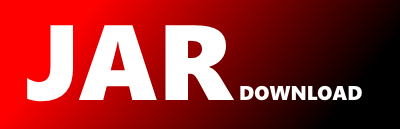
com.sinch.sdk.domains.sms.models.requests.GroupCreateRequestParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.sms.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import java.util.Collection;
/**
* Parameters request to create a group
*
* @see https://developers.sinch.com/docs/sms/api-reference/sms/tag/Groups/#tag/Groups/operation/CreateGroup
* @since 1.0
*/
public class GroupCreateRequestParameters {
private final OptionalValue name;
private final OptionalValue> members;
private final OptionalValue> childGroupIds;
private final OptionalValue autoUpdate;
private GroupCreateRequestParameters(
OptionalValue name,
OptionalValue> members,
OptionalValue> childGroupIds,
OptionalValue autoUpdate) {
this.name = name;
this.members = members;
this.childGroupIds = childGroupIds;
this.autoUpdate = autoUpdate;
}
public OptionalValue getName() {
return name;
}
public OptionalValue> getMembers() {
return members;
}
public OptionalValue> getChildGroupIds() {
return childGroupIds;
}
public OptionalValue getAutoUpdate() {
return autoUpdate;
}
@Override
public String toString() {
return "GroupCreateRequestParameters{"
+ "name='"
+ name
+ '\''
+ ", members="
+ members
+ ", childGroupIds="
+ childGroupIds
+ ", autoUpdate="
+ autoUpdate
+ '}';
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GroupCreateRequestParameters parameters) {
return new Builder(parameters);
}
public static class Builder {
OptionalValue name = OptionalValue.empty();
OptionalValue> members = OptionalValue.empty();
OptionalValue> childGroupIds = OptionalValue.empty();
OptionalValue autoUpdate = OptionalValue.empty();
private Builder() {}
private Builder(GroupCreateRequestParameters parameters) {
this.name = parameters.getName();
this.members = parameters.getMembers();
this.childGroupIds = parameters.getChildGroupIds();
this.autoUpdate = parameters.getAutoUpdate();
}
/**
* @param name Name of the group
* @return current builder
*/
public Builder setName(String name) {
this.name = OptionalValue.of(name);
return this;
}
/**
* @param members Initial list of phone numbers in E.164 format MSISDNs for the group
* @return current builder
*/
public Builder setMembers(Collection members) {
this.members = OptionalValue.of(members);
return this;
}
/**
* @param childGroupIds Child group IDs
* @return current builder
*/
public Builder setChildGroupIds(Collection childGroupIds) {
this.childGroupIds = OptionalValue.of(childGroupIds);
return this;
}
/**
* @param autoUpdate Auto update settings
* @return current builder
*/
public Builder setAutoUpdate(GroupAutoUpdateRequestParameters autoUpdate) {
this.autoUpdate = OptionalValue.of(autoUpdate);
return this;
}
public GroupCreateRequestParameters build() {
return new GroupCreateRequestParameters(name, members, childGroupIds, autoUpdate);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy