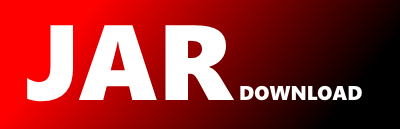
com.sinch.sdk.domains.sms.models.dto.v1.CreateGroupResponseDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import java.time.OffsetDateTime;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.Set;
/** CreateGroupResponseDto */
@JsonPropertyOrder({
CreateGroupResponseDto.JSON_PROPERTY_ID,
CreateGroupResponseDto.JSON_PROPERTY_NAME,
CreateGroupResponseDto.JSON_PROPERTY_SIZE,
CreateGroupResponseDto.JSON_PROPERTY_CREATED_AT,
CreateGroupResponseDto.JSON_PROPERTY_MODIFIED_AT,
CreateGroupResponseDto.JSON_PROPERTY_CHILD_GROUPS,
CreateGroupResponseDto.JSON_PROPERTY_AUTO_UPDATE
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class CreateGroupResponseDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_ID = "id";
private String id;
private boolean idDefined = false;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
private boolean nameDefined = false;
public static final String JSON_PROPERTY_SIZE = "size";
private Integer size;
private boolean sizeDefined = false;
public static final String JSON_PROPERTY_CREATED_AT = "created_at";
private OffsetDateTime createdAt;
private boolean createdAtDefined = false;
public static final String JSON_PROPERTY_MODIFIED_AT = "modified_at";
private OffsetDateTime modifiedAt;
private boolean modifiedAtDefined = false;
public static final String JSON_PROPERTY_CHILD_GROUPS = "child_groups";
private Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy