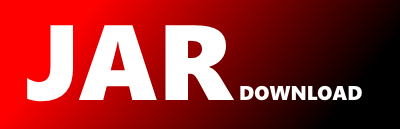
com.sinch.sdk.domains.numbers.models.v1.response.AvailableNumber Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* Numbers | Sinch
*
* OpenAPI document version: 1.0.2
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.numbers.models.v1.response;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.sinch.sdk.domains.numbers.models.v1.Capability;
import com.sinch.sdk.domains.numbers.models.v1.Money;
import com.sinch.sdk.domains.numbers.models.v1.NumberType;
import java.util.List;
/**
* The phone numbers that are available to be rented in the Sinch Customer Dashboard or via the
* public numbers API.
*/
@JsonDeserialize(builder = AvailableNumberImpl.Builder.class)
public interface AvailableNumber {
/**
* The phone number in E.164 format with
* leading +
.
*
* @return phoneNumber
* @readOnly This field is returned by the server and cannot be modified
*/
String getPhoneNumber();
/**
* ISO 3166-1 alpha-2 country code of the phone number. Example: US
, GB
* or SE
.
*
* @return regionCode
* @readOnly This field is returned by the server and cannot be modified
*/
String getRegionCode();
/**
* Get type
*
* @return type
*/
NumberType getType();
/**
* The capability of the number.
*
* @return capability
*/
List getCapability();
/**
* Get setupPrice
*
* @return setupPrice
*/
Money getSetupPrice();
/**
* Get monthlyPrice
*
* @return monthlyPrice
*/
Money getMonthlyPrice();
/**
* How often the recurring price is charged in months.
*
* @return paymentIntervalMonths
* @readOnly This field is returned by the server and cannot be modified
*/
Integer getPaymentIntervalMonths();
/**
* Whether or not supplementary documentation will be required to complete the number rental.
*
* @return supportingDocumentationRequired
* @readOnly This field is returned by the server and cannot be modified
*/
Boolean getSupportingDocumentationRequired();
/**
* Getting builder
*
* @return New Builder instance
*/
static Builder builder() {
return new AvailableNumberImpl.Builder();
}
/** Dedicated Builder */
interface Builder {
/**
* see getter
*
* @param phoneNumber see getter
* @return Current builder
* @see #getPhoneNumber
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setPhoneNumber(String phoneNumber);
/**
* see getter
*
* @param regionCode see getter
* @return Current builder
* @see #getRegionCode
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setRegionCode(String regionCode);
/**
* see getter
*
* @param type see getter
* @return Current builder
* @see #getType
*/
Builder setType(NumberType type);
/**
* see getter
*
* @param capability see getter
* @return Current builder
* @see #getCapability
*/
Builder setCapability(List capability);
/**
* see getter
*
* @param setupPrice see getter
* @return Current builder
* @see #getSetupPrice
*/
Builder setSetupPrice(Money setupPrice);
/**
* see getter
*
* @param monthlyPrice see getter
* @return Current builder
* @see #getMonthlyPrice
*/
Builder setMonthlyPrice(Money monthlyPrice);
/**
* see getter
*
* @param paymentIntervalMonths see getter
* @return Current builder
* @see #getPaymentIntervalMonths
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setPaymentIntervalMonths(Integer paymentIntervalMonths);
/**
* see getter
*
* @param supportingDocumentationRequired see getter
* @return Current builder
* @see #getSupportingDocumentationRequired
* @readOnly This field is returned by the server and cannot be modified
*/
Builder setSupportingDocumentationRequired(Boolean supportingDocumentationRequired);
/**
* Create instance
*
* @return The instance build with current builder values
*/
AvailableNumber build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy