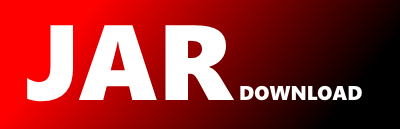
com.sinch.sdk.domains.conversation.models.v1.credentials.ConversationChannelCredentials Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
/*
* Conversation API | Sinch
*
* OpenAPI document version: 1.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.conversation.models.v1.credentials;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.sinch.sdk.domains.conversation.models.v1.ConversationChannel;
/**
* Enables access to the underlying messaging channel.
*
* Use the {@link
* com.sinch.sdk.domains.conversation.models.v1.credentials.ConversationChannelCredentialsBuilderFactory}
* factory builder helper to create credentials related to channel
*
* @see
* com.sinch.sdk.domains.conversation.models.v1.credentials.ConversationChannelCredentialsBuilderFactory
*/
@JsonDeserialize(builder = ConversationChannelCredentialsImpl.Builder.class)
public interface ConversationChannelCredentials {
/**
* Get credentials related to conversation channel
*
* @return credentials
*/
ChannelCredentials getCredentials();
/**
* The secret used to verify the channel callbacks for channels which support callback
* verification. The callback verification is not needed for Sinch-managed channels because the
* callbacks are not leaving Sinch internal networks. Max length is 256 characters. Note: leaving
* channel_callback_secret empty for channels with callback verification will disable the
* verification.
*
* @return callbackSecret
*/
String getCallbackSecret();
/**
* Get channel
*
* @return channel
*/
ConversationChannel getChannel();
/**
* Get state
*
* @return state
* @readOnly This field is returned by the server and cannot be modified
*/
ChannelIntegrationState getState();
/**
* Additional identifier set by the channel that represents an specific id used by the channel.
*
* @return channelKnownId
* @readOnly This field is returned by the server and cannot be modified
*/
String getChannelKnownId();
/**
* Getting builder
*
* @return New Builder instance
*/
static Builder builder() {
return new ConversationChannelCredentialsImpl.Builder();
}
/** Dedicated Builder */
interface Builder {
/**
* see getter
*
* @param credentials see getter
* @return Current builder
* @see #getCredentials
*/
Builder setCredentials(ChannelCredentials credentials);
/**
* see getter
*
* @param callbackSecret see getter
* @return Current builder
* @see #getCallbackSecret
*/
Builder setCallbackSecret(String callbackSecret);
/**
* see getter
*
* @param channel see getter
* @return Current builder
* @see #getChannel
*/
Builder setChannel(ConversationChannel channel);
/**
* see getter
*
* @param state see getter
* @return Current builder
* @see #getState
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setState(ChannelIntegrationState state);
/**
* see getter
*
* @param channelKnownId see getter
* @return Current builder
* @see #getChannelKnownId
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setChannelKnownId(String channelKnownId);
/**
* Create instance
*
* @return The instance build with current builder values
*/
ConversationChannelCredentials build();
}
}