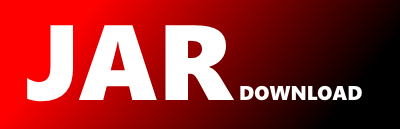
com.sinch.sdk.domains.sms.models.dto.v1.MOTextDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonValue;
import com.sinch.sdk.core.utils.databind.JSONNavigator;
import java.time.OffsetDateTime;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
/** MOTextDto */
@JsonPropertyOrder({MOTextDto.JSON_PROPERTY_BODY, MOTextDto.JSON_PROPERTY_TYPE})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
/*@JsonIgnoreProperties(
value = "type", // ignore manually set type, it will be automatically generated by Jackson during serialization
allowSetters = true // allows the type to be set during deserialization
)*/
@JsonTypeInfo(
use = JsonTypeInfo.Id.NONE,
include = JsonTypeInfo.As.EXISTING_PROPERTY,
property = "type",
visible = true)
public class MOTextDto extends ApiMoMessageDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_BODY = "body";
private String body;
private boolean bodyDefined = false;
/** Regular SMS */
public enum TypeEnum {
MO_TEXT("mo_text"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
private boolean typeDefined = false;
public MOTextDto() {}
@JsonCreator
public MOTextDto(@JsonProperty(JSON_PROPERTY_TYPE) String type) {
this();
this.type = type;
this.typeDefined = true;
}
public MOTextDto body(String body) {
this.body = body;
this.bodyDefined = true;
return this;
}
/**
* Get body
*
* @return body
*/
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getBody() {
return body;
}
@JsonIgnore
public boolean getBodyDefined() {
return bodyDefined;
}
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBody(String body) {
this.body = body;
this.bodyDefined = true;
}
/**
* Regular SMS
*
* @return type
*/
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getType() {
return type;
}
@JsonIgnore
public boolean getTypeDefined() {
return typeDefined;
}
@Override
public MOTextDto from(String from) {
this.setFrom(from);
return this;
}
@Override
public MOTextDto id(String id) {
this.setId(id);
return this;
}
@Override
public MOTextDto receivedAt(OffsetDateTime receivedAt) {
this.setReceivedAt(receivedAt);
return this;
}
@Override
public MOTextDto to(String to) {
this.setTo(to);
return this;
}
@Override
public MOTextDto clientReference(String clientReference) {
this.setClientReference(clientReference);
return this;
}
@Override
public MOTextDto operatorId(String operatorId) {
this.setOperatorId(operatorId);
return this;
}
@Override
public MOTextDto sentAt(OffsetDateTime sentAt) {
this.setSentAt(sentAt);
return this;
}
/** Return true if this MOText object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MOTextDto moText = (MOTextDto) o;
return Objects.equals(this.body, moText.body)
&& Objects.equals(this.type, moText.type)
&& super.equals(o);
}
@Override
public int hashCode() {
return Objects.hash(body, type, super.hashCode());
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MOTextDto {\n");
sb.append(" ").append(toIndentedString(super.toString())).append("\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
static {
// Initialize and register the discriminator mappings.
Map> mappings = new HashMap>();
mappings.put("MOText", MOTextDto.class);
JSONNavigator.registerDiscriminator(MOTextDto.class, "type", mappings);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy