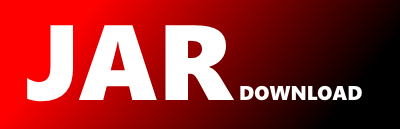
com.sinch.sdk.domains.sms.models.dto.v1.GroupObjectAutoUpdateDto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
/*
* API Overview | Sinch
* Sinch SMS API is one of the easiest APIs we offer and enables you to add fast and reliable global SMS to your applications. Send single messages, scheduled batch messages, use available message templates and more.
*
* The version of the OpenAPI document: v1
* Contact: [email protected]
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.sinch.sdk.domains.sms.models.dto.v1;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import java.util.Objects;
/** GroupObjectAutoUpdateDto */
@JsonPropertyOrder({
GroupObjectAutoUpdateDto.JSON_PROPERTY_TO,
GroupObjectAutoUpdateDto.JSON_PROPERTY_ADD,
GroupObjectAutoUpdateDto.JSON_PROPERTY_REMOVE
})
@JsonFilter("uninitializedFilter")
@JsonInclude(value = JsonInclude.Include.CUSTOM)
public class GroupObjectAutoUpdateDto {
private static final long serialVersionUID = 1L;
public static final String JSON_PROPERTY_TO = "to";
private String to;
private boolean toDefined = false;
public static final String JSON_PROPERTY_ADD = "add";
private UpdateGroupRequestAutoUpdateAddDto add;
private boolean addDefined = false;
public static final String JSON_PROPERTY_REMOVE = "remove";
private GroupObjectAutoUpdateRemoveDto remove;
private boolean removeDefined = false;
public GroupObjectAutoUpdateDto() {}
public GroupObjectAutoUpdateDto to(String to) {
this.to = to;
this.toDefined = true;
return this;
}
/**
* Short code or long number addressed in <a
* href=\"https://community.sinch.com/t5/Glossary/MO-Mobile-Originated/ta-p/7618\"
* target=\"_blank\">MO</a>. Constraints: Must be valid phone number or
* short code.
*
* @return to
*/
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getTo() {
return to;
}
@JsonIgnore
public boolean getToDefined() {
return toDefined;
}
@JsonProperty(JSON_PROPERTY_TO)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTo(String to) {
this.to = to;
this.toDefined = true;
}
public GroupObjectAutoUpdateDto add(UpdateGroupRequestAutoUpdateAddDto add) {
this.add = add;
this.addDefined = true;
return this;
}
/**
* Get add
*
* @return add
*/
@JsonProperty(JSON_PROPERTY_ADD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UpdateGroupRequestAutoUpdateAddDto getAdd() {
return add;
}
@JsonIgnore
public boolean getAddDefined() {
return addDefined;
}
@JsonProperty(JSON_PROPERTY_ADD)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setAdd(UpdateGroupRequestAutoUpdateAddDto add) {
this.add = add;
this.addDefined = true;
}
public GroupObjectAutoUpdateDto remove(GroupObjectAutoUpdateRemoveDto remove) {
this.remove = remove;
this.removeDefined = true;
return this;
}
/**
* Get remove
*
* @return remove
*/
@JsonProperty(JSON_PROPERTY_REMOVE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GroupObjectAutoUpdateRemoveDto getRemove() {
return remove;
}
@JsonIgnore
public boolean getRemoveDefined() {
return removeDefined;
}
@JsonProperty(JSON_PROPERTY_REMOVE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setRemove(GroupObjectAutoUpdateRemoveDto remove) {
this.remove = remove;
this.removeDefined = true;
}
/** Return true if this groupObject_auto_update object is equal to o. */
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
GroupObjectAutoUpdateDto groupObjectAutoUpdate = (GroupObjectAutoUpdateDto) o;
return Objects.equals(this.to, groupObjectAutoUpdate.to)
&& Objects.equals(this.add, groupObjectAutoUpdate.add)
&& Objects.equals(this.remove, groupObjectAutoUpdate.remove);
}
@Override
public int hashCode() {
return Objects.hash(to, add, remove);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class GroupObjectAutoUpdateDto {\n");
sb.append(" to: ").append(toIndentedString(to)).append("\n");
sb.append(" add: ").append(toIndentedString(add)).append("\n");
sb.append(" remove: ").append(toIndentedString(remove)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy