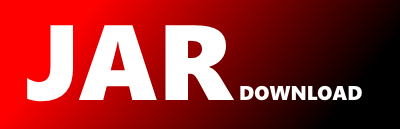
com.sinch.sdk.domains.sms.models.dto.v1.ApiUpdateMmsMtMessageDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** ApiUpdateMmsMtMessageDto */
@JsonPropertyOrder({
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_FROM,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_TYPE,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_TO_ADD,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_TO_REMOVE,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_DELIVERY_REPORT,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_SEND_AT,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_EXPIRE_AT,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_CALLBACK_URL,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_BODY,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_PARAMETERS,
ApiUpdateMmsMtMessageDto.JSON_PROPERTY_STRICT_VALIDATION
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class ApiUpdateMmsMtMessageDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_FROM = "from";
private String from;
private boolean fromDefined = false;
/** MMS */
public enum TypeEnum {
MT_MEDIA("mt_media"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
private boolean typeDefined = false;
public static final String JSON_PROPERTY_TO_ADD = "to_add";
private List toAdd;
private boolean toAddDefined = false;
public static final String JSON_PROPERTY_TO_REMOVE = "to_remove";
private List toRemove;
private boolean toRemoveDefined = false;
public static final String JSON_PROPERTY_DELIVERY_REPORT = "delivery_report";
private String deliveryReport;
private boolean deliveryReportDefined = false;
public static final String JSON_PROPERTY_SEND_AT = "send_at";
private OffsetDateTime sendAt;
private boolean sendAtDefined = false;
public static final String JSON_PROPERTY_EXPIRE_AT = "expire_at";
private OffsetDateTime expireAt;
private boolean expireAtDefined = false;
public static final String JSON_PROPERTY_CALLBACK_URL = "callback_url";
private String callbackUrl;
private boolean callbackUrlDefined = false;
public static final String JSON_PROPERTY_BODY = "body";
private MediaBodyDto body;
private boolean bodyDefined = false;
public static final String JSON_PROPERTY_PARAMETERS = "parameters";
private ParameterObjDto parameters;
private boolean parametersDefined = false;
public static final String JSON_PROPERTY_STRICT_VALIDATION = "strict_validation";
private Boolean strictValidation;
private boolean strictValidationDefined = false;
public ApiUpdateMmsMtMessageDto() {}
public ApiUpdateMmsMtMessageDto from(String from) {
this.from = from;
this.fromDefined = true;
return this;
}
/**
* Sender number. Must be valid phone number, short code or alphanumeric.
*
* @return from
*/
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFrom() {
return from;
}
@JsonIgnore
public boolean getFromDefined() {
return fromDefined;
}
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrom(String from) {
this.from = from;
this.fromDefined = true;
}
public ApiUpdateMmsMtMessageDto type(String type) {
this.type = type;
this.typeDefined = true;
return this;
}
/**
* MMS
*
* @return type
*/
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getType() {
return type;
}
@JsonIgnore
public boolean getTypeDefined() {
return typeDefined;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(String type) {
this.type = type;
this.typeDefined = true;
}
public ApiUpdateMmsMtMessageDto toAdd(List toAdd) {
this.toAdd = toAdd;
this.toAddDefined = true;
return this;
}
public ApiUpdateMmsMtMessageDto addToAddItem(String toAddItem) {
if (this.toAdd == null) {
this.toAdd = new ArrayList<>();
}
this.toAddDefined = true;
this.toAdd.add(toAddItem);
return this;
}
/**
* List of phone numbers and group IDs to add to the batch.
*
* @return toAdd
*/
@JsonProperty(JSON_PROPERTY_TO_ADD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getToAdd() {
return toAdd;
}
@JsonIgnore
public boolean getToAddDefined() {
return toAddDefined;
}
@JsonProperty(JSON_PROPERTY_TO_ADD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setToAdd(List toAdd) {
this.toAdd = toAdd;
this.toAddDefined = true;
}
public ApiUpdateMmsMtMessageDto toRemove(List toRemove) {
this.toRemove = toRemove;
this.toRemoveDefined = true;
return this;
}
public ApiUpdateMmsMtMessageDto addToRemoveItem(String toRemoveItem) {
if (this.toRemove == null) {
this.toRemove = new ArrayList<>();
}
this.toRemoveDefined = true;
this.toRemove.add(toRemoveItem);
return this;
}
/**
* List of phone numbers and group IDs to remove from the batch.
*
* @return toRemove
*/
@JsonProperty(JSON_PROPERTY_TO_REMOVE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getToRemove() {
return toRemove;
}
@JsonIgnore
public boolean getToRemoveDefined() {
return toRemoveDefined;
}
@JsonProperty(JSON_PROPERTY_TO_REMOVE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setToRemove(List toRemove) {
this.toRemove = toRemove;
this.toRemoveDefined = true;
}
public ApiUpdateMmsMtMessageDto deliveryReport(String deliveryReport) {
this.deliveryReport = deliveryReport;
this.deliveryReportDefined = true;
return this;
}
/**
* Request delivery report callback. Note that delivery reports can be fetched from the API
* regardless of this setting.
*
* @return deliveryReport
*/
@JsonProperty(JSON_PROPERTY_DELIVERY_REPORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDeliveryReport() {
return deliveryReport;
}
@JsonIgnore
public boolean getDeliveryReportDefined() {
return deliveryReportDefined;
}
@JsonProperty(JSON_PROPERTY_DELIVERY_REPORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeliveryReport(String deliveryReport) {
this.deliveryReport = deliveryReport;
this.deliveryReportDefined = true;
}
public ApiUpdateMmsMtMessageDto sendAt(OffsetDateTime sendAt) {
this.sendAt = sendAt;
this.sendAtDefined = true;
return this;
}
/**
* If set, in the future the message will be delayed until `send_at` occurs. Formatted
* as <a href=\"https://en.wikipedia.org/wiki/ISO_8601\"
* target=\"_blank\">ISO-8601</a>: `YYYY-MM-DDThh:mm:ss.SSSZ`.
* Constraints: Must be before expire_at. If set in the past, messages will be sent immediately.
*
* @return sendAt
*/
@JsonProperty(JSON_PROPERTY_SEND_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getSendAt() {
return sendAt;
}
@JsonIgnore
public boolean getSendAtDefined() {
return sendAtDefined;
}
@JsonProperty(JSON_PROPERTY_SEND_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSendAt(OffsetDateTime sendAt) {
this.sendAt = sendAt;
this.sendAtDefined = true;
}
public ApiUpdateMmsMtMessageDto expireAt(OffsetDateTime expireAt) {
this.expireAt = expireAt;
this.expireAtDefined = true;
return this;
}
/**
* If set, the system will stop trying to deliver the message at this point. Constraints: Must be
* after `send_at` Default: 3 days after `send_at`
*
* @return expireAt
*/
@JsonProperty(JSON_PROPERTY_EXPIRE_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getExpireAt() {
return expireAt;
}
@JsonIgnore
public boolean getExpireAtDefined() {
return expireAtDefined;
}
@JsonProperty(JSON_PROPERTY_EXPIRE_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExpireAt(OffsetDateTime expireAt) {
this.expireAt = expireAt;
this.expireAtDefined = true;
}
public ApiUpdateMmsMtMessageDto callbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
this.callbackUrlDefined = true;
return this;
}
/**
* Override the default callback URL for this batch. Constraints: Must be valid URL.
*
* @return callbackUrl
*/
@JsonProperty(JSON_PROPERTY_CALLBACK_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCallbackUrl() {
return callbackUrl;
}
@JsonIgnore
public boolean getCallbackUrlDefined() {
return callbackUrlDefined;
}
@JsonProperty(JSON_PROPERTY_CALLBACK_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCallbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
this.callbackUrlDefined = true;
}
public ApiUpdateMmsMtMessageDto body(MediaBodyDto body) {
this.body = body;
this.bodyDefined = true;
return this;
}
/**
* Get body
*
* @return body
*/
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public MediaBodyDto getBody() {
return body;
}
@JsonIgnore
public boolean getBodyDefined() {
return bodyDefined;
}
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setBody(MediaBodyDto body) {
this.body = body;
this.bodyDefined = true;
}
public ApiUpdateMmsMtMessageDto parameters(ParameterObjDto parameters) {
this.parameters = parameters;
this.parametersDefined = true;
return this;
}
/**
* Get parameters
*
* @return parameters
*/
@JsonProperty(JSON_PROPERTY_PARAMETERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public ParameterObjDto getParameters() {
return parameters;
}
@JsonIgnore
public boolean getParametersDefined() {
return parametersDefined;
}
@JsonProperty(JSON_PROPERTY_PARAMETERS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setParameters(ParameterObjDto parameters) {
this.parameters = parameters;
this.parametersDefined = true;
}
public ApiUpdateMmsMtMessageDto strictValidation(Boolean strictValidation) {
this.strictValidation = strictValidation;
this.strictValidationDefined = true;
return this;
}
/**
* Whether or not you want the media included in your message to be checked against [Sinch MMS
* channel best practices](/docs/mms/bestpractices/). If set to true, your message will be
* rejected if it doesn't conform to the listed recommendations, otherwise no validation will
* be performed.
*
* @return strictValidation
*/
@JsonProperty(JSON_PROPERTY_STRICT_VALIDATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getStrictValidation() {
return strictValidation;
}
@JsonIgnore
public boolean getStrictValidationDefined() {
return strictValidationDefined;
}
@JsonProperty(JSON_PROPERTY_STRICT_VALIDATION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStrictValidation(Boolean strictValidation) {
this.strictValidation = strictValidation;
this.strictValidationDefined = true;
}
/** Return true if this ApiUpdateMmsMtMessage object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ApiUpdateMmsMtMessageDto apiUpdateMmsMtMessage = (ApiUpdateMmsMtMessageDto) o;
return Objects.equals(this.from, apiUpdateMmsMtMessage.from)
&& Objects.equals(this.type, apiUpdateMmsMtMessage.type)
&& Objects.equals(this.toAdd, apiUpdateMmsMtMessage.toAdd)
&& Objects.equals(this.toRemove, apiUpdateMmsMtMessage.toRemove)
&& Objects.equals(this.deliveryReport, apiUpdateMmsMtMessage.deliveryReport)
&& Objects.equals(this.sendAt, apiUpdateMmsMtMessage.sendAt)
&& Objects.equals(this.expireAt, apiUpdateMmsMtMessage.expireAt)
&& Objects.equals(this.callbackUrl, apiUpdateMmsMtMessage.callbackUrl)
&& Objects.equals(this.body, apiUpdateMmsMtMessage.body)
&& Objects.equals(this.parameters, apiUpdateMmsMtMessage.parameters)
&& Objects.equals(this.strictValidation, apiUpdateMmsMtMessage.strictValidation);
}
@Override
public int hashCode() {
return Objects.hash(
from,
type,
toAdd,
toRemove,
deliveryReport,
sendAt,
expireAt,
callbackUrl,
body,
parameters,
strictValidation);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApiUpdateMmsMtMessageDto {\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" toAdd: ").append(toIndentedString(toAdd)).append("\n");
sb.append(" toRemove: ").append(toIndentedString(toRemove)).append("\n");
sb.append(" deliveryReport: ").append(toIndentedString(deliveryReport)).append("\n");
sb.append(" sendAt: ").append(toIndentedString(sendAt)).append("\n");
sb.append(" expireAt: ").append(toIndentedString(expireAt)).append("\n");
sb.append(" callbackUrl: ").append(toIndentedString(callbackUrl)).append("\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" strictValidation: ").append(toIndentedString(strictValidation)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy