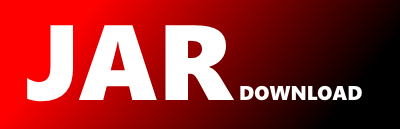
com.sinch.sdk.domains.conversation.api.v1.internal.AppApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Conversation API | Sinch
*
* OpenAPI document version: 1.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.conversation.api.v1.internal;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.conversation.models.v1.app.request.AppCreateRequest;
import com.sinch.sdk.domains.conversation.models.v1.app.request.AppUpdateRequest;
import com.sinch.sdk.domains.conversation.models.v1.app.response.AppResponse;
import com.sinch.sdk.domains.conversation.models.v1.app.response.ListAppsResponse;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class AppApi {
private static final Logger LOGGER = Logger.getLogger(AppApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public AppApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Create an app You can create a new Conversation API app using the API. You can create an app
* for one or more channels at once. The ID of the app is generated at creation and will be
* returned in the response.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param appCreateRequest The app to create. (required)
* @return AppResponse
* @throws ApiException if fails to make API call
*/
public AppResponse appCreateApp(String projectId, AppCreateRequest appCreateRequest)
throws ApiException {
LOGGER.finest(
"[appCreateApp]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "appCreateRequest: "
+ appCreateRequest);
HttpRequest httpRequest = appCreateAppRequestBuilder(projectId, appCreateRequest);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest appCreateAppRequestBuilder(
String projectId, AppCreateRequest appCreateRequest) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling appCreateApp");
}
// verify the required parameter 'appCreateRequest' is set
if (appCreateRequest == null) {
throw new ApiException(
400, "Missing the required parameter 'appCreateRequest' when calling appCreateApp");
}
String localVarPath =
"/v1/projects/{project_id}/apps"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = mapper.serialize(localVarContentTypes, appCreateRequest);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Delete an app Deletes the app specified by the App ID. Note that this operation will not delete
* contacts (which are stored at the project level) nor any channel-specific resources (for
* example, WhatsApp Sender Identities will not be deleted).
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param appId The unique ID of the app. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @throws ApiException if fails to make API call
*/
public void appDeleteApp(String projectId, String appId) throws ApiException {
LOGGER.finest("[appDeleteApp]" + " " + "projectId: " + projectId + ", " + "appId: " + appId);
HttpRequest httpRequest = appDeleteAppRequestBuilder(projectId, appId);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
return;
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest appDeleteAppRequestBuilder(String projectId, String appId)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling appDeleteApp");
}
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(
400, "Missing the required parameter 'appId' when calling appDeleteApp");
}
String localVarPath =
"/v1/projects/{project_id}/apps/{app_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll("\\{" + "app_id" + "\\}", URLPathUtils.encodePathSegment(appId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.DELETE,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Get an app Returns a particular app as specified by the App ID.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param appId The unique ID of the app. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @return AppResponse
* @throws ApiException if fails to make API call
*/
public AppResponse appGetApp(String projectId, String appId) throws ApiException {
LOGGER.finest("[appGetApp]" + " " + "projectId: " + projectId + ", " + "appId: " + appId);
HttpRequest httpRequest = appGetAppRequestBuilder(projectId, appId);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest appGetAppRequestBuilder(String projectId, String appId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling appGetApp");
}
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(400, "Missing the required parameter 'appId' when calling appGetApp");
}
String localVarPath =
"/v1/projects/{project_id}/apps/{app_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll("\\{" + "app_id" + "\\}", URLPathUtils.encodePathSegment(appId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* List all apps for a given project Get a list of all apps in the specified project.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @return ListAppsResponse
* @throws ApiException if fails to make API call
*/
public ListAppsResponse appListApps(String projectId) throws ApiException {
LOGGER.finest("[appListApps]" + " " + "projectId: " + projectId);
HttpRequest httpRequest = appListAppsRequestBuilder(projectId);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest appListAppsRequestBuilder(String projectId) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling appListApps");
}
String localVarPath =
"/v1/projects/{project_id}/apps"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Update an app Updates a particular app as specified by the App ID. Note that this is a
* `PATCH` operation, so any specified field values will replace existing values.
* Therefore, **if you'd like to add additional configurations to an existing Conversation API
* app, ensure that you include existing values AND new values in the call**. For example, if
* you'd like to add new `channel_credentials`, you can
* [get](https://developers.sinch.com/docs/conversation/api-reference/conversation/tag/App/#tag/App/operation/App_GetApp)
* your existing Conversation API app, extract the existing `channel_credentials` list,
* append your new configuration to that list, and include the updated
* `channel_credentials` list in this update call.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param appId The unique ID of the app. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param appUpdateRequest The updated app. (required)
* @param updateMask The set of field mask paths. (optional
* @return AppResponse
* @throws ApiException if fails to make API call
*/
public AppResponse appUpdateApp(
String projectId, String appId, AppUpdateRequest appUpdateRequest, List updateMask)
throws ApiException {
LOGGER.finest(
"[appUpdateApp]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "appId: "
+ appId
+ ", "
+ "appUpdateRequest: "
+ appUpdateRequest
+ ", "
+ "updateMask: "
+ updateMask);
HttpRequest httpRequest =
appUpdateAppRequestBuilder(projectId, appId, appUpdateRequest, updateMask);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest appUpdateAppRequestBuilder(
String projectId, String appId, AppUpdateRequest appUpdateRequest, List updateMask)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling appUpdateApp");
}
// verify the required parameter 'appId' is set
if (appId == null) {
throw new ApiException(
400, "Missing the required parameter 'appId' when calling appUpdateApp");
}
// verify the required parameter 'appUpdateRequest' is set
if (appUpdateRequest == null) {
throw new ApiException(
400, "Missing the required parameter 'appUpdateRequest' when calling appUpdateApp");
}
String localVarPath =
"/v1/projects/{project_id}/apps/{app_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll("\\{" + "app_id" + "\\}", URLPathUtils.encodePathSegment(appId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != updateMask) {
localVarQueryParams.add(
new URLParameter(
"update_mask", updateMask, URLParameter.STYLE.valueOf("form".toUpperCase()), false));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = mapper.serialize(localVarContentTypes, appUpdateRequest);
return new HttpRequest(
localVarPath,
HttpMethod.PATCH,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy