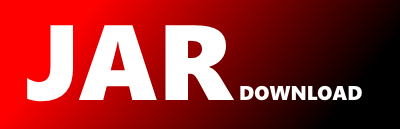
com.sinch.sdk.domains.sms.models.DeliveryReportBatch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.sms.models;
import java.util.Collection;
import java.util.Objects;
/**
* Common Definition to Delivery Report
*
* @since 1.0
*/
public abstract class DeliveryReportBatch extends BaseDeliveryReport {
private final Collection statuses;
private final Integer totalMessageCount;
/**
* @param batchId Required.
The ID of the batch this delivery report belongs to.
* @param clientReference The client identifier of the batch this delivery report belongs to, if
* set when submitting batch.
* @param statuses Required.
Array with status objects. Only status codes with at
* least one recipient will be listed.
* @param totalMessageCount Required.
The total number of messages in the batch.
*/
public DeliveryReportBatch(
String batchId,
String clientReference,
Collection statuses,
Integer totalMessageCount) {
super(batchId, clientReference);
Objects.requireNonNull(statuses);
Objects.requireNonNull(totalMessageCount);
this.statuses = statuses;
this.totalMessageCount = totalMessageCount;
}
public Collection getStatuses() {
return statuses;
}
public Integer getTotalMessageCount() {
return totalMessageCount;
}
@Override
public String toString() {
return "DeliveryReportBatch{"
+ "statuses="
+ statuses
+ ", totalMessageCount="
+ totalMessageCount
+ "} "
+ super.toString();
}
public abstract static class Builder> extends BaseDeliveryReport.Builder {
protected Collection statuses;
protected Integer totalMessageCount;
public B setStatuses(Collection statuses) {
this.statuses = statuses;
return self();
}
public B setTotalMessageCount(Integer totalMessageCount) {
this.totalMessageCount = totalMessageCount;
return self();
}
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
public abstract DeliveryReportBatch build();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy