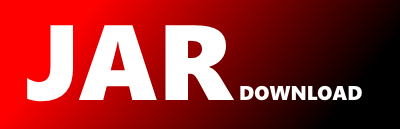
com.sinch.sdk.domains.verification.models.requests.VerificationReportRequestParameters Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sinch-sdk-java Show documentation
Show all versions of sinch-sdk-java Show documentation
SDK providing a Java API for the Sinch REST APIs.
package com.sinch.sdk.domains.verification.models.requests;
import com.sinch.sdk.core.models.OptionalValue;
import com.sinch.sdk.domains.verification.models.VerificationMethodType;
/**
* Base class for verification report request parameters
*
* @since 1.0
*/
public class VerificationReportRequestParameters {
private final OptionalValue method;
protected VerificationReportRequestParameters(OptionalValue method) {
this.method = method;
}
/**
* The type of the verification request.
*
* @return method value
* @since 1.0
*/
public OptionalValue getMethod() {
return method;
}
@Override
public String toString() {
return "VerificationReportRequestParameters{" + "method=" + method + '}';
}
protected static Builder> builder() {
return new Builder<>();
}
/**
* Dedicated Builder
*
* @param Builder
* @since 1.0
*/
public static class Builder> {
OptionalValue method;
protected Builder() {}
protected Builder(VerificationMethodType method) {
this.method = OptionalValue.of(method);
}
/**
* Create instance
*
* @return The instance build with current builder values
* @since 1.0
*/
public VerificationReportRequestParameters build() {
return new VerificationReportRequestParameters(method);
}
@SuppressWarnings("unchecked")
protected B self() {
return (B) this;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy