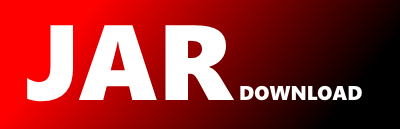
com.sinch.sdk.domains.conversation.models.v1.internal.ConversationMessageInternal Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Conversation API | Sinch
*
* OpenAPI document version: 1.0
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.conversation.models.v1.internal;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.sinch.sdk.domains.conversation.models.v1.ChannelIdentity;
import com.sinch.sdk.domains.conversation.models.v1.ConversationDirection;
import com.sinch.sdk.domains.conversation.models.v1.ProcessingMode;
import com.sinch.sdk.domains.conversation.models.v1.messages.AppMessage;
import com.sinch.sdk.domains.conversation.models.v1.messages.ContactMessage;
import java.time.Instant;
/** A message on a particular channel. */
@JsonDeserialize(builder = ConversationMessageInternalImpl.Builder.class)
public interface ConversationMessageInternal {
/**
* Get appMessage
*
* @return appMessage
*/
AppMessage> getAppMessage();
/**
* Get contactMessage
*
* @return contactMessage
*/
ContactMessage getContactMessage();
/**
* The time Conversation API processed the message.
*
* @return acceptTime
* @readOnly This field is returned by the server and cannot be modified
*/
Instant getAcceptTime();
/**
* Get channelIdentity
*
* @return channelIdentity
*/
ChannelIdentity getChannelIdentity();
/**
* The ID of the contact.
*
* @return contactId
*/
String getContactId();
/**
* The ID of the conversation.
*
* @return conversationId
*/
String getConversationId();
/**
* Get direction
*
* @return direction
*/
ConversationDirection getDirection();
/**
* The ID of the message.
*
* @return id
*/
String getId();
/**
* Optional. Metadata associated with the contact. Up to 1024 characters long.
*
* @return metadata
*/
String getMetadata();
/**
* Flag for whether this message was injected.
*
* @return injected
* @readOnly This field is returned by the server and cannot be modified
*/
Boolean getInjected();
/**
* For Contact Messages the sender ID is the contact sent the message to. For App Messages the
* sender that was used to send the message, if applicable.
*
* @return senderId
*/
String getSenderId();
/**
* Get processingMode
*
* @return processingMode
*/
ProcessingMode getProcessingMode();
/**
* Getting builder
*
* @return New Builder instance
*/
static Builder builder() {
return new ConversationMessageInternalImpl.Builder();
}
/** Dedicated Builder */
interface Builder {
/**
* see getter
*
* @param appMessage see getter
* @return Current builder
* @see #getAppMessage
*/
Builder setAppMessage(AppMessage> appMessage);
/**
* see getter
*
* @param contactMessage see getter
* @return Current builder
* @see #getContactMessage
*/
Builder setContactMessage(ContactMessage contactMessage);
/**
* see getter
*
* @param acceptTime see getter
* @return Current builder
* @see #getAcceptTime
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setAcceptTime(Instant acceptTime);
/**
* see getter
*
* @param channelIdentity see getter
* @return Current builder
* @see #getChannelIdentity
*/
Builder setChannelIdentity(ChannelIdentity channelIdentity);
/**
* see getter
*
* @param contactId see getter
* @return Current builder
* @see #getContactId
*/
Builder setContactId(String contactId);
/**
* see getter
*
* @param conversationId see getter
* @return Current builder
* @see #getConversationId
*/
Builder setConversationId(String conversationId);
/**
* see getter
*
* @param direction see getter
* @return Current builder
* @see #getDirection
*/
Builder setDirection(ConversationDirection direction);
/**
* see getter
*
* @param id see getter
* @return Current builder
* @see #getId
*/
Builder setId(String id);
/**
* see getter
*
* @param metadata see getter
* @return Current builder
* @see #getMetadata
*/
Builder setMetadata(String metadata);
/**
* see getter
*
* @param injected see getter
* @return Current builder
* @see #getInjected
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setInjected(Boolean injected);
/**
* see getter
*
* @param senderId see getter
* @return Current builder
* @see #getSenderId
*/
Builder setSenderId(String senderId);
/**
* see getter
*
* @param processingMode see getter
* @return Current builder
* @see #getProcessingMode
*/
Builder setProcessingMode(ProcessingMode processingMode);
/**
* Create instance
*
* @return The instance build with current builder values
*/
ConversationMessageInternal build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy