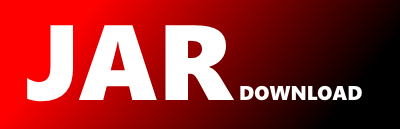
com.sinch.sdk.domains.numbers.api.v1.internal.ActiveNumberApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Numbers | Sinch
*
* OpenAPI document version: 1.0.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.numbers.api.v1.internal;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.numbers.models.v1.ActiveNumber;
import com.sinch.sdk.domains.numbers.models.v1.request.ActiveNumberUpdateRequest;
import com.sinch.sdk.domains.numbers.models.v1.response.internal.ActiveNumberListResponseInternal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class ActiveNumberApi {
private static final Logger LOGGER = Logger.getLogger(ActiveNumberApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public ActiveNumberApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Get active Number Get a virtual number
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param phoneNumber Output only. The phone number in
* [E.164](https://community.sinch.com/t5/Glossary/E-164/ta-p/7537\") format with leading
* `+`. (required)
* @return ActiveNumber
* @throws ApiException if fails to make API call
*/
public ActiveNumber numberServiceGetActiveNumber(String projectId, String phoneNumber)
throws ApiException {
LOGGER.finest(
"[numberServiceGetActiveNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "phoneNumber: "
+ phoneNumber);
HttpRequest httpRequest = numberServiceGetActiveNumberRequestBuilder(projectId, phoneNumber);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceGetActiveNumberRequestBuilder(
String projectId, String phoneNumber) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling numberServiceGetActiveNumber");
}
// verify the required parameter 'phoneNumber' is set
if (phoneNumber == null) {
throw new ApiException(
400,
"Missing the required parameter 'phoneNumber' when calling numberServiceGetActiveNumber");
}
String localVarPath =
"/v1/projects/{projectId}/activeNumbers/{phoneNumber}"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "phoneNumber" + "\\}",
URLPathUtils.encodePathSegment(phoneNumber.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* List active numbers Lists all virtual numbers for a project.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param regionCode Region code to filter by. ISO 3166-1 alpha-2 country code of the phone
* number. Example: `US`, `GB` or `SE`. (required)
* @param type Number type to filter by. Options include, `MOBILE`, `LOCAL` or
* `TOLL_FREE`. (required)
* @param numberPatternPattern Sequence of digits to search for. If you prefer or need certain
* digits in sequential order, you can enter the sequence of numbers here. For example,
* `2020`. (optional)
* @param numberPatternSearchPattern Search pattern to apply. The options are, `START`,
* `CONTAIN`, and `END`. (optional)
* @param capability Number capabilities to filter by, `SMS` and/or `VOICE`.
* (optional
* @param pageSize The maximum number of items to return. (optional)
* @param pageToken The next page token value returned from a previous List request, if any.
* (optional)
* @param orderBy Supported fields for ordering by `phoneNumber` or
* `displayName`. (optional)
* @return ActiveNumberListResponseInternal
* @throws ApiException if fails to make API call
*/
public ActiveNumberListResponseInternal numberServiceListActiveNumbers(
String projectId,
String regionCode,
String type,
String numberPatternPattern,
String numberPatternSearchPattern,
List capability,
Integer pageSize,
String pageToken,
String orderBy)
throws ApiException {
LOGGER.finest(
"[numberServiceListActiveNumbers]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "regionCode: "
+ regionCode
+ ", "
+ "type: "
+ type
+ ", "
+ "numberPatternPattern: "
+ numberPatternPattern
+ ", "
+ "numberPatternSearchPattern: "
+ numberPatternSearchPattern
+ ", "
+ "capability: "
+ capability
+ ", "
+ "pageSize: "
+ pageSize
+ ", "
+ "pageToken: "
+ pageToken
+ ", "
+ "orderBy: "
+ orderBy);
HttpRequest httpRequest =
numberServiceListActiveNumbersRequestBuilder(
projectId,
regionCode,
type,
numberPatternPattern,
numberPatternSearchPattern,
capability,
pageSize,
pageToken,
orderBy);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceListActiveNumbersRequestBuilder(
String projectId,
String regionCode,
String type,
String numberPatternPattern,
String numberPatternSearchPattern,
List capability,
Integer pageSize,
String pageToken,
String orderBy)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling numberServiceListActiveNumbers");
}
// verify the required parameter 'regionCode' is set
if (regionCode == null) {
throw new ApiException(
400,
"Missing the required parameter 'regionCode' when calling"
+ " numberServiceListActiveNumbers");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(
400, "Missing the required parameter 'type' when calling numberServiceListActiveNumbers");
}
String localVarPath =
"/v1/projects/{projectId}/activeNumbers"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != regionCode) {
localVarQueryParams.add(
new URLParameter(
"regionCode", regionCode, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != numberPatternPattern) {
localVarQueryParams.add(
new URLParameter(
"numberPattern.pattern",
numberPatternPattern,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != numberPatternSearchPattern) {
localVarQueryParams.add(
new URLParameter(
"numberPattern.searchPattern",
numberPatternSearchPattern,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != type) {
localVarQueryParams.add(
new URLParameter("type", type, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != capability) {
localVarQueryParams.add(
new URLParameter(
"capability", capability, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != pageSize) {
localVarQueryParams.add(
new URLParameter(
"pageSize", pageSize, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != pageToken) {
localVarQueryParams.add(
new URLParameter(
"pageToken", pageToken, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != orderBy) {
localVarQueryParams.add(
new URLParameter(
"orderBy", orderBy, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Release number With this endpoint, you can cancel your subscription for a specific virtual
* phone number.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param phoneNumber Output only. The phone number in
* [E.164](https://community.sinch.com/t5/Glossary/E-164/ta-p/7537\") format with leading
* `+`. (required)
* @return ActiveNumber
* @throws ApiException if fails to make API call
*/
public ActiveNumber numberServiceReleaseNumber(String projectId, String phoneNumber)
throws ApiException {
LOGGER.finest(
"[numberServiceReleaseNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "phoneNumber: "
+ phoneNumber);
HttpRequest httpRequest = numberServiceReleaseNumberRequestBuilder(projectId, phoneNumber);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceReleaseNumberRequestBuilder(String projectId, String phoneNumber)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling numberServiceReleaseNumber");
}
// verify the required parameter 'phoneNumber' is set
if (phoneNumber == null) {
throw new ApiException(
400,
"Missing the required parameter 'phoneNumber' when calling numberServiceReleaseNumber");
}
String localVarPath =
"/v1/projects/{projectId}/activeNumbers/{phoneNumber}:release"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "phoneNumber" + "\\}",
URLPathUtils.encodePathSegment(phoneNumber.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Update active number Update a virtual phone number. For example: you can configure SMS/Voice
* services or set a friendly name. To update the name that displays, modify the
* `displayName` parameter. You'll use `smsConfiguration` to update your
* SMS configuration and `voiceConfiguration` to update the voice configuration.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param phoneNumber Output only. The phone number in
* [E.164](https://community.sinch.com/t5/Glossary/E-164/ta-p/7537\") format with leading
* `+`. (required)
* @param activeNumberUpdateRequest The number body to be updated. (optional)
* @return ActiveNumber
* @throws ApiException if fails to make API call
*/
public ActiveNumber numberServiceUpdateActiveNumber(
String projectId, String phoneNumber, ActiveNumberUpdateRequest activeNumberUpdateRequest)
throws ApiException {
LOGGER.finest(
"[numberServiceUpdateActiveNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "phoneNumber: "
+ phoneNumber
+ ", "
+ "activeNumberUpdateRequest: "
+ activeNumberUpdateRequest);
HttpRequest httpRequest =
numberServiceUpdateActiveNumberRequestBuilder(
projectId, phoneNumber, activeNumberUpdateRequest);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceUpdateActiveNumberRequestBuilder(
String projectId, String phoneNumber, ActiveNumberUpdateRequest activeNumberUpdateRequest)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling"
+ " numberServiceUpdateActiveNumber");
}
// verify the required parameter 'phoneNumber' is set
if (phoneNumber == null) {
throw new ApiException(
400,
"Missing the required parameter 'phoneNumber' when calling"
+ " numberServiceUpdateActiveNumber");
}
String localVarPath =
"/v1/projects/{projectId}/activeNumbers/{phoneNumber}"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "phoneNumber" + "\\}",
URLPathUtils.encodePathSegment(phoneNumber.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = mapper.serialize(localVarContentTypes, activeNumberUpdateRequest);
return new HttpRequest(
localVarPath,
HttpMethod.PATCH,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy