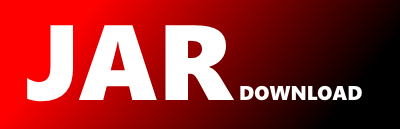
com.sinch.sdk.domains.sms.models.dto.v1.DryRun200ResponseDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
/** DryRun200ResponseDto */
@JsonPropertyOrder({
DryRun200ResponseDto.JSON_PROPERTY_NUMBER_OF_RECIPIENTS,
DryRun200ResponseDto.JSON_PROPERTY_NUMBER_OF_MESSAGES,
DryRun200ResponseDto.JSON_PROPERTY_PER_RECIPIENT
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class DryRun200ResponseDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_NUMBER_OF_RECIPIENTS = "number_of_recipients";
private Integer numberOfRecipients;
private boolean numberOfRecipientsDefined = false;
public static final String JSON_PROPERTY_NUMBER_OF_MESSAGES = "number_of_messages";
private Integer numberOfMessages;
private boolean numberOfMessagesDefined = false;
public static final String JSON_PROPERTY_PER_RECIPIENT = "per_recipient";
private List perRecipient;
private boolean perRecipientDefined = false;
public DryRun200ResponseDto() {}
public DryRun200ResponseDto numberOfRecipients(Integer numberOfRecipients) {
this.numberOfRecipients = numberOfRecipients;
this.numberOfRecipientsDefined = true;
return this;
}
/**
* The number of recipients in the batch
*
* @return numberOfRecipients
*/
@JsonProperty(JSON_PROPERTY_NUMBER_OF_RECIPIENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getNumberOfRecipients() {
return numberOfRecipients;
}
@JsonIgnore
public boolean getNumberOfRecipientsDefined() {
return numberOfRecipientsDefined;
}
@JsonProperty(JSON_PROPERTY_NUMBER_OF_RECIPIENTS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNumberOfRecipients(Integer numberOfRecipients) {
this.numberOfRecipients = numberOfRecipients;
this.numberOfRecipientsDefined = true;
}
public DryRun200ResponseDto numberOfMessages(Integer numberOfMessages) {
this.numberOfMessages = numberOfMessages;
this.numberOfMessagesDefined = true;
return this;
}
/**
* The total number of SMS message parts to be sent in the batch
*
* @return numberOfMessages
*/
@JsonProperty(JSON_PROPERTY_NUMBER_OF_MESSAGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getNumberOfMessages() {
return numberOfMessages;
}
@JsonIgnore
public boolean getNumberOfMessagesDefined() {
return numberOfMessagesDefined;
}
@JsonProperty(JSON_PROPERTY_NUMBER_OF_MESSAGES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setNumberOfMessages(Integer numberOfMessages) {
this.numberOfMessages = numberOfMessages;
this.numberOfMessagesDefined = true;
}
public DryRun200ResponseDto perRecipient(
List perRecipient) {
this.perRecipient = perRecipient;
this.perRecipientDefined = true;
return this;
}
public DryRun200ResponseDto addPerRecipientItem(
DryRun200ResponsePerRecipientInnerDto perRecipientItem) {
if (this.perRecipient == null) {
this.perRecipient = new ArrayList<>();
}
this.perRecipientDefined = true;
this.perRecipient.add(perRecipientItem);
return this;
}
/**
* The recipient, the number of message parts to this recipient, the body of the message, and the
* encoding type of each message
*
* @return perRecipient
*/
@JsonProperty(JSON_PROPERTY_PER_RECIPIENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getPerRecipient() {
return perRecipient;
}
@JsonIgnore
public boolean getPerRecipientDefined() {
return perRecipientDefined;
}
@JsonProperty(JSON_PROPERTY_PER_RECIPIENT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setPerRecipient(List perRecipient) {
this.perRecipient = perRecipient;
this.perRecipientDefined = true;
}
/** Return true if this Dry_Run_200_response object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DryRun200ResponseDto dryRun200Response = (DryRun200ResponseDto) o;
return Objects.equals(this.numberOfRecipients, dryRun200Response.numberOfRecipients)
&& Objects.equals(this.numberOfMessages, dryRun200Response.numberOfMessages)
&& Objects.equals(this.perRecipient, dryRun200Response.perRecipient);
}
@Override
public int hashCode() {
return Objects.hash(numberOfRecipients, numberOfMessages, perRecipient);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DryRun200ResponseDto {\n");
sb.append(" numberOfRecipients: ").append(toIndentedString(numberOfRecipients)).append("\n");
sb.append(" numberOfMessages: ").append(toIndentedString(numberOfMessages)).append("\n");
sb.append(" perRecipient: ").append(toIndentedString(perRecipient)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy