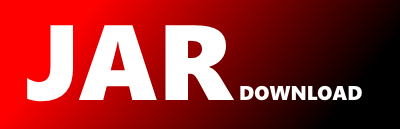
com.sinch.sdk.domains.voice.models.dto.v1.CalloutRequestDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Voice API | Sinch
* The Voice API exposes calling- and conference-related functionality in the Sinch Voice Platform.
*
* The version of the OpenAPI document: 1.0.1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.voice.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.Objects;
/**
* Currently three types of callouts are supported: conference callouts, text-to-speech callouts and
* custom callouts. The custom callout is the most flexible, but text-to-speech and conference
* callouts are more convenient.
*/
@JsonPropertyOrder({
CalloutRequestDto.JSON_PROPERTY_METHOD,
CalloutRequestDto.JSON_PROPERTY_CONFERENCE_CALLOUT,
CalloutRequestDto.JSON_PROPERTY_TTS_CALLOUT,
CalloutRequestDto.JSON_PROPERTY_CUSTOM_CALLOUT
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class CalloutRequestDto {
private static final long serialVersionUID = 1L;
/** Sets the type of callout. */
public enum MethodEnum {
CONFERENCECALLOUT("conferenceCallout"),
TTSCALLOUT("ttsCallout"),
CUSTOMCALLOUT("customCallout"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
MethodEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static MethodEnum fromValue(String value) {
for (MethodEnum b : MethodEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_METHOD = "method";
private String method;
private boolean methodDefined = false;
public static final String JSON_PROPERTY_CONFERENCE_CALLOUT = "conferenceCallout";
private ConferenceCalloutRequestDto conferenceCallout;
private boolean conferenceCalloutDefined = false;
public static final String JSON_PROPERTY_TTS_CALLOUT = "ttsCallout";
private TtsCalloutRequestDto ttsCallout;
private boolean ttsCalloutDefined = false;
public static final String JSON_PROPERTY_CUSTOM_CALLOUT = "customCallout";
private CustomCalloutRequestDto customCallout;
private boolean customCalloutDefined = false;
public CalloutRequestDto() {}
public CalloutRequestDto method(String method) {
this.method = method;
this.methodDefined = true;
return this;
}
/**
* Sets the type of callout.
*
* @return method
*/
@JsonProperty(JSON_PROPERTY_METHOD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getMethod() {
return method;
}
@JsonIgnore
public boolean getMethodDefined() {
return methodDefined;
}
@JsonProperty(JSON_PROPERTY_METHOD)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setMethod(String method) {
this.method = method;
this.methodDefined = true;
}
public CalloutRequestDto conferenceCallout(ConferenceCalloutRequestDto conferenceCallout) {
this.conferenceCallout = conferenceCallout;
this.conferenceCalloutDefined = true;
return this;
}
/**
* Get conferenceCallout
*
* @return conferenceCallout
*/
@JsonProperty(JSON_PROPERTY_CONFERENCE_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ConferenceCalloutRequestDto getConferenceCallout() {
return conferenceCallout;
}
@JsonIgnore
public boolean getConferenceCalloutDefined() {
return conferenceCalloutDefined;
}
@JsonProperty(JSON_PROPERTY_CONFERENCE_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setConferenceCallout(ConferenceCalloutRequestDto conferenceCallout) {
this.conferenceCallout = conferenceCallout;
this.conferenceCalloutDefined = true;
}
public CalloutRequestDto ttsCallout(TtsCalloutRequestDto ttsCallout) {
this.ttsCallout = ttsCallout;
this.ttsCalloutDefined = true;
return this;
}
/**
* Get ttsCallout
*
* @return ttsCallout
*/
@JsonProperty(JSON_PROPERTY_TTS_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public TtsCalloutRequestDto getTtsCallout() {
return ttsCallout;
}
@JsonIgnore
public boolean getTtsCalloutDefined() {
return ttsCalloutDefined;
}
@JsonProperty(JSON_PROPERTY_TTS_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTtsCallout(TtsCalloutRequestDto ttsCallout) {
this.ttsCallout = ttsCallout;
this.ttsCalloutDefined = true;
}
public CalloutRequestDto customCallout(CustomCalloutRequestDto customCallout) {
this.customCallout = customCallout;
this.customCalloutDefined = true;
return this;
}
/**
* Get customCallout
*
* @return customCallout
*/
@JsonProperty(JSON_PROPERTY_CUSTOM_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CustomCalloutRequestDto getCustomCallout() {
return customCallout;
}
@JsonIgnore
public boolean getCustomCalloutDefined() {
return customCalloutDefined;
}
@JsonProperty(JSON_PROPERTY_CUSTOM_CALLOUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCustomCallout(CustomCalloutRequestDto customCallout) {
this.customCallout = customCallout;
this.customCalloutDefined = true;
}
/** Return true if this CalloutRequest object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CalloutRequestDto calloutRequest = (CalloutRequestDto) o;
return Objects.equals(this.method, calloutRequest.method)
&& Objects.equals(this.conferenceCallout, calloutRequest.conferenceCallout)
&& Objects.equals(this.ttsCallout, calloutRequest.ttsCallout)
&& Objects.equals(this.customCallout, calloutRequest.customCallout);
}
@Override
public int hashCode() {
return Objects.hash(method, conferenceCallout, ttsCallout, customCallout);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CalloutRequestDto {\n");
sb.append(" method: ").append(toIndentedString(method)).append("\n");
sb.append(" conferenceCallout: ").append(toIndentedString(conferenceCallout)).append("\n");
sb.append(" ttsCallout: ").append(toIndentedString(ttsCallout)).append("\n");
sb.append(" customCallout: ").append(toIndentedString(customCallout)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy