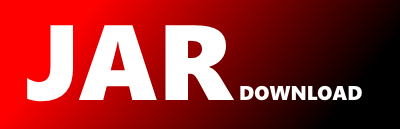
com.sinch.sdk.domains.sms.GroupsService Maven / Gradle / Ivy
Show all versions of sinch-sdk-java Show documentation
package com.sinch.sdk.domains.sms;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.domains.sms.models.Group;
import com.sinch.sdk.domains.sms.models.requests.GroupCreateRequestParameters;
import com.sinch.sdk.domains.sms.models.requests.GroupReplaceRequestParameters;
import com.sinch.sdk.domains.sms.models.requests.GroupUpdateRequestParameters;
import com.sinch.sdk.domains.sms.models.requests.GroupsListRequestParameters;
import com.sinch.sdk.domains.sms.models.responses.GroupsListResponse;
import java.util.Collection;
/**
* Groups Service
*
* A group is a set of phone numbers (or MSISDNs) that can be used as a target when sending an
* SMS. An phone number (MSISDN) can only occur once in a group and any attempts to add a duplicate
* are ignored but not rejected.
*
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups
* @since 1.0
*/
public interface GroupsService {
/**
* Retrieve a group.
*
*
This operation retrieves a specific group with the provided group ID.
*
* @param groupId The inbound ID found when listing inbound messages
* @return Group associated to groupId
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/RetrieveGroup
* @since 1.0
*/
Group get(String groupId) throws ApiException;
/**
* Create a group.
*
*
A group is a set of phone numbers (MSISDNs) that can be used as a target in the
* send_batch_msg
operation. An MSISDN can only occur once in a group and any attempts to
* add a duplicate would be ignored but not rejected.
*
* @param parameters Parameters to be used to define group onto creation
* @return Created group
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/CreateGroup
* @since 1.0
*/
Group create(GroupCreateRequestParameters parameters) throws ApiException;
/**
* Create an unnamed and empty group
*
* @return See {@link #create(GroupCreateRequestParameters)}
* @since 1.0
*/
Group create() throws ApiException;
/**
* List Groups
*
*
With the list operation you can list all groups that you have created. This operation
* supports pagination.
*
*
Groups are returned in reverse chronological order.
*
* @param parameters Filtering parameters
* @return group list
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/ListGroups
* @since 1.0
*/
GroupsListResponse list(GroupsListRequestParameters parameters) throws ApiException;
/**
* List groups with default parameters
*
* @return See {@link #list(GroupsListRequestParameters)}
* @since 1.0
*/
GroupsListResponse list() throws ApiException;
/**
* Replace a group
*
*
The replace operation will replace all parameters, including members, of an existing group
* with new values.
*
*
Replacing a group targeted by a batch message scheduled in the future is allowed and changes
* will be reflected when the batch is sent.
*
* @param groupId ID of a group that you are interested in getting.
* @param parameters Parameters to be replaced for group
* @return Group associated to groupId
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/ReplaceGroup
* @since 1.0
*/
Group replace(String groupId, GroupReplaceRequestParameters parameters) throws ApiException;
/**
* Update a group
*
* @param groupId ID of a group that you are interested in getting.
* @param parameters Parameters to be used to update group
* @return Modified group associated to groupId
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/UpdateGroup
* @since 1.0
*/
Group update(String groupId, GroupUpdateRequestParameters parameters) throws ApiException;
/**
* Delete a group
*
* @param groupId ID of a group that you are interested in getting.
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/deleteGroup
* @since 1.0
*/
void delete(String groupId) throws ApiException;
/**
* Get phone numbers for a group
*
* @param groupId ID of a group that you are interested in getting.
* @return A list of phone numbers in E.164 format.
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/deleteGroup
* @since 1.0
*/
Collection listMembers(String groupId) throws ApiException;
}