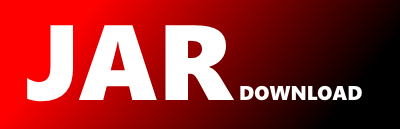
com.sinch.sdk.domains.numbers.api.v1.internal.AvailableNumberApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Numbers | Sinch
*
* OpenAPI document version: 1.0.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.numbers.api.v1.internal;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.numbers.models.v1.ActiveNumber;
import com.sinch.sdk.domains.numbers.models.v1.request.AvailableNumberRentAnyRequest;
import com.sinch.sdk.domains.numbers.models.v1.request.AvailableNumberRentRequest;
import com.sinch.sdk.domains.numbers.models.v1.response.AvailableNumber;
import com.sinch.sdk.domains.numbers.models.v1.response.internal.AvailableNumberListResponseInternal;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class AvailableNumberApi {
private static final Logger LOGGER = Logger.getLogger(AvailableNumberApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public AvailableNumberApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* Get available number This endpoint allows you to enter a specific phone number to check if
* it's available for use. A 200 response will return the number's capability, setup
* costs, monthly costs and if supporting documentation is required.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param phoneNumber Output only. The phone number in
* [E.164](https://community.sinch.com/t5/Glossary/E-164/ta-p/7537) format with leading
* `+`. (required)
* @return AvailableNumber
* @throws ApiException if fails to make API call
*/
public AvailableNumber numberServiceGetAvailableNumber(String projectId, String phoneNumber)
throws ApiException {
LOGGER.finest(
"[numberServiceGetAvailableNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "phoneNumber: "
+ phoneNumber);
HttpRequest httpRequest = numberServiceGetAvailableNumberRequestBuilder(projectId, phoneNumber);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceGetAvailableNumberRequestBuilder(
String projectId, String phoneNumber) throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling"
+ " numberServiceGetAvailableNumber");
}
// verify the required parameter 'phoneNumber' is set
if (phoneNumber == null) {
throw new ApiException(
400,
"Missing the required parameter 'phoneNumber' when calling"
+ " numberServiceGetAvailableNumber");
}
String localVarPath =
"/v1/projects/{projectId}/availableNumbers/{phoneNumber}"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "phoneNumber" + "\\}",
URLPathUtils.encodePathSegment(phoneNumber.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* List available numbers Search for virtual numbers that are available for you to activate. You
* can filter by any property on the available number resource.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param regionCode Region code to filter by. ISO 3166-1 alpha-2 country code of the phone
* number. Example: `US`, `GB` or `SE`. (required)
* @param type Number type to filter by. Options include, `MOBILE`, `LOCAL` or
* `TOLL_FREE`. (required)
* @param numberPatternPattern Sequence of digits to search for. If you prefer or need certain
* digits in sequential order, you can enter the sequence of numbers here. For example,
* `2020`. (optional)
* @param numberPatternSearchPattern Search pattern to apply. The options are, `START`,
* `CONTAIN`, and `END`. (optional)
* @param capabilities Number capabilities to filter by SMS and/or VOICE. When searching, indicate
* the `capabilities` of the number as `SMS` and/or `VOICE`. To
* search for a number capable of both, list both `SMS` and `VOICE`.
* (optional
* @param size Optional. The maximum number of items to return. (optional)
* @return AvailableNumberListResponseInternal
* @throws ApiException if fails to make API call
*/
public AvailableNumberListResponseInternal numberServiceListAvailableNumbers(
String projectId,
String regionCode,
String type,
String numberPatternPattern,
String numberPatternSearchPattern,
List capabilities,
Integer size)
throws ApiException {
LOGGER.finest(
"[numberServiceListAvailableNumbers]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "regionCode: "
+ regionCode
+ ", "
+ "type: "
+ type
+ ", "
+ "numberPatternPattern: "
+ numberPatternPattern
+ ", "
+ "numberPatternSearchPattern: "
+ numberPatternSearchPattern
+ ", "
+ "capabilities: "
+ capabilities
+ ", "
+ "size: "
+ size);
HttpRequest httpRequest =
numberServiceListAvailableNumbersRequestBuilder(
projectId,
regionCode,
type,
numberPatternPattern,
numberPatternSearchPattern,
capabilities,
size);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceListAvailableNumbersRequestBuilder(
String projectId,
String regionCode,
String type,
String numberPatternPattern,
String numberPatternSearchPattern,
List capabilities,
Integer size)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling"
+ " numberServiceListAvailableNumbers");
}
// verify the required parameter 'regionCode' is set
if (regionCode == null) {
throw new ApiException(
400,
"Missing the required parameter 'regionCode' when calling"
+ " numberServiceListAvailableNumbers");
}
// verify the required parameter 'type' is set
if (type == null) {
throw new ApiException(
400,
"Missing the required parameter 'type' when calling numberServiceListAvailableNumbers");
}
String localVarPath =
"/v1/projects/{projectId}/availableNumbers"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != numberPatternPattern) {
localVarQueryParams.add(
new URLParameter(
"numberPattern.pattern",
numberPatternPattern,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != numberPatternSearchPattern) {
localVarQueryParams.add(
new URLParameter(
"numberPattern.searchPattern",
numberPatternSearchPattern,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != regionCode) {
localVarQueryParams.add(
new URLParameter(
"regionCode", regionCode, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != type) {
localVarQueryParams.add(
new URLParameter("type", type, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != capabilities) {
localVarQueryParams.add(
new URLParameter(
"capabilities",
capabilities,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
if (null != size) {
localVarQueryParams.add(
new URLParameter("size", size, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Rent any number that matches the criteria Search for and activate an available Sinch virtual
* number all in one API call. Currently the rentAny operation works only for US 10DLC numbers
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param availableNumberRentAnyRequest The request to search and rent a number that matches the
* criteria. (required)
* @return ActiveNumber
* @throws ApiException if fails to make API call
*/
public ActiveNumber numberServiceRentAnyNumber(
String projectId, AvailableNumberRentAnyRequest availableNumberRentAnyRequest)
throws ApiException {
LOGGER.finest(
"[numberServiceRentAnyNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "availableNumberRentAnyRequest: "
+ availableNumberRentAnyRequest);
HttpRequest httpRequest =
numberServiceRentAnyNumberRequestBuilder(projectId, availableNumberRentAnyRequest);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceRentAnyNumberRequestBuilder(
String projectId, AvailableNumberRentAnyRequest availableNumberRentAnyRequest)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400,
"Missing the required parameter 'projectId' when calling numberServiceRentAnyNumber");
}
// verify the required parameter 'availableNumberRentAnyRequest' is set
if (availableNumberRentAnyRequest == null) {
throw new ApiException(
400,
"Missing the required parameter 'availableNumberRentAnyRequest' when calling"
+ " numberServiceRentAnyNumber");
}
String localVarPath =
"/v1/projects/{projectId}/availableNumbers:rentAny"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody =
mapper.serialize(localVarContentTypes, availableNumberRentAnyRequest);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Rent an available number Activate a virtual number to use with SMS products, Voice products, or
* both. You'll use `smsConfiguration` to setup your number for SMS and
* `voiceConfiguration` for Voice.
*
* @param projectId Found on your [Sinch Customer
* Dashboard](https://dashboard.sinch.com/settings/project-management). (required)
* @param phoneNumber Output only. The phone number in
* [E.164](https://community.sinch.com/t5/Glossary/E-164/ta-p/7537) format with leading
* `+`. (required)
* @param availableNumberRentRequest The request to rent a number. (required)
* @return ActiveNumber
* @throws ApiException if fails to make API call
*/
public ActiveNumber numberServiceRentNumber(
String projectId, String phoneNumber, AvailableNumberRentRequest availableNumberRentRequest)
throws ApiException {
LOGGER.finest(
"[numberServiceRentNumber]"
+ " "
+ "projectId: "
+ projectId
+ ", "
+ "phoneNumber: "
+ phoneNumber
+ ", "
+ "availableNumberRentRequest: "
+ availableNumberRentRequest);
HttpRequest httpRequest =
numberServiceRentNumberRequestBuilder(projectId, phoneNumber, availableNumberRentRequest);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest numberServiceRentNumberRequestBuilder(
String projectId, String phoneNumber, AvailableNumberRentRequest availableNumberRentRequest)
throws ApiException {
// verify the required parameter 'projectId' is set
if (projectId == null) {
throw new ApiException(
400, "Missing the required parameter 'projectId' when calling numberServiceRentNumber");
}
// verify the required parameter 'phoneNumber' is set
if (phoneNumber == null) {
throw new ApiException(
400, "Missing the required parameter 'phoneNumber' when calling numberServiceRentNumber");
}
// verify the required parameter 'availableNumberRentRequest' is set
if (availableNumberRentRequest == null) {
throw new ApiException(
400,
"Missing the required parameter 'availableNumberRentRequest' when calling"
+ " numberServiceRentNumber");
}
String localVarPath =
"/v1/projects/{projectId}/availableNumbers/{phoneNumber}:rent"
.replaceAll(
"\\{" + "projectId" + "\\}", URLPathUtils.encodePathSegment(projectId.toString()))
.replaceAll(
"\\{" + "phoneNumber" + "\\}",
URLPathUtils.encodePathSegment(phoneNumber.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList("application/json");
final Collection localVarAuthNames = Arrays.asList("BasicAuth");
final String serializedBody =
mapper.serialize(localVarContentTypes, availableNumberRentRequest);
return new HttpRequest(
localVarPath,
HttpMethod.POST,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy