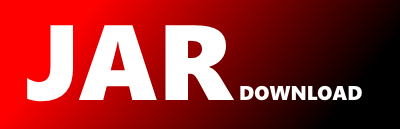
com.sinch.sdk.domains.voice.models.ConferenceDtfmOptions Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
package com.sinch.sdk.domains.voice.models;
import com.sinch.sdk.core.models.OptionalValue;
/**
* Options to control how DTMF signals are used by the participant in the conference. For
* information on how to use this feature, read more
*
* @see Using DTMF
* in conferences
*/
public class ConferenceDtfmOptions {
OptionalValue mode;
OptionalValue maxDigits;
OptionalValue timeoutMills;
public ConferenceDtfmOptions(
OptionalValue mode,
OptionalValue maxDigits,
OptionalValue timeoutMills) {
this.mode = mode;
this.maxDigits = maxDigits;
this.timeoutMills = timeoutMills;
}
public OptionalValue getMode() {
return mode;
}
public OptionalValue getMaxDigits() {
return maxDigits;
}
public OptionalValue getTimeoutMills() {
return timeoutMills;
}
@Override
public String toString() {
return "ConferenceDtfmOptions{"
+ "mode="
+ mode
+ ", maxDigits="
+ maxDigits
+ ", timeoutMills="
+ timeoutMills
+ '}';
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
OptionalValue mode = OptionalValue.empty();
OptionalValue maxDigits = OptionalValue.empty();
OptionalValue timeoutMills = OptionalValue.empty();
public Builder() {}
/**
* Determines what DTMF mode the participant will use in the call.
*
* @param mode DTFM mode
* @return current builder
*/
public Builder setMode(DtfmModeType mode) {
this.mode = OptionalValue.of(mode);
return this;
}
/**
* The maximum number of accepted digits before sending the collected input via a PIE callback.
* The default value is 1. If the value is greater than 1, the PIE callback is triggered by one
* of the three following events:
*
* - No additional digit is entered before the timeoutMills timeout period has elapsed. - The
* # character is entered. - The maximum number of digits has been entered.
*
* @param maxDigits Max digits
* @return current builder
*/
public Builder setMaxDigits(Integer maxDigits) {
this.maxDigits = OptionalValue.of(maxDigits);
return this;
}
/**
* The number of milliseconds that the system will wait between entered digits before triggering
* the PIE callback
*
* @param timeoutMills Timeout in milliseconds
* @return current builder
*/
public Builder setTimeoutMills(Integer timeoutMills) {
this.timeoutMills = OptionalValue.of(timeoutMills);
return this;
}
public ConferenceDtfmOptions build() {
return new ConferenceDtfmOptions(mode, maxDigits, timeoutMills);
}
}
}