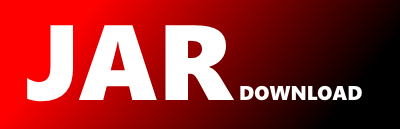
com.sinch.sdk.domains.verification.models.requests.StartVerificationRequestParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.verification.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.domains.verification.models.Identity;
import com.sinch.sdk.domains.verification.models.NumberIdentity;
import com.sinch.sdk.domains.verification.models.VerificationMethodType;
import com.sinch.sdk.domains.verification.models.VerificationReference;
/**
* Base class for start verification request parameters
*
* @since 1.0
*/
public abstract class StartVerificationRequestParameters {
private final VerificationMethodType method;
private final OptionalValue identity;
private final OptionalValue reference;
private final OptionalValue custom;
protected StartVerificationRequestParameters(
OptionalValue identity,
VerificationMethodType method,
OptionalValue reference,
OptionalValue custom) {
this.identity = identity;
this.method = method;
this.reference = reference;
this.custom = custom;
}
/**
* See {@link StartVerificationRequestParameters.Builder#setIdentity(NumberIdentity) builder
* setter}
*
* @return The reference value
* @since 1.0
*/
public OptionalValue getIdentity() {
return identity;
}
/**
* The type of the verification request.
*
* @return The method value
* @since 1.0
*/
public VerificationMethodType getMethod() {
return method;
}
/**
* See {@link StartVerificationRequestParameters.Builder#setReference(VerificationReference)
* builder setter}
*
* @return The reference value
* @since 1.0
*/
public OptionalValue getReference() {
return reference;
}
/**
* See {@link StartVerificationRequestParameters.Builder#setCustom(String) builder setter}
*
* @return The custom value
* @since 1.0
*/
public OptionalValue getCustom() {
return custom;
}
@Override
public String toString() {
return "StartVerificationRequestParameters{"
+ "method="
+ method
+ ", identity="
+ identity
+ ", reference='"
+ reference
+ '\''
+ ", custom='"
+ custom
+ '\''
+ '}';
}
/**
* Dedicated Builder
*
* @param Builder
* @since 1.0
*/
public abstract static class Builder> {
OptionalValue identity = OptionalValue.empty();
OptionalValue reference = OptionalValue.empty();
OptionalValue custom = OptionalValue.empty();
/**
* Specifies the type of endpoint that will be verified and the particular endpoint.
* NumberIdentity is currently the only supported endpoint type
*
* @param identity Specifies the type of endpoint that will be verified and the particular
* endpoint. number is currently the only supported endpoint type
* @return current builder
* @since 1.0
*/
public B setIdentity(NumberIdentity identity) {
this.identity = OptionalValue.of(identity);
return self();
}
/**
* Used to pass your own reference in the request for tracking purposes
*
* @param reference your own reference to be used
* @return current builder
* @since 1.0
*/
public B setReference(VerificationReference reference) {
this.reference = OptionalValue.of(reference);
return self();
}
/**
* Pass custom data in the request
*
* @param custom custom data
* @return current builder
* @since 1.0
*/
public B setCustom(String custom) {
this.custom = OptionalValue.of(custom);
return self();
}
/**
* Create instance
*
* @return The instance build with current builder values
* @since 1.0
*/
public abstract StartVerificationRequestParameters build();
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy