms.models;
import java.util.Collection;
/**
* Details related to a delivery report status
*
* @since 1.0
*/
public class DeliveryReportStatusDetails {
private final Integer code;
private final Integer count;
private final Collection recipients;
private final DeliveryReportStatus status;
/**
* @param code Required.
The detailed status
* code.
* @param count Required.
The number of messages that currently has this code
* @param recipients Required.
Only for full report. A list of the phone number
* recipients which messages has this status code
* @param status Required.
The simplified status as described in Delivery Report
* Statuses
*/
public DeliveryReportStatusDetails(
Integer code, Integer count, Collection recipients, String status) {
this.code = code;
this.count = count;
this.recipients = recipients;
this.status = DeliveryReportStatus.from(status);
}
public Integer getCode() {
return code;
}
public Integer getCount() {
return count;
}
public Collection getRecipients() {
return recipients;
}
public DeliveryReportStatus getStatus() {
return status;
}
@Override
public String toString() {
return "DeliveryReportStatusDetails{"
+ "code="
+ code
+ ", count="
+ count
+ ", recipients="
+ recipients
+ ", status="
+ status
+ '}';
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Integer code;
private Integer count;
private Collection recipients;
private DeliveryReportStatus status;
private Builder() {}
public Builder setCode(Integer code) {
this.code = code;
return this;
}
public Builder setCount(Integer count) {
this.count = count;
return this;
}
public Builder setRecipients(Collection recipients) {
this.recipients = recipients;
return this;
}
public Builder setStatus(DeliveryReportStatus status) {
this.status = status;
return this;
}
public DeliveryReportStatusDetails build() {
return new DeliveryReportStatusDetails(code, count, recipients, status.value());
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy