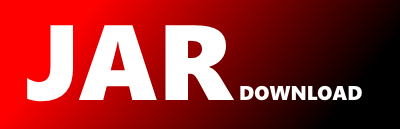
com.sinch.sdk.domains.conversation.api.v1.internal.MessagesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Conversation API | Sinch
*
* OpenAPI document version: 1.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.conversation.api.v1.internal;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.conversation.models.v1.ConversationChannel;
import com.sinch.sdk.domains.conversation.models.v1.internal.ConversationMessageInternal;
import com.sinch.sdk.domains.conversation.models.v1.messages.internal.ListMessagesResponseInternal;
import com.sinch.sdk.domains.conversation.models.v1.messages.request.ConversationMessagesView;
import com.sinch.sdk.domains.conversation.models.v1.messages.request.MessageUpdateRequest;
import com.sinch.sdk.domains.conversation.models.v1.messages.request.SendMessageRequest;
import com.sinch.sdk.domains.conversation.models.v1.messages.response.SendMessageResponse;
import java.time.Instant;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class MessagesApi {
private static final Logger LOGGER = Logger.getLogger(MessagesApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public MessagesApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Delete a message Delete a specific message by its ID. Note: Removing all messages of a
* conversation will not automatically delete the conversation.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param messageId The unique ID of the message. (required)
* @param messagesSource Specifies the message source for which the request will be processed.
* Used for operations on messages in Dispatch Mode. For more information, see [Processing
* Modes](https://developers.sinch.com/docs/conversation/processing-modes/). (optional,
* default to CONVERSATION_SOURCE)
* @throws ApiException if fails to make API call
*/
public void messagesDeleteMessage(String projectId, String messageId, String messagesSource)
throws ApiException {
LOGGER.finest(
"[messagesDeleteMessage]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "messageId: "
+ messageId
+ ", "
+ "messagesSource: "
+ messagesSource);
HttpRequest httpRequest =
messagesDeleteMessageRequestBuilder(projectId, messageId, messagesSource);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
return;
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest messagesDeleteMessageRequestBuilder(
String projectId, String messageId, String messagesSource) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling messagesDeleteMessage");
}
// verify the required parameter 'messageId' is set
if (messageId == null) {
throw new ApiException(
400, "Missing the required parameter 'messageId' when calling messagesDeleteMessage");
}
String localVarPath =
"/v1/projects/{project_id}/messages/{message_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "message_id" + "\\}", URLPathUtils.encodePathSegment(messageId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != messagesSource) {
localVarQueryParams.add(
new URLParameter(
"messages_source",
messagesSource,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.DELETE,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Get a message Retrieves a specific message by its ID.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param messageId The unique ID of the message. (required)
* @param messagesSource Specifies the message source for which the request will be processed.
* Used for operations on messages in Dispatch Mode. For more information, see [Processing
* Modes](https://developers.sinch.com/docs/conversation/processing-modes/). (optional,
* default to CONVERSATION_SOURCE)
* @return ConversationMessageInternal
* @throws ApiException if fails to make API call
*/
public ConversationMessageInternal messagesGetMessage(
String projectId, String messageId, String messagesSource) throws ApiException {
LOGGER.finest(
"[messagesGetMessage]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "messageId: "
+ messageId
+ ", "
+ "messagesSource: "
+ messagesSource);
HttpRequest httpRequest =
messagesGetMessageRequestBuilder(projectId, messageId, messagesSource);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest messagesGetMessageRequestBuilder(
String projectId, String messageId, String messagesSource) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling messagesGetMessage");
}
// verify the required parameter 'messageId' is set
if (messageId == null) {
throw new ApiException(
400, "Missing the required parameter 'messageId' when calling messagesGetMessage");
}
String localVarPath =
"/v1/projects/{project_id}/messages/{message_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "message_id" + "\\}", URLPathUtils.encodePathSegment(messageId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != messagesSource) {
localVarQueryParams.add(
new URLParameter(
"messages_source",
messagesSource,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* List messages This operation lists all messages sent or received via particular [Processing
* Modes](https://developers.sinch.com/docs/conversation/processing-modes/). Setting the
* `messages_source` parameter to `CONVERSATION_SOURCE` allows for querying
* messages in `CONVERSATION` mode, and setting it to `DISPATCH_SOURCE` will
* allow for queries of messages in `DISPATCH` mode. Combining multiple parameters is
* supported for more detailed filtering of messages, but some of them are not supported depending
* on the value specified for `messages_source`. The description for each field will
* inform if that field may not be supported. The messages are ordered by their
* `accept_time` property in descending order, where `accept_time` is a
* timestamp of when the message was enqueued by the Conversation API. This means messages
* received most recently will be listed first.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param conversationId Resource name (ID) of the conversation. (optional)
* @param contactId Resource name (ID) of the contact. Note that either `app_id` or
* `contact_id` is required in order for the operation to function correctly.
* (optional)
* @param appId Id of the app. (optional)
* @param channelIdentity Channel identity of the contact. (optional)
* @param startTime Filter messages with `accept_time` after this timestamp. Must be
* before `end_time` if that is specified. (optional)
* @param endTime Filter messages with `accept_time` before this timestamp. (optional)
* @param pageSize Maximum number of messages to fetch. Defaults to 10 and the maximum is 1000.
* (optional)
* @param pageToken Next page token previously returned if any. When specifying this token, make
* sure to use the same values for the other parameters from the request that originated the
* token, otherwise the paged results may be inconsistent. (optional)
* @param view (optional, default to WITH_METADATA)
* @param messagesSource Specifies the message source for which the request will be processed.
* Used for operations on messages in Dispatch Mode. For more information, see [Processing
* Modes](https://developers.sinch.com/docs/conversation/processing-modes/). (optional,
* default to CONVERSATION_SOURCE)
* @param onlyRecipientOriginated If true, fetch only recipient originated messages. Available
* only when `messages_source` is `DISPATCH_SOURCE`. (optional)
* @param channel Only fetch messages from the `channel`. (optional)
* @return ListMessagesResponseInternal
* @throws ApiException if fails to make API call
*/
public ListMessagesResponseInternal messagesListMessages(
String projectId,
String conversationId,
String contactId,
String appId,
String channelIdentity,
Instant startTime,
Instant endTime,
Integer pageSize,
String pageToken,
ConversationMessagesView view,
String messagesSource,
Boolean onlyRecipientOriginated,
ConversationChannel channel)
throws ApiException {
LOGGER.finest(
"[messagesListMessages]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "conversationId: "
+ conversationId
+ ", "
+ "contactId: "
+ contactId
+ ", "
+ "appId: "
+ appId
+ ", "
+ "channelIdentity: "
+ channelIdentity
+ ", "
+ "startTime: "
+ startTime
+ ", "
+ "endTime: "
+ endTime
+ ", "
+ "pageSize: "
+ pageSize
+ ", "
+ "pageToken: "
+ pageToken
+ ", "
+ "view: "
+ view
+ ", "
+ "messagesSource: "
+ messagesSource
+ ", "
+ "onlyRecipientOriginated: "
+ onlyRecipientOriginated
+ ", "
+ "channel: "
+ channel);
HttpRequest httpRequest =
messagesListMessagesRequestBuilder(
projectId,
conversationId,
contactId,
appId,
channelIdentity,
startTime,
endTime,
pageSize,
pageToken,
view,
messagesSource,
onlyRecipientOriginated,
channel);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest messagesListMessagesRequestBuilder(
String projectId,
String conversationId,
String contactId,
String appId,
String channelIdentity,
Instant startTime,
Instant endTime,
Integer pageSize,
String pageToken,
ConversationMessagesView view,
String messagesSource,
Boolean onlyRecipientOriginated,
ConversationChannel channel)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling messagesListMessages");
}
String localVarPath =
"/v1/projects/{project_id}/messages"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != conversationId) {
localVarQueryParams.add(
new URLParameter(
"conversation_id",
conversationId,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != contactId) {
localVarQueryParams.add(
new URLParameter(
"contact_id", contactId, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != appId) {
localVarQueryParams.add(
new URLParameter(
"app_id", appId, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != channelIdentity) {
localVarQueryParams.add(
new URLParameter(
"channel_identity",
channelIdentity,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != startTime) {
localVarQueryParams.add(
new URLParameter(
"start_time", startTime, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != endTime) {
localVarQueryParams.add(
new URLParameter(
"end_time", endTime, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != pageSize) {
localVarQueryParams.add(
new URLParameter(
"page_size", pageSize, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != pageToken) {
localVarQueryParams.add(
new URLParameter(
"page_token", pageToken, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != view) {
localVarQueryParams.add(
new URLParameter("view", view, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != messagesSource) {
localVarQueryParams.add(
new URLParameter(
"messages_source",
messagesSource,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != onlyRecipientOriginated) {
localVarQueryParams.add(
new URLParameter(
"only_recipient_originated",
onlyRecipientOriginated,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != channel) {
localVarQueryParams.add(
new URLParameter(
"channel", channel, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Send a message You can send a message from a Conversation app to a contact associated with that
* app. If the recipient is not associated with an existing contact, a new contact will be
* created. The message is added to the active conversation with the contact if a conversation
* already exists. If no active conversation exists a new one is started automatically. You can
* find all of your IDs and authentication credentials on the [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/access-keys).
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param sendMessageRequest This is the request body for sending a message. `app_id`,
* `recipient`, and `message` are all required fields. (required)
* @return SendMessageResponse
* @throws ApiException if fails to make API call
*/
public SendMessageResponse messagesSendMessage(
String projectId, SendMessageRequest sendMessageRequest) throws ApiException {
LOGGER.finest(
"[messagesSendMessage]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "sendMessageRequest: "
+ sendMessageRequest);
HttpRequest httpRequest = messagesSendMessageRequestBuilder(projectId, sendMessageRequest);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest messagesSendMessageRequestBuilder(
String projectId, SendMessageRequest sendMessageRequest) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling messagesSendMessage");
}
// verify the required parameter 'sendMessageRequest' is set
if (sendMessageRequest == null) {
throw new ApiException(
400,
"Missing the required parameter 'sendMessageRequest' when calling messagesSendMessage");
}
String localVarPath =
"/v1/projects/{project_id}/messages:send"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = mapper.serialize(localVarContentTypes, sendMessageRequest);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Update message metadata Update a specific message metadata by its ID.
*
* @param projectId The unique ID of the project. You can find this on the [Sinch
* Dashboard](https://dashboard.sinch.com/convapi/apps). (required)
* @param messageId The unique ID of the message. (required)
* @param messageUpdateRequest Update message metadata request. (required)
* @param messagesSource Specifies the message source for which the request will be processed.
* Used for operations on messages in Dispatch Mode. For more information, see [Processing
* Modes](https://developers.sinch.com/docs/conversation/processing-modes/). (optional,
* default to CONVERSATION_SOURCE)
* @return ConversationMessageInternal
* @throws ApiException if fails to make API call
*/
public ConversationMessageInternal messagesUpdateMessageMetadata(
String projectId,
String messageId,
MessageUpdateRequest messageUpdateRequest,
String messagesSource)
throws ApiException {
LOGGER.finest(
"[messagesUpdateMessageMetadata]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "messageId: "
+ messageId
+ ", "
+ "messageUpdateRequest: "
+ messageUpdateRequest
+ ", "
+ "messagesSource: "
+ messagesSource);
HttpRequest httpRequest =
messagesUpdateMessageMetadataRequestBuilder(
projectId, messageId, messageUpdateRequest, messagesSource);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest messagesUpdateMessageMetadataRequestBuilder(
String projectId,
String messageId,
MessageUpdateRequest messageUpdateRequest,
String messagesSource)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling messagesUpdateMessageMetadata");
}
// verify the required parameter 'messageId' is set
if (messageId == null) {
throw new ApiException(
400,
"Missing the required parameter 'messageId' when calling messagesUpdateMessageMetadata");
}
// verify the required parameter 'messageUpdateRequest' is set
if (messageUpdateRequest == null) {
throw new ApiException(
400,
"Missing the required parameter 'messageUpdateRequest' when calling"
+ " messagesUpdateMessageMetadata");
}
String localVarPath =
"/v1/projects/{project_id}/messages/{message_id}"
.replaceAll(
"\\{" + "project_id" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "message_id" + "\\}", URLPathUtils.encodePathSegment(messageId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != messagesSource) {
localVarQueryParams.add(
new URLParameter(
"messages_source",
messagesSource,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("Basic", "oAuth2");
final String serializedBody = mapper.serialize(localVarContentTypes, messageUpdateRequest);
return new HttpRequest(
localVarPath,
HttpMethod.PATCH,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy