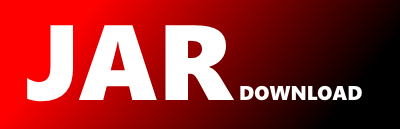
com.sinch.sdk.domains.sms.adapters.api.v1.InboundsApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.adapters.api.v1;
import com.fasterxml.jackson.core.type.TypeReference;
import com.sinch.sdk.core.exceptions.ApiException;
import com.sinch.sdk.core.exceptions.ApiExceptionBuilder;
import com.sinch.sdk.core.http.AuthManager;
import com.sinch.sdk.core.http.HttpClient;
import com.sinch.sdk.core.http.HttpMapper;
import com.sinch.sdk.core.http.HttpMethod;
import com.sinch.sdk.core.http.HttpRequest;
import com.sinch.sdk.core.http.HttpResponse;
import com.sinch.sdk.core.http.HttpStatus;
import com.sinch.sdk.core.http.URLParameter;
import com.sinch.sdk.core.http.URLPathUtils;
import com.sinch.sdk.core.models.ServerConfiguration;
import com.sinch.sdk.domains.sms.models.dto.v1.ApiInboundListDto;
import com.sinch.sdk.domains.sms.models.dto.v1.InboundDto;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Logger;
public class InboundsApi {
private static final Logger LOGGER = Logger.getLogger(InboundsApi.class.getName());
private HttpClient httpClient;
private ServerConfiguration serverConfiguration;
private Map authManagersByOasSecuritySchemes;
private HttpMapper mapper;
public InboundsApi(
HttpClient httpClient,
ServerConfiguration serverConfiguration,
Map authManagersByOasSecuritySchemes,
HttpMapper mapper) {
this.httpClient = httpClient;
this.serverConfiguration = serverConfiguration;
this.authManagersByOasSecuritySchemes = authManagersByOasSecuritySchemes;
this.mapper = mapper;
}
/**
* List incoming messages With the list operation, you can list all inbound messages that you have
* received. This operation supports pagination. Inbounds are returned in reverse chronological
* order.
*
* @param servicePlanId Your service plan ID. You can find this on your
* [Dashboard](https://dashboard.sinch.com/sms/api/rest). (required)
* @param page The page number starting from 0. (optional, default to 0)
* @param pageSize Determines the size of a page (optional, default to 30)
* @param to Only list messages sent to this destination. Multiple phone numbers formatted as
* either <a
* href=\"https://community.sinch.com/t5/Glossary/E-164/ta-p/7537\"
* target=\"_blank\">E.164</a> or short codes can be comma separated.
* (optional)
* @param startDate Only list messages received at or after this date/time. Formatted as
* [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601): `YYYY-MM-DDThh:mm:ss.SSSZ`.
* Default: Now-24 (optional, default to Now-24)
* @param endDate Only list messages received before this date/time. Formatted as
* [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601): `YYYY-MM-DDThh:mm:ss.SSSZ`.
* (optional)
* @param clientReference Using a client reference in inbound messages requires additional setup
* on your account. Contact your <a
* href=\"https://dashboard.sinch.com/settings/account-details\"
* target=\"_blank\">account manager</a> to enable this feature. Only
* list inbound messages that are in response to messages with a previously provided client
* reference. (optional)
* @return ApiInboundListDto
* @throws ApiException if fails to make API call
*/
public ApiInboundListDto listInboundMessages(
String servicePlanId,
Integer page,
Integer pageSize,
String to,
String startDate,
String endDate,
String clientReference)
throws ApiException {
LOGGER.finest(
"[listInboundMessages]"
+ " "
+ "servicePlanId: "
+ servicePlanId
+ ", "
+ "page: "
+ page
+ ", "
+ "pageSize: "
+ pageSize
+ ", "
+ "to: "
+ to
+ ", "
+ "startDate: "
+ startDate
+ ", "
+ "endDate: "
+ endDate
+ ", "
+ "clientReference: "
+ clientReference);
HttpRequest httpRequest =
listInboundMessagesRequestBuilder(
servicePlanId, page, pageSize, to, startDate, endDate, clientReference);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType =
new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest listInboundMessagesRequestBuilder(
String servicePlanId,
Integer page,
Integer pageSize,
String to,
String startDate,
String endDate,
String clientReference)
throws ApiException {
// verify the required parameter 'servicePlanId' is set
if (servicePlanId == null) {
throw new ApiException(
400, "Missing the required parameter 'servicePlanId' when calling listInboundMessages");
}
String localVarPath =
"/xms/v1/{service_plan_id}/inbounds"
.replaceAll(
"\\{" + "service_plan_id" + "\\}",
URLPathUtils.encodePathSegment(servicePlanId.toString()));
List localVarQueryParams = new ArrayList<>();
if (null != page) {
localVarQueryParams.add(
new URLParameter("page", page, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != pageSize) {
localVarQueryParams.add(
new URLParameter(
"page_size", pageSize, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != to) {
localVarQueryParams.add(
new URLParameter("to", to, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != startDate) {
localVarQueryParams.add(
new URLParameter(
"start_date", startDate, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != endDate) {
localVarQueryParams.add(
new URLParameter(
"end_date", endDate, URLParameter.STYLE.valueOf("form".toUpperCase()), true));
}
if (null != clientReference) {
localVarQueryParams.add(
new URLParameter(
"client_reference",
clientReference,
URLParameter.STYLE.valueOf("form".toUpperCase()),
true));
}
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BearerAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
/**
* Retrieve inbound message This operation retrieves a specific inbound message with the provided
* inbound ID.
*
* @param servicePlanId Your service plan ID. You can find this on your
* [Dashboard](https://dashboard.sinch.com/sms/api/rest). (required)
* @param inboundId The inbound ID found when listing inbound messages. (required)
* @return InboundDto
* @throws ApiException if fails to make API call
*/
public InboundDto retrieveInboundMessage(String servicePlanId, String inboundId)
throws ApiException {
LOGGER.finest(
"[retrieveInboundMessage]"
+ " "
+ "servicePlanId: "
+ servicePlanId
+ ", "
+ "inboundId: "
+ inboundId);
HttpRequest httpRequest = retrieveInboundMessageRequestBuilder(servicePlanId, inboundId);
HttpResponse response =
httpClient.invokeAPI(
this.serverConfiguration, this.authManagersByOasSecuritySchemes, httpRequest);
if (HttpStatus.isSuccessfulStatus(response.getCode())) {
TypeReference localVarReturnType = new TypeReference() {};
return mapper.deserialize(response, localVarReturnType);
}
// fallback to default errors handling:
// all error cases definition are not required from specs: will try some "hardcoded" content
// parsing
throw ApiExceptionBuilder.build(
response.getMessage(),
response.getCode(),
mapper.deserialize(response, new TypeReference>() {}));
}
private HttpRequest retrieveInboundMessageRequestBuilder(String servicePlanId, String inboundId)
throws ApiException {
// verify the required parameter 'servicePlanId' is set
if (servicePlanId == null) {
throw new ApiException(
400,
"Missing the required parameter 'servicePlanId' when calling retrieveInboundMessage");
}
// verify the required parameter 'inboundId' is set
if (inboundId == null) {
throw new ApiException(
400, "Missing the required parameter 'inboundId' when calling retrieveInboundMessage");
}
String localVarPath =
"/xms/v1/{service_plan_id}/inbounds/{inbound_id}"
.replaceAll(
"\\{" + "service_plan_id" + "\\}",
URLPathUtils.encodePathSegment(servicePlanId.toString()))
.replaceAll(
"\\{" + "inbound_id" + "\\}", URLPathUtils.encodePathSegment(inboundId.toString()));
List localVarQueryParams = new ArrayList<>();
Map localVarHeaderParams = new HashMap<>();
final Collection localVarAccepts = Arrays.asList("application/json");
final Collection localVarContentTypes = Arrays.asList();
final Collection localVarAuthNames = Arrays.asList("BearerAuth");
final String serializedBody = null;
return new HttpRequest(
localVarPath,
HttpMethod.GET,
localVarQueryParams,
serializedBody,
localVarHeaderParams,
localVarAccepts,
localVarContentTypes,
localVarAuthNames);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy