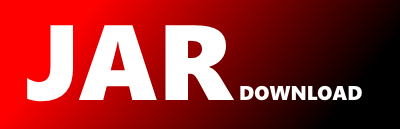
com.sinch.sdk.domains.sms.models.dto.v1.BinaryRequestDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** BinaryRequestDto */
@JsonPropertyOrder({
BinaryRequestDto.JSON_PROPERTY_TO,
BinaryRequestDto.JSON_PROPERTY_BODY,
BinaryRequestDto.JSON_PROPERTY_UDH,
BinaryRequestDto.JSON_PROPERTY_FROM,
BinaryRequestDto.JSON_PROPERTY_TYPE,
BinaryRequestDto.JSON_PROPERTY_DELIVERY_REPORT,
BinaryRequestDto.JSON_PROPERTY_SEND_AT,
BinaryRequestDto.JSON_PROPERTY_EXPIRE_AT,
BinaryRequestDto.JSON_PROPERTY_CALLBACK_URL,
BinaryRequestDto.JSON_PROPERTY_CLIENT_REFERENCE,
BinaryRequestDto.JSON_PROPERTY_FEEDBACK_ENABLED,
BinaryRequestDto.JSON_PROPERTY_FLASH_MESSAGE,
BinaryRequestDto.JSON_PROPERTY_TRUNCATE_CONCAT,
BinaryRequestDto.JSON_PROPERTY_MAX_NUMBER_OF_MESSAGE_PARTS,
BinaryRequestDto.JSON_PROPERTY_FROM_TON,
BinaryRequestDto.JSON_PROPERTY_FROM_NPI
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class BinaryRequestDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_TO = "to";
private List to;
private boolean toDefined = false;
public static final String JSON_PROPERTY_BODY = "body";
private String body;
private boolean bodyDefined = false;
public static final String JSON_PROPERTY_UDH = "udh";
private String udh;
private boolean udhDefined = false;
public static final String JSON_PROPERTY_FROM = "from";
private String from;
private boolean fromDefined = false;
/**
* SMS in <a
* href=\"https://community.sinch.com/t5/Glossary/Binary-SMS/ta-p/7470\"
* target=\"_blank\">binary</a> format.
*/
public enum TypeEnum {
MT_BINARY("mt_binary"),
UNKNOWN_DEFAULT_OPEN_API("unknown_default_open_api");
private String value;
TypeEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static TypeEnum fromValue(String value) {
for (TypeEnum b : TypeEnum.values()) {
if (b.value.equals(value)) {
return b;
}
}
return UNKNOWN_DEFAULT_OPEN_API;
}
}
public static final String JSON_PROPERTY_TYPE = "type";
private String type;
private boolean typeDefined = false;
public static final String JSON_PROPERTY_DELIVERY_REPORT = "delivery_report";
private String deliveryReport;
private boolean deliveryReportDefined = false;
public static final String JSON_PROPERTY_SEND_AT = "send_at";
private OffsetDateTime sendAt;
private boolean sendAtDefined = false;
public static final String JSON_PROPERTY_EXPIRE_AT = "expire_at";
private OffsetDateTime expireAt;
private boolean expireAtDefined = false;
public static final String JSON_PROPERTY_CALLBACK_URL = "callback_url";
private String callbackUrl;
private boolean callbackUrlDefined = false;
public static final String JSON_PROPERTY_CLIENT_REFERENCE = "client_reference";
private String clientReference;
private boolean clientReferenceDefined = false;
public static final String JSON_PROPERTY_FEEDBACK_ENABLED = "feedback_enabled";
private Boolean feedbackEnabled;
private boolean feedbackEnabledDefined = false;
public static final String JSON_PROPERTY_FLASH_MESSAGE = "flash_message";
private Boolean flashMessage;
private boolean flashMessageDefined = false;
public static final String JSON_PROPERTY_TRUNCATE_CONCAT = "truncate_concat";
private Boolean truncateConcat;
private boolean truncateConcatDefined = false;
public static final String JSON_PROPERTY_MAX_NUMBER_OF_MESSAGE_PARTS =
"max_number_of_message_parts";
private Integer maxNumberOfMessageParts;
private boolean maxNumberOfMessagePartsDefined = false;
public static final String JSON_PROPERTY_FROM_TON = "from_ton";
private Integer fromTon;
private boolean fromTonDefined = false;
public static final String JSON_PROPERTY_FROM_NPI = "from_npi";
private Integer fromNpi;
private boolean fromNpiDefined = false;
public BinaryRequestDto() {}
public BinaryRequestDto to(List to) {
this.to = to;
this.toDefined = true;
return this;
}
public BinaryRequestDto addToItem(String toItem) {
if (this.to == null) {
this.to = new ArrayList<>();
}
this.toDefined = true;
this.to.add(toItem);
return this;
}
/**
* A list of phone numbers and group IDs that will receive the batch. [More
* info](https://community.sinch.com/t5/Glossary/MSISDN/ta-p/7628).
*
* @return to
*/
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public List getTo() {
return to;
}
@JsonIgnore
public boolean getToDefined() {
return toDefined;
}
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setTo(List to) {
this.to = to;
this.toDefined = true;
}
public BinaryRequestDto body(String body) {
this.body = body;
this.bodyDefined = true;
return this;
}
/**
* The message content Base64 encoded. Max 140 bytes including `udh`.
*
* @return body
*/
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getBody() {
return body;
}
@JsonIgnore
public boolean getBodyDefined() {
return bodyDefined;
}
@JsonProperty(JSON_PROPERTY_BODY)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setBody(String body) {
this.body = body;
this.bodyDefined = true;
}
public BinaryRequestDto udh(String udh) {
this.udh = udh;
this.udhDefined = true;
return this;
}
/**
* The UDH header of a binary message HEX encoded. Max 140 bytes including the `body`.
*
* @return udh
*/
@JsonProperty(JSON_PROPERTY_UDH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getUdh() {
return udh;
}
@JsonIgnore
public boolean getUdhDefined() {
return udhDefined;
}
@JsonProperty(JSON_PROPERTY_UDH)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setUdh(String udh) {
this.udh = udh;
this.udhDefined = true;
}
public BinaryRequestDto from(String from) {
this.from = from;
this.fromDefined = true;
return this;
}
/**
* Sender number. Must be valid phone number, short code or alphanumeric. Required if Automatic
* Default Originator not configured.
*
* @return from
*/
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getFrom() {
return from;
}
@JsonIgnore
public boolean getFromDefined() {
return fromDefined;
}
@JsonProperty(JSON_PROPERTY_FROM)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFrom(String from) {
this.from = from;
this.fromDefined = true;
}
public BinaryRequestDto type(String type) {
this.type = type;
this.typeDefined = true;
return this;
}
/**
* SMS in <a
* href=\"https://community.sinch.com/t5/Glossary/Binary-SMS/ta-p/7470\"
* target=\"_blank\">binary</a> format.
*
* @return type
*/
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getType() {
return type;
}
@JsonIgnore
public boolean getTypeDefined() {
return typeDefined;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setType(String type) {
this.type = type;
this.typeDefined = true;
}
public BinaryRequestDto deliveryReport(String deliveryReport) {
this.deliveryReport = deliveryReport;
this.deliveryReportDefined = true;
return this;
}
/**
* Request delivery report callback. Note that delivery reports can be fetched from the API
* regardless of this setting.
*
* @return deliveryReport
*/
@JsonProperty(JSON_PROPERTY_DELIVERY_REPORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDeliveryReport() {
return deliveryReport;
}
@JsonIgnore
public boolean getDeliveryReportDefined() {
return deliveryReportDefined;
}
@JsonProperty(JSON_PROPERTY_DELIVERY_REPORT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDeliveryReport(String deliveryReport) {
this.deliveryReport = deliveryReport;
this.deliveryReportDefined = true;
}
public BinaryRequestDto sendAt(OffsetDateTime sendAt) {
this.sendAt = sendAt;
this.sendAtDefined = true;
return this;
}
/**
* If set in the future the message will be delayed until `send_at` occurs. Must be
* before `expire_at`. If set in the past, messages will be sent immediately. Formatted
* as [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601). For example:
* `YYYY-MM-DDThh:mm:ss.SSSZ`.
*
* @return sendAt
*/
@JsonProperty(JSON_PROPERTY_SEND_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getSendAt() {
return sendAt;
}
@JsonIgnore
public boolean getSendAtDefined() {
return sendAtDefined;
}
@JsonProperty(JSON_PROPERTY_SEND_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSendAt(OffsetDateTime sendAt) {
this.sendAt = sendAt;
this.sendAtDefined = true;
}
public BinaryRequestDto expireAt(OffsetDateTime expireAt) {
this.expireAt = expireAt;
this.expireAtDefined = true;
return this;
}
/**
* If set, the system will stop trying to deliver the message at this point. Must be after
* `send_at`. Default and max is 3 days after `send_at`. Formatted as
* [ISO-8601](https://en.wikipedia.org/wiki/ISO_8601). For example:
* `YYYY-MM-DDThh:mm:ss.SSSZ`.
*
* @return expireAt
*/
@JsonProperty(JSON_PROPERTY_EXPIRE_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getExpireAt() {
return expireAt;
}
@JsonIgnore
public boolean getExpireAtDefined() {
return expireAtDefined;
}
@JsonProperty(JSON_PROPERTY_EXPIRE_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setExpireAt(OffsetDateTime expireAt) {
this.expireAt = expireAt;
this.expireAtDefined = true;
}
public BinaryRequestDto callbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
this.callbackUrlDefined = true;
return this;
}
/**
* Override the *default* callback URL for this batch. Must be a valid URL. Learn how to set a
* default callback URL <a
* href=\"https://community.sinch.com/t5/SMS/How-do-I-assign-a-callback-URL-to-an-SMS-service-plan/ta-p/8414\"
* target=\"_blank\">here</a>.
*
* @return callbackUrl
*/
@JsonProperty(JSON_PROPERTY_CALLBACK_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getCallbackUrl() {
return callbackUrl;
}
@JsonIgnore
public boolean getCallbackUrlDefined() {
return callbackUrlDefined;
}
@JsonProperty(JSON_PROPERTY_CALLBACK_URL)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCallbackUrl(String callbackUrl) {
this.callbackUrl = callbackUrl;
this.callbackUrlDefined = true;
}
public BinaryRequestDto clientReference(String clientReference) {
this.clientReference = clientReference;
this.clientReferenceDefined = true;
return this;
}
/**
* The client identifier of a batch message. If set, the identifier will be added in the delivery
* report/callback of this batch.
*
* @return clientReference
*/
@JsonProperty(JSON_PROPERTY_CLIENT_REFERENCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getClientReference() {
return clientReference;
}
@JsonIgnore
public boolean getClientReferenceDefined() {
return clientReferenceDefined;
}
@JsonProperty(JSON_PROPERTY_CLIENT_REFERENCE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setClientReference(String clientReference) {
this.clientReference = clientReference;
this.clientReferenceDefined = true;
}
public BinaryRequestDto feedbackEnabled(Boolean feedbackEnabled) {
this.feedbackEnabled = feedbackEnabled;
this.feedbackEnabledDefined = true;
return this;
}
/**
* If set to true then
* [feedback](/docs/sms/api-reference/sms/tag/Batches/#tag/Batches/operation/deliveryFeedback) is
* expected after successful delivery.
*
* @return feedbackEnabled
*/
@JsonProperty(JSON_PROPERTY_FEEDBACK_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getFeedbackEnabled() {
return feedbackEnabled;
}
@JsonIgnore
public boolean getFeedbackEnabledDefined() {
return feedbackEnabledDefined;
}
@JsonProperty(JSON_PROPERTY_FEEDBACK_ENABLED)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFeedbackEnabled(Boolean feedbackEnabled) {
this.feedbackEnabled = feedbackEnabled;
this.feedbackEnabledDefined = true;
}
public BinaryRequestDto flashMessage(Boolean flashMessage) {
this.flashMessage = flashMessage;
this.flashMessageDefined = true;
return this;
}
/**
* Shows message on screen without user interaction while not saving the message to the inbox.
*
* @return flashMessage
*/
@JsonProperty(JSON_PROPERTY_FLASH_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getFlashMessage() {
return flashMessage;
}
@JsonIgnore
public boolean getFlashMessageDefined() {
return flashMessageDefined;
}
@JsonProperty(JSON_PROPERTY_FLASH_MESSAGE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFlashMessage(Boolean flashMessage) {
this.flashMessage = flashMessage;
this.flashMessageDefined = true;
}
public BinaryRequestDto truncateConcat(Boolean truncateConcat) {
this.truncateConcat = truncateConcat;
this.truncateConcatDefined = true;
return this;
}
/**
* If set to `true`, the message will be shortened when exceeding one part.
*
* @return truncateConcat
*/
@JsonProperty(JSON_PROPERTY_TRUNCATE_CONCAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getTruncateConcat() {
return truncateConcat;
}
@JsonIgnore
public boolean getTruncateConcatDefined() {
return truncateConcatDefined;
}
@JsonProperty(JSON_PROPERTY_TRUNCATE_CONCAT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTruncateConcat(Boolean truncateConcat) {
this.truncateConcat = truncateConcat;
this.truncateConcatDefined = true;
}
public BinaryRequestDto maxNumberOfMessageParts(Integer maxNumberOfMessageParts) {
this.maxNumberOfMessageParts = maxNumberOfMessageParts;
this.maxNumberOfMessagePartsDefined = true;
return this;
}
/**
* Message will be dispatched only if it is not split to more parts than the maximum number of
* message parts. minimum: 1
*
* @return maxNumberOfMessageParts
*/
@JsonProperty(JSON_PROPERTY_MAX_NUMBER_OF_MESSAGE_PARTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getMaxNumberOfMessageParts() {
return maxNumberOfMessageParts;
}
@JsonIgnore
public boolean getMaxNumberOfMessagePartsDefined() {
return maxNumberOfMessagePartsDefined;
}
@JsonProperty(JSON_PROPERTY_MAX_NUMBER_OF_MESSAGE_PARTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMaxNumberOfMessageParts(Integer maxNumberOfMessageParts) {
this.maxNumberOfMessageParts = maxNumberOfMessageParts;
this.maxNumberOfMessagePartsDefined = true;
}
public BinaryRequestDto fromTon(Integer fromTon) {
this.fromTon = fromTon;
this.fromTonDefined = true;
return this;
}
/**
* The type of number for the sender number. Use to override the automatic detection. minimum: 0
* maximum: 6
*
* @return fromTon
*/
@JsonProperty(JSON_PROPERTY_FROM_TON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getFromTon() {
return fromTon;
}
@JsonIgnore
public boolean getFromTonDefined() {
return fromTonDefined;
}
@JsonProperty(JSON_PROPERTY_FROM_TON)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFromTon(Integer fromTon) {
this.fromTon = fromTon;
this.fromTonDefined = true;
}
public BinaryRequestDto fromNpi(Integer fromNpi) {
this.fromNpi = fromNpi;
this.fromNpiDefined = true;
return this;
}
/**
* Number Plan Indicator for the sender number. Use to override the automatic detection. minimum:
* 0 maximum: 18
*
* @return fromNpi
*/
@JsonProperty(JSON_PROPERTY_FROM_NPI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getFromNpi() {
return fromNpi;
}
@JsonIgnore
public boolean getFromNpiDefined() {
return fromNpiDefined;
}
@JsonProperty(JSON_PROPERTY_FROM_NPI)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setFromNpi(Integer fromNpi) {
this.fromNpi = fromNpi;
this.fromNpiDefined = true;
}
/** Return true if this BinaryRequest object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
BinaryRequestDto binaryRequest = (BinaryRequestDto) o;
return Objects.equals(this.to, binaryRequest.to)
&& Objects.equals(this.body, binaryRequest.body)
&& Objects.equals(this.udh, binaryRequest.udh)
&& Objects.equals(this.from, binaryRequest.from)
&& Objects.equals(this.type, binaryRequest.type)
&& Objects.equals(this.deliveryReport, binaryRequest.deliveryReport)
&& Objects.equals(this.sendAt, binaryRequest.sendAt)
&& Objects.equals(this.expireAt, binaryRequest.expireAt)
&& Objects.equals(this.callbackUrl, binaryRequest.callbackUrl)
&& Objects.equals(this.clientReference, binaryRequest.clientReference)
&& Objects.equals(this.feedbackEnabled, binaryRequest.feedbackEnabled)
&& Objects.equals(this.flashMessage, binaryRequest.flashMessage)
&& Objects.equals(this.truncateConcat, binaryRequest.truncateConcat)
&& Objects.equals(this.maxNumberOfMessageParts, binaryRequest.maxNumberOfMessageParts)
&& Objects.equals(this.fromTon, binaryRequest.fromTon)
&& Objects.equals(this.fromNpi, binaryRequest.fromNpi);
}
@Override
public int hashCode() {
return Objects.hash(
to,
body,
udh,
from,
type,
deliveryReport,
sendAt,
expireAt,
callbackUrl,
clientReference,
feedbackEnabled,
flashMessage,
truncateConcat,
maxNumberOfMessageParts,
fromTon,
fromNpi);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class BinaryRequestDto {\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append(" body: ").append(toIndentedString(body)).append("\n");
sb.append(" udh: ").append(toIndentedString(udh)).append("\n");
sb.append(" from: ").append(toIndentedString(from)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" deliveryReport: ").append(toIndentedString(deliveryReport)).append("\n");
sb.append(" sendAt: ").append(toIndentedString(sendAt)).append("\n");
sb.append(" expireAt: ").append(toIndentedString(expireAt)).append("\n");
sb.append(" callbackUrl: ").append(toIndentedString(callbackUrl)).append("\n");
sb.append(" clientReference: ").append(toIndentedString(clientReference)).append("\n");
sb.append(" feedbackEnabled: ").append(toIndentedString(feedbackEnabled)).append("\n");
sb.append(" flashMessage: ").append(toIndentedString(flashMessage)).append("\n");
sb.append(" truncateConcat: ").append(toIndentedString(truncateConcat)).append("\n");
sb.append(" maxNumberOfMessageParts: ")
.append(toIndentedString(maxNumberOfMessageParts))
.append("\n");
sb.append(" fromTon: ").append(toIndentedString(fromTon)).append("\n");
sb.append(" fromNpi: ").append(toIndentedString(fromNpi)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy